Spring Data JPA虽然大大的简化了持久层的开发,但是在实际开发中,很多地方都需要高级动态查询,在实现动态查询时我们需要用到Criteria API,主要是以下三个:
1、Criteria 查询是以元模型的概念为基础的,元模型是为具体持久化单元的受管实体定义的,这些实体可以是实体类,嵌入类或者映射的父类。
2、CriteriaQuery接口:代表一个specific的顶层查询对象,它包含着查询的各个部分,比如:select 、from、where、group by、order by等注意:CriteriaQuery对象只对实体类型或嵌入式类型的Criteria查询起作用
3、Root接口:代表Criteria查询的根对象,Criteria查询的查询根定义了实体类型,能为将来导航获得想要的结果,它与SQL查询中的FROM子句类似
在JPA中,标准查询是以元模型的概念为基础的.元模型是为具体持久化单元的受管实体定义的.这些实体可以是实体类,嵌入类或者映射的父类.提供受管实体元信息的类就是元模型类。使用元模型类最大的优势是凭借其实例化可以在编译时访问实体的持久属性.该特性使得criteria 查询更加类型安全。例如:Product实体类对应的元模型Product_:
package powerx.io.bean; import javax.annotation.Generated; import javax.persistence.metamodel.SingularAttribute; import javax.persistence.metamodel.StaticMetamodel; @Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor") @StaticMetamodel(Product.class) public abstract class Product_ { public static volatile SingularAttribute<Product, Double> price; public static volatile SingularAttribute<Product, String> name; public static volatile SingularAttribute<Product, Integer> id; }
这样的元模型不用手动创建,有多种构建方式,本人使用的IDE是STS,具体操作如下:选中项目——鼠标右键Properties进入配置界面——java compiler下Annotation Processing——勾选enable project specific settings——Annotation Processing下Factory path——add external JARs ——加入事先下载好的hibernate-jpamodelgen-5.2.10.Final.jar——apply后则会在项目根目录下生成.apt_generated包,包内含有对应的实体类元模型(看不到的同学需要把resource过滤去掉),还有其它的方式,可参考http://docs.jboss.org/hibernate/jpamodelgen/1.0/reference/en-US/html/chapter-usage.html。
使用criteria 查询简单Demo
@Resource private EntityManager entityManager; public List<Product> findByConditions(String name, Double price) { //创建CriteriaBuilder安全查询工厂 //CriteriaBuilder是一个工厂对象,安全查询的开始.用于构建JPA安全查询. CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder(); //创建CriteriaQuery安全查询主语句 //CriteriaQuery对象必须在实体类型或嵌入式类型上的Criteria 查询上起作用。 CriteriaQuery<Product> query = criteriaBuilder.createQuery(Product.class); //Root 定义查询的From子句中能出现的类型 Root<Product> ProductRoot = query.from(Product.class); //Predicate 过滤条件 构建where字句可能的各种条件 //这里用List存放多种查询条件,实现动态查询 List<Predicate> predicatesList = new ArrayList<>(); //name模糊查询 ,like语句 if (name != null) { predicatesList.add( criteriaBuilder.and( criteriaBuilder.like( ProductRoot.get(Product_.name), "%" + name + "%"))); } // ProductPrice 小于等于 <= 语句 if (price != null) { predicatesList.add( criteriaBuilder.and( criteriaBuilder.le( ProductRoot.get(Product_.price), price))); } query.where(predicatesList.toArray(new Predicate[predicatesList.size()])); TypedQuery<Product> typedQuery = entityManager.createQuery(query); List<Product> resultList = typedQuery.getResultList(); return resultList; }
criteriaBuilder中各方法对应的语句:
equle : filed = value
gt / greaterThan : filed > value
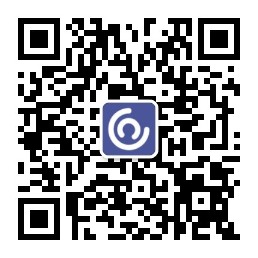
lt / lessThan : filed < value
ge / greaterThanOrEqualTo : filed >= value
le / lessThanOrEqualTo: filed <= value
notEqule : filed != value
like : filed like value
notLike : filed not like value
如果每个动态查询的地方都这么写,那就感觉太麻烦了。那实际上,在使用Spring Data JPA的时候,只要我们的Repo层接口继承JpaSpecificationExecutor接口就可以使用Specification进行动态查询,在这里因为findAll(Specification<T> var1, Pageable var2)
方法中参数 Specification<T>
是一个匿名内部类
那这里就可以直接用lambda表达式直接简化代码。
持久层接口类:
package powerx.io; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.data.jpa.repository.JpaSpecificationExecutor; import powerx.io.bean.Product; public interface ProductDao extends JpaRepository<Product, Integer>,JpaSpecificationExecutor<Product> { }
service层:
@Autowired private ProductDao productDao; public List<Product> findByConditions2(String name, Double price) { return productDao.findAll((root, criteriaQuery, criteriaBuilder) -> { List<Predicate> predicatesList = new ArrayList<>(); if (name != null) { predicatesList.add( criteriaBuilder.and( criteriaBuilder.like( root.get(Product_.name), "%" + name + "%"))); } // itemPrice 小于等于 <= 语句 if (price != null) { predicatesList.add( criteriaBuilder.and( criteriaBuilder.le( root.get(Product_.price), price))); } return criteriaBuilder.and( predicatesList.toArray(new Predicate[predicatesList.size()])); }); }
控制器类:
package powerx.io; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; @RestController public class ProductController { @Autowired private ProductServiceImpl productServiceImpl; @GetMapping("/find") public Object find(@RequestParam String name ,@RequestParam Double price) { return productServiceImpl.findByConditions2(name, price); } }
实体类:
package powerx.io.bean; import java.io.Serializable; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; @Entity public class Product implements Serializable{ private static final long serialVersionUID = 5800591455207338888L; @Id @GeneratedValue private Integer id; private String name; private Double price; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Double getPrice() { return price; } public void setPrice(Double price) { this.price = price; } }
使用springboot构建项目,pom.xml 和application.yml如下
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 连接mysql数据库驱动 --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <!-- 整合jpa --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
spring: datasource: url: jdbc:mysql://localhost:3306/springdatajpa username: root password: root jpa: show-sql: true hibernate: ddl-auto: update
启动项目,会根据实体类自动创建对应的表,加入测试数据,访问http://localhost:8080/find?name=apple&price=88,结果如下:
参考文章:https://www.jianshu.com/p/0939cec7e207