一,jackson
Java->Json
1 ObjectMap
2 JsonGenerator
3 @JsonIgnore
hibernate中一对多、多对多双向关联会出现这种死循环
二,HttpClient
1、简介:
HttpClient是Apache Jakarta Common下的子项目,用来提供高效的、最新的、
功能丰富的支持HTTP协议的客户端编程工具包,并且它支持HTTP协议最新的版本和协议
2、Android已成功集成了HttpClient,这意味这开发人员可以直接在Android应用中使用
HtppClient来提交请求、接收响应
注:在API 23中,Google已经移除了移除了Apache HttpClient相关的类 ,
在build.gradle中的android {}中加上useLibrary 'org.apache.http.legacy',
详情见资料“”
============================================================================================
案例一:HttpClient的使用步骤
1 创建HttpClient对象
HttpClient httpClient = new DefaultHttpClient();
2 创建HttpGet(或HttpPost)对象
HttpGet HttpGet = new HttpGet("http://www.baidu.com");
HttpPost httpPost = new HttpPost("http://www.baidu.com");
3 添加参数(可选)
setParams(HttpParams params)//HttpGet和HttpPost共有
setEntity(HttpEntity entity)//HttpPost独有
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("age", "20"));
params.add(new BasicNameValuePair("like", "aa"));
params.add(new BasicNameValuePair("like", "bb"));
params.add(new BasicNameValuePair("like", "cc"));
params.add(new BasicNameValuePair("newsCategoryId", "1"));
HttpEntity paramEntity = new UrlEncodedFormEntity(params,"UTF-8");
httpPost.setEntity(paramEntity);
4 发送GET(或POST)请求,并获得响应
HttpResponse httpResponse = httpClient.execute(HttpUriRequest request);
注1:HttpUriRequest为HttpGet和HttpPost的父类
注2:需要添加允许网络访问权限,不然会报错“java.lang.SecurityException: Permission denied (missing INTERNET permission?)”
<uses-permission android:name="android.permission.INTERNET" />
注3:如果地址错误,或服务器未开户,HttpClient这SB会等待N久(>24小时)。
所以请记得设置超时时间,所以请记得设置超时时间,所以请记得设置超时时间
所以请记得设置超时时间,所以请记得设置超时时间,所以请记得设置超时时间
所以请记得设置超时时间,所以请记得设置超时时间,所以请记得设置超时时间
另外HttpClient版本不一样,代码也不一样。下面的4.0版本的写法
httpClient.getParams().setParameter(CoreConnectionPNames.CONNECTION_TIMEOUT, 2000);// 连接时间
httpClient.getParams().setParameter(CoreConnectionPNames.SO_TIMEOUT, 2000);// 数据传输时间
5 处理响应
(1) 响应状态码(200)
httpResponse.getStatusLine().getStatusCode()
(2) 响应头
getAllHeaders()/getHeaders(String name)
(3) 响应内容
HttpEntity httpEntity = httpResponse.getEntity();//此对象包含服务器的响应内容
String result = EntityUtils.toString(httpEntity);
案例二:使用HttpClientUtils与服务器通信
Android客户端与服务器通信
1 JSON数据交换
android系统内置了对象json的支持,另外json其实就以下几个对象的使用:
JSONObject、JSONArray、JSONStringer、JSONException
2 web service,底层使用xml交换数据
补充:
1、关于org.apache.http.conn.HttpHostConnectException: Connection to refused错误的解决办法
2、增加网络访问权限:
找到 AndroidManifest.xml 文件。在application标签后面加上
3、检查ip地址:
启动的android模拟器吧自己也当成127.0.0.1和localhost,如果使用了localhost或者127.0.0.1则会被拒绝访问,
把ip地址改成实际地址,如:192.168.1.5
============================================================================================
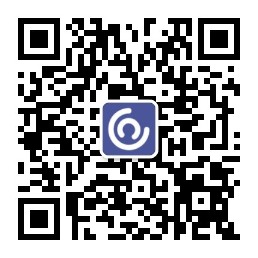
实例:
增删查改:
xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/bt_main_add"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="新增" />
<Button
android:id="@+id/bt_main_edit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="修改" />
<Button
android:id="@+id/bt_main_del"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="删除" />
<Button
android:id="@+id/bt_main_load"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="查单个" />
<Button
android:id="@+id/bt_main_list"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="查全部" />
</LinearLayout>
MainActivity.java
package com.example.t212_a08;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.Map;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Button bt_main_add;
private Button bt_main_edit;
private Button bt_main_del;
private Button bt_main_load;
private Button bt_main_list;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//查找控件
findViews();
//绑定监听器
bt_main_add.setOnClickListener(this);
bt_main_edit.setOnClickListener(this);
bt_main_del.setOnClickListener(this);
bt_main_load.setOnClickListener(this);
bt_main_list.setOnClickListener(this);
}
private void findViews() {
bt_main_add = (Button) findViewById(R.id.bt_main_add);
bt_main_edit = (Button) findViewById(R.id.bt_main_edit);
bt_main_del = (Button) findViewById(R.id.bt_main_del);
bt_main_load = (Button) findViewById(R.id.bt_main_load);
bt_main_list = (Button) findViewById(R.id.bt_main_list);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.bt_main_add:
add();
break;
case R.id.bt_main_edit:
edit();
break;
case R.id.bt_main_del:
del();
break;
case R.id.bt_main_load:
load();
break;
case R.id.bt_main_list:
list();
break;
}
}
private void add() {
