版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/m0_37972348/article/details/81020981
Android 使用 smartTable 表格工具 实现选中一行 并获得行所有信息
强力推荐一款 android 自动生成表格框架 smartTable,它有着非常强大的功能,而且日趋完善,能实现很多功能,而且使用也十分简单。
简单介绍它的功能:
- 快速配置自动生成表格;
- 自动计算表格宽高;
- 表格列标题组合;
- 表格固定左序列、顶部序列、第一行、列标题、统计行;
- 自动统计,排序(自定义统计规则);
- 表格图文、序列号、列标题格式化;
- 表格各组成背景、文字、网格、padding等配置;
- 表格批注;
- 表格内容、列标题点击事件;
- 缩放模式和滚动模式;
- 注解模式;
- 内容多行显示;
- 分页模式;
- 首尾动态添加数据;
- 丰富的格式化;
- 支持二维数组展示(用于类似日程表,电影选票等);
- 导入excel(支持颜色,字体,背景,批注,对齐,图片等基本Excel属性);
- 表格合并单元(支持注解合并,支持自动合并);
- 支持其他刷新框架SmartRefreshLayout;
- 可配置表格最小宽度(小于该宽度自动适配);
- 支持直接List或数组字段转列;
- 支持Json数据直接转换成表格;
- 支持表格网格指定行列显示;
- 支持自动生成表单。
具体使用方法不再赘述,可以去 GitHub 去查看:https://github.com/huangyanbin/smartTable
由于smartTable中没有实现选中行的功能,现在让我们来手动实现,效果图在文章最后,实现思路:
smartTable 生成的表格数据是按列逐个创建出来的,每一列可以建立一个监听事件,获得被点击的行索引,再根据行索引去其他列找到对应的记录,即可拼接出完整的行记录。
步骤一:引入 smartTable
添加 JitPack repository 到你的 Project build 文件
allprojects {
repositories {
...
maven { url 'https://www.jitpack.io' }
}
}
在 app build 文件中增加依赖
扫描二维码关注公众号,回复:
3747726 查看本文章
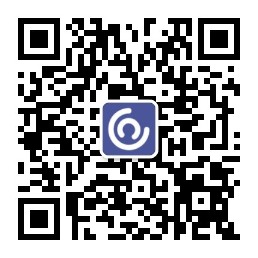
dependencies {
compile 'com.github.huangyanbin:SmartTable:2.2.0'
}
步骤二:定义一个简单的 User 对象,用于填充表格数据
public class User {
private String name;
private Integer age;
private Boolean operation; //用于记录是否被选中的状态
public User(String name, Integer age, boolean operation){
this.name = name;
this.age = age;
this.operation = operation;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Boolean getOperation() {
return operation;
}
public void setOperation(Boolean operation) {
this.operation = operation;
}
}
步骤三:按要求建立表格
public class MainActivity extends AppCompatActivity {
private SmartTable<User> table;
Column<String> name;
Column<Integer> age;
Column<Boolean> operation;
List<String> name_selected = new ArrayList<String>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
table = (SmartTable<User>)findViewById(R.id.table);
List<User> codeList = new ArrayList<User>();
codeList.add(new User("user_01",20,false));
codeList.add(new User("user_02",21,false));
codeList.add(new User("user_03",22,false));
codeList.add(new User("user_04",22,false));
codeList.add(new User("user_05",21,false));
codeList.add(new User("user_06",21,false));
codeList.add(new User("user_07",23,false));
codeList.add(new User("user_08",20,false));
name = new Column<>("姓名", "name");
name.setOnColumnItemClickListener(new OnColumnItemClickListener<String>() {
@Override
public void onClick(Column<String> column, String value, String bool, int position) {
Toast.makeText(MainActivity.this,"点击了"+value,Toast.LENGTH_SHORT).show();
if(operation.getDatas().get(position)){
showName(position, false);
operation.getDatas().set(position,false);
}else{
showName(position, true);
operation.getDatas().set(position,true);
}
table.refreshDrawableState(); //不要忘记刷新表格,否则选中效果会延时一步
table.invalidate();
}
});
age = new Column<>("年龄", "age");
age.setOnColumnItemClickListener(new OnColumnItemClickListener<Integer>() {
@Override
public void onClick(Column<Integer> column, String value, Integer bool, int position) {
// Toast.makeText(CodeListActivity.this,"点击了"+value,Toast.LENGTH_SHORT).show();
if(operation.getDatas().get(position)){
showName(position, false);
operation.getDatas().set(position,false);
}else{
showName(position, true);
operation.getDatas().set(position,true);
}
table.refreshDrawableState();
table.invalidate();
}
});
int size = DensityUtils.dp2px(this,30);
operation = new Column<>("勾选", "operation", new ImageResDrawFormat<Boolean>(size,size) { //设置"操作"这一列以图标显示 true、false 的状态
@Override
protected Context getContext() {
return MainActivity.this;
}
@Override
protected int getResourceID(Boolean isCheck, String value, int position) {
if(isCheck){
return R.mipmap.check; //将图标提前放入 app/res/mipmap 目录下
}
return R.mipmap.unselect_check;
}
});
operation.setComputeWidth(40);
operation.setOnColumnItemClickListener(new OnColumnItemClickListener<Boolean>() {
@Override
public void onClick(Column<Boolean> column, String value, Boolean bool, int position) {
// Toast.makeText(CodeListActivity.this,"点击了"+value,Toast.LENGTH_SHORT).show();
if(operation.getDatas().get(position)){
showName(position, false);
operation.getDatas().set(position,false);
}else{
showName(position, true);
operation.getDatas().set(position,true);
}
table.refreshDrawableState();
table.invalidate();
}
});
final TableData<User> tableData = new TableData<>("测试标题",codeList, operation, name, age);
table.getConfig().setShowTableTitle(false);
table.setTableData(tableData);
// table.getConfig().setContentStyle(new FontStyle(50, Color.BLUE));
table.getConfig().setMinTableWidth(1024); //设置表格最小宽度
FontStyle style = new FontStyle();
style.setTextSize(30);
table.getConfig().setContentStyle(style); //设置表格主题字体样式
table.getConfig().setColumnTitleStyle(style); //设置表格标题字体样式
table.getConfig().setContentCellBackgroundFormat(new BaseCellBackgroundFormat<CellInfo>() { //设置隔行变色
@Override
public int getBackGroundColor(CellInfo cellInfo) {
if(cellInfo.row%2 ==1) {
return ContextCompat.getColor(MainActivity.this, R.color.tableBackground); //需要在 app/res/values 中添加 <color name="tableBackground">#d4d4d4</color>
}else{
return TableConfig.INVALID_COLOR;
}
}
});
table.getConfig().setMinTableWidth(1024); //设置最小宽度
}
/**
* 收集所有被勾选的姓名记录到 name_selected 集合中,并实时更新
* @param position 被选择记录的行数,根据行数用来找到其他列中该行记录对应的信息
* @param selectedState 当前的操作状态:选中 || 取消选中
*/
public void showName(int position, boolean selectedState){
List<String> rotorIdList = name.getDatas();
if(position >-1){
String rotorTemp = rotorIdList.get(position);
if(selectedState && !name_selected.contains(rotorTemp)){ //当前操作是选中,并且“所有选中的姓名的集合”中没有该记录,则添加进去
name_selected.add(rotorTemp);
}else if(!selectedState && name_selected.contains(rotorTemp)){ // 当前操作是取消选中,并且“所有选中姓名的集合”总含有该记录,则删除该记录
name_selected.remove(rotorTemp);
}
}
for(String s:name_selected){
System.out.print(s + " -- ");
}
}
}