最近使用了spring data jpa来完成数据访问层的实现。感觉比较强大,也比较复杂,中间还有不少限制。个人还只学了一点皮毛。对于一般应用中各类简单的增删查改,spring data提供了根据名字直接查询的代理方法,啥都不需要做,唯一需要编写接口,命名方法,这部分实在是太方便了,而且简单查询解决了差不多80%的问题。
步骤:
1.配置pom.xml
a.配置Spring-data-jpa
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-jpa</artifactId>
<version>1.10.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
b.配置mysql连接
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.39</version>
</dependency>
2.配置application.properties
# 数据源配置
spring.datasource.url=jdbc:mysql://127.0.0.1:3306/test?useUnicode=true&characterEncoding=utf8
spring.datasource.username=test
spring.datasource.password=123123
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
#JPA (JpaBaseConfiguration, HibernateJpaAutoConfiguration)
spring.data.jpa.repositories.enabled=true
spring.jpa.database-platform=org.hibernate.dialect.MySQL5Dialect
spring.jpa.hibernate.naming-strategy=org.hibernate.cfg.DefaultNamingStrategy
spring.jpa.generate-ddl=false
spring.jpa.show-sql=true
spring.jpa.hibernate.naming_strategy=org.hibernate.cfg.DefaultNamingStrategy
spring.jpa.hibernate.naming.physical-strategy=org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl
3.实体类
@Entity
@Table(name = "bos_customer", schema = "dbo", catalog = "userdb")
public class BosCustomerModel {
private int id;
private String cname;
private String openId;
@Id
@Column(name = "id")
@GeneratedValue
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@Basic
@Column(name = "cname")
public String getCname() {
return cname;
}
public void setCname(String cname) {
this.cname = cname;
}
@Basic
@Column(name = "openId")
public String getOpenId() {
return openId;
}
public void setOpenId(String openId) {
this.openId = openId;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
BosCustomerModel that = (BosCustomerModel) o;
return id == that.id &&
Objects.equals(cname, that.cname) &&
Objects.equals(openId, that.openId);
}
@Override
public int hashCode() {
return Objects.hash(id, cname, openId);
}
}
4. CustomerJPARespository类
public interface CustomerJPARepository extends JpaRepository<BosCustomerModel, Integer>, JpaSpecificationExecutor<BosCustomerModel> {
@Query("select count(*) from BosCustomerModel where openId=?1")
int findBosCustomerModelByOpenId(String openId);
}
5.WeiService
public interface WeiService {
void insertCustomer(String openId,String cname);
BosCustomerModel findBosCustomerModelByOpenId(String openId);
}
6.WeiServiceImpl
@Service("weiServiceImpl")
public class WeiServiceImpl implements WeiService {
@Autowired
private CustomerJPARepository customerJPARepository;
@Transactional
@Override
public void insertCustomer(String openId,String cname){
BosCustomerModel model=new BosCustomerModel();
model.setCname(cname);
model.setOpenId(openId);
int row=customerJPARepository.findBosCustomerModelByOpenId(openId);
if(row==0){
customerJPARepository.saveAndFlush(model);
}
}
7.WeiController
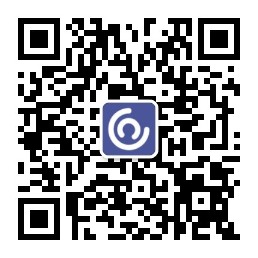
@RequestMapping(value="/insertData",method = RequestMethod.POST)
public String insertCustomer(@RequestParam("cname") String cname){
//第一次输入的时候将名字和openId保存到客户表中
weiService.insertCustomer(WeiXinController.OPENID,cname);
return "sucess";
}
8.补充一下springJPA的用法:
1.增加:
Bos_customerModel customer=new Bos_customerModel();
customer.setId(1);
customer.setCname("小王");
保存单个: customerJPARespository.save(customerModel);
保存或更新:customerJPARespository.saveAndFlush(customerModel);
保存多个: List<customerModel> customers=new ArrayList<customerModel>();
customers.add(customer);
customerJPARespository.save(customers);
2.删除
删除都是按照主键来删除的,区别是多条sql和单条sql
删除单条: customerJPARespository.delete(1);
删除全部(删除全部,先findALL查找出来,再一条一条删除,最后提交事务): customerJPARespository.deleteAll();
删除全部,一条sql
customerJPARespository.deleteAllInBatch();
删除集合,一条一条删除
List<customerModel> customers=new ArrayList<customerModel>();
CustomerModel customer=new CustomerModel();
customer.setId(1);
customer.setCname("gag);
customers.add(customer);
customerJPARespository.delete(customers);
删除集合,一条sql,拼接or
customerJPARespository.deleteInBatch(customers);
3.修改 (更新也是按照主键来更新)
CustomerModel customer=new CustomerModel();
customer.setId(1);
customer.setCname("gag);
customerJPARespository.saveAndFlush(customer);