Log.i("test", "add...");
//定义查询的方法
HttpClientPost post = new HttpClientPost("/jsp/userAction.action");
//定义要查询的条件
post.addParam("methodName","userAdd");
post.addParam("uid","007");
post.addParam("uname","lixiao");
post.addParam("roleid","001");
post.addParam("upwd","123456");
//点击回车键查询
post.submit(new HttpClientPost.Callback() {
@Override
public void execute(String json) {
Log.i("server22:" ,json);
}
});
}
//修改
private void edit() {
Log.i("test", "edit...");
//定义查询的方法
HttpClientPost post = new HttpClientPost("/jsp/userAction.action");
//定义要查询的条件
post.addParam("methodName","userUpdate");
post.addParam("SerialNo","1");
post.addParam("uid","001");
post.addParam("uname","张三3333");
post.addParam("roleid","001");
post.addParam("upwd","123456");
//点击回车键查询
post.submit(new HttpClientPost.Callback() {
@Override
public void execute(String json) {
Log.i("server22:" ,json);
}
});
}
//删除
private void del() {
Log.i("test", "del...");
//定义查询的方法
HttpClientPost post = new HttpClientPost("/jsp/userAction.action");
//定义要查询的条件
post.addParam("methodName","userDelete");
post.addParam("SerialNo","1");
//点击回车键查询
post.submit(new HttpClientPost.Callback() {
@Override
public void execute(String json) {
Log.i("server3:" ,json);
}
});
}
//查询单个
private void load() {
Log.i("test", "load...");
//定义查询的方法
HttpClientPost post = new HttpClientPost("/jsp/userAction.action");
//定义要查询的条件
post.addParam("methodName","userList");
post.addParam("uname","123123");
//点击回车键查询
post.submit(new HttpClientPost.Callback() {
@Override
public void execute(String json) {
ObjectMapper om = new ObjectMapper();
try {
Map map = om.readValue(json, Map.class);
Log.i("server:" ,map.get("total").toString());
//获取要查询的类型
Log.i("server:" ,map.get("rows").getClass().getName());
} catch (IOException e) {
e.printStackTrace();
}
}
});
}
//查询所有
private void list() {
Log.i("test", "list...");
//定义查询的方法
HttpClientPost post = new HttpClientPost("/jsp/userAction.action");
//定义要查询的条件
post.addParam("methodName","userList");
//点击回车键查询
post.submit(new HttpClientPost.Callback() {
@Override
public void execute(String json) {
Log.i("server11:" ,json);
}
});
}
}
HttpClientPost.java
package com.example.t212_a08;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.params.CoreConnectionPNames;
import org.apache.http.util.EntityUtils;
import android.os.AsyncTask;
/**
* 封装 HttpClient对象POST请求
*
*/
public class HttpClientPost implements Serializable {
private static final long serialVersionUID = 1777547416049652217L;
private static HttpClient httpClient = new DefaultHttpClient();
static {
httpClient.getParams().setParameter(
CoreConnectionPNames.CONNECTION_TIMEOUT, 2000);// 连接时间
httpClient.getParams().setParameter(CoreConnectionPNames.SO_TIMEOUT,
3000);// 数据传输时间
}
// 字符集
private static String encoding = "UTF-8";
// 服务器地址+端口号+项目名
private static String basePath = "http://192.168.43.158:8080/T212_easyui";
// 子控制器的路径
private String path;
// 保存请求中的参数
private List<NameValuePair> params = new ArrayList<NameValuePair>();;
public HttpClientPost(String path) {
super();
this.path = path;
}
/**
* 向POST请求中添加参数
*
* @param name
* @param value
*/
public void addParam(String name, String value) {
if (value == null) {
params.add(new BasicNameValuePair(name, ""));
} else {
params.add(new BasicNameValuePair(name, value));
}
}
/**
* 提交POST请求
*/
@SuppressWarnings("unchecked")
public void submit(final HttpClientPost.Callback callback) {
new AsyncTask() {
private String json;
@Override
protected Object doInBackground(Object... args) {
try {
// 1. 创建HttpClient对象
// 2. 创建HttpGet(或HttpPost)对象
HttpPost httpPost = new HttpPost(basePath + path);
// 3. 向POST请求中添加参数(可选)
if (0 != params.size()) {
HttpEntity paramEntity = new UrlEncodedFormEntity(
params, encoding);
httpPost.setEntity(paramEntity);
}
// 4. 发送POST请求,并获得响应
HttpResponse httpResponse = httpClient.execute(httpPost);
// 5. 处理响应
if (200 == httpResponse.getStatusLine().getStatusCode()) {
HttpEntity responseEntity = httpResponse.getEntity();// 此对象包含服务器的响应内容
this.json = EntityUtils.toString(responseEntity);
}
} catch (Exception e) {
throw new RuntimeException(e);
}
return null;
}
protected void onPostExecute(Object result) {
callback.execute(this.json);
}
}.execute();
}
public static interface Callback {
void execute(String json);
}
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.t212_a08">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>