一. 为什么SimpleDateFormat不是线程安全的?
Java源码如下:
/** * Date formats are not synchronized. * It is recommended to create separate format instances for each thread. * If multiple threads access a format concurrently, it must be synchronized * externally. */ public class SimpleDateFormat extends DateFormat { public Date parse(String text, ParsePosition pos){ calendar.clear(); // Clears all the time fields // other logic ... Date parsedDate = calendar.getTime(); } } abstract class DateFormat{ // other logic ... protected Calendar calendar; public Date parse(String source) throws ParseException{ ParsePosition pos = new ParsePosition(0); Date result = parse(source, pos); if (pos.index == 0) throw new ParseException("Unparseable date: \"" + source + "\"" , pos.errorIndex); return result; } }
如果我们把SimpleDateFormat定义成static成员变量,那么多个thread之间会共享这个sdf对象, 所以Calendar对象也会共享。
假定线程A和线程B都进入了parse(text, pos) 方法, 线程B执行到calendar.clear()后,线程A执行到calendar.getTime(), 那么就会有问题。
二. 问题重现:
package cn.com.common.thread; import java.text.SimpleDateFormat; import java.util.Locale; /** * * @ClassName: ThreadSimpledateformat * @Description:Simpledateformat线程安全 * @author linsky328 * @date 2017年7月4日 上午11:29:20 * */ public class ThreadSimpledateformat { private static SimpleDateFormat sdf = new SimpleDateFormat("dd-MMM-yyyy", Locale.US); private static String date[] = { "01-Jan-1999", "01-Jan-2000", "01-Jan-2001" }; public static void main(String[] args) { for (int i = 0; i < date.length; i++) { final int temp = i; new Thread(new Runnable() { @Override public void run() { try { while (true) { String str1 = date[temp]; String str2 = sdf.format(sdf.parse(str1)); System.out.println(Thread.currentThread().getName() + ", " + str1 + "," + str2); if(!str1.equals(str2)){ throw new RuntimeException(Thread.currentThread().getName() + ", Expected " + str1 + " but got " + str2); } } } catch (Exception e) { throw new RuntimeException("parse failed", e); } } }).start(); } } }
多次运行,便会出现异常错误:
Exception in thread "Thread-1" Thread-0, 01-Jan-1999,01-Jan-1999
Thread-0, 01-Jan-1999,01-Jan-1999
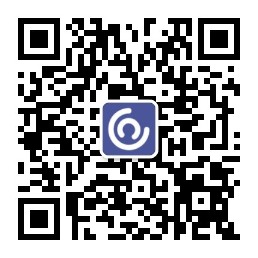
Thread-0, 01-Jan-1999,01-Jan-1999
Thread-0, 01-Jan-1999,01-Jan-1999
java.lang.RuntimeException: parse failed
at cn.com.common.thread.ThreadSimpledateformat$1.run(ThreadSimpledateformat.java:36)
at java.lang.Thread.run(Unknown Source)
Caused by: java.lang.NumberFormatException: For input string: ".11E1.11E1"
at sun.misc.FloatingDecimal.readJavaFormatString(Unknown Source)
线程访问的情况大致如下图:
SimpleDateFormat 类内部有一个 Calendar 对象引用,它用来储存和这个 SimpleDateFormat 相关的日期信息,例如sdf.parse(dateStr), sdf.format(date) 诸如此类的方法参数传入的日期相关String, Date等等, 都是交给 Calendar 引用来储存的.这样就会导致一个问题,如果你的 SimpleDateFormat 是个static 的, 那么多个thread 之间就会共享这个SimpleDateFormat , 同时也是共享这个Calendar引用,那么就出现时间混乱的情况。
三. 解决方案:
1. 解决方案a:
将SimpleDateFormat定义成局部变量:
private SimpleDateFormat sdf = new SimpleDateFormat("dd-MMM-yyyy", Locale.US);
缺点:每调用一次方法就会创建一个SimpleDateFormat对象,方法结束又要作为垃圾回收。,这样是非常耗费资源的。
2 解决方案b:
加一把线程同步锁:synchronized(lock)
package cn.com.common.thread; import java.text.SimpleDateFormat; import java.util.Locale; /** * * @ClassName: ThreadSimpledateformat * @Description:Simpledateformat线程安全 * @author linsky328 * @date 2017年7月4日 上午11:29:20 * */ public class ThreadSimpledateformat { private static SimpleDateFormat sdf = new SimpleDateFormat("dd-MMM-yyyy", Locale.US); private static String date[] = { "01-Jan-1999", "01-Jan-2000", "01-Jan-2001" }; public static void main(String[] args) { for (int i = 0; i < date.length; i++) { final int temp = i; new Thread(new Runnable() { @Override public void run() { try { while (true) { synchronized (sdf) { String str1 = date[temp]; String str2 = sdf.format(sdf.parse(str1)); System.out.println(Thread.currentThread().getName() + ", " + str1 + "," + str2); if (!str1.equals(str2)) { throw new RuntimeException(Thread.currentThread().getName() + ", Expected " + str1 + " but got " + str2); } } } } catch (Exception e) { throw new RuntimeException("parse failed", e); } } }).start(); } } }
缺点:性能较差,每次都要等待锁释放后其他线程才能进入。
3. 解决方案c: (推荐)
使用ThreadLocal: 每个线程都将拥有自己的SimpleDateFormat对象副本。
package cn.com.common.thread;
import java.text.SimpleDateFormat;
import java.util.Locale;
/**
*
* @ClassName: ThreadSimpledateformat
* @Description:Simpledateformat线程安全
* @author linsky328
* @date 2017年7月4日 上午11:29:20
*
*/
public class ThreadSimpledateformat {
private static String date[] = { "01-Jan-1999", "01-Jan-2000", "01-Jan-2001" };
private static ThreadLocal<SimpleDateFormat> local = new ThreadLocal<SimpleDateFormat>();
public static SimpleDateFormat getSimpleDateFormat(){
SimpleDateFormat sdf = local.get();
if (sdf == null) {
sdf = new SimpleDateFormat("dd-MMM-yyyy", Locale.US);
local.set(sdf);
}
return sdf;
}
public static void main(String[] args) {
for (int i = 0; i < date.length; i++) {
final int temp = i;
new Thread(new Runnable() {
@Override
public void run() {
try {
while (true) {
String str1 = date[temp];
String str2 = getSimpleDateFormat().format(getSimpleDateFormat().parse(str1));
System.out.println(Thread.currentThread().getName() + ", " + str1 + "," + str2);
if (!str1.equals(str2)) {
throw new RuntimeException(Thread.currentThread().getName() + ", Expected " + str1
+ " but got " + str2);
}
}
} catch (Exception e) {
throw new RuntimeException("parse failed", e);
}
}
}).start();
}
}
}