字母和数字之间转换
单个字母和字符之间的转换
字母和数字之间的转换利用ascii进行转换
a --> 97 int(a) 或者a+0
97--> a char(97) 1的ascii为49
1 --> '1' char('1'的ascii)
字母转数字, int(字母)
数字转字母 char(数字) 该数字 对应字母的ascii
#include <iostream> #include <cstdlib> #include <ctime> using namespace std; int main() { { cout << "a --> 97" << endl; char a = 'a'; int b = int(a); cout << b<<endl; } { //97 --> a cout << "97 --> a:"<<endl; int a = 97; char b = char(a); cout << b<<endl; } { cout << "1-->'1' "<<endl; // 1 --> '1' int a1 = 1; char b1 = 1+'0'; // // char b1 = int('1'); cout << b1<<endl; } { // '1' --> 1 cout << "'1' --> 1"<<endl; char a = '1'; int b = int(a-'0'); cout << b<<endl; } #if 0 字母和数字之间的转换利用ascii进行转换 a --> 97 int(a) 或者a+0 97--> a char(97) 1的ascii为49 1 --> '1' char('1'的ascii) 字母转数字, int(字母) 数字转字母 char(数字) 该数字 对应字母的ascii #endif return 0; }
字符串和数字之间的转化
方法一:
用stringstream 类
字符串转数字
1 #include <iostream> 2 #include <string> 3 #include <sstream> 4 #include <typeinfo> 5 using namespace std; 6 7 int main(){ 8 #if 0 9 double a = 123.32; 10 string res; 11 stringstream ss; 12 ss << a; // 将a的数据放入到ss 13 ss >> res;//或者 res = ss.str(); 将ss中的数据赋值给res 14 cout << typeid(ss).name() << endl; 15 #endif 16 string a = "123.32"; 17 double res; 18 stringstream temp; 19 temp << a; // 将a传入temp中 20 temp >> res; // 将转变后的temp输出到res中 21 cout << res+100; // 223.32; 22 return 0; 23 }
数字转字符串
1 #include <iostream> 2 #include <string> 3 #include <sstream> 4 #include <typeinfo> 5 #include <vector> 6 using namespace std; 7 8 int main(){ 9 10 double a = 123.32; 11 string res; 12 stringstream ss; 13 ss << a; // 将a的数据放入到ss 14 ss >> res;//或者 res = ss.str(); 将ss中的数据赋值给res 15 cout << res <<endl; 16 cout << typeid(ss).name() << endl; 17 18 19 return 0; 20 }
123.32
NSt7__cxx1118basic_stringstreamIcSt11char_traitsIcESaIcEEE
方法二数字转字符串
整型--->字符串
1 #include <iostream> 2 #include <string> 3 using namespace std; 4 5 int main(){ 6 7 char str[10]; 8 int old=1234321; 9 sprintf(str,"%d",old); // 将old中的数字转换成字符串存放在str中 10 cout << str; // 1234321 11 return 0; 12 }
浮点型
1 #include <iostream> 2 #include <string> 3 using namespace std; 4 5 int main(){ 6 7 char str[10]; 8 double a=123.321; 9 sprintf(str,"%.3lf",a); 10 cout << str; // 1234321 11 return 0; 12 }
方法二 字符转数字
用sscanf函数
1 #include <iostream> 2 #include <string> 3 using namespace std; 4 5 int main(){ 6 7 char str[]="1234321"; 8 int a; 9 sscanf(str,"%d",&a); // 将 str的字符转换成 数字 10 cout << a; // 1234321 11 return 0; 12 }
字符串和字符串之间的转换
1 (1)string --> char * 2 string str("OK"); 3 char * p = str.c_str(); 4 5 (2)char * -->string 6 char *p = "OK"; 7 string str(p); 8 9 (3)char * -->CString 10 char *p ="OK"; 11 CString m_Str(p); 12 //或者 13 CString m_Str; 14 m_Str.Format("%s",p); 15 16 (4)CString --> char * 17 CString str("OK"); 18 char * p = str.GetBuffer(0); 19 ... 20 str.ReleaseBuffer(); 21 22 (5)string --> CString 23 CString.Format("%s", string.c_str()); 24 25 (6)CString --> string 26 string s(CString.GetBuffer(0)); 27 GetBuffer()后一定要ReleaseBuffer(),否则就没有释放缓冲区所占的空间,CString对象不能动态增长了。 28 29 (7)double/float->CString 30 double data; 31 CString.Format("%.2f",data); //保留2位小数 32 33 (8)CString->double 34 CString s="123.12"; 35 double d=atof(s); 36 37 (9)string->double 38 double d=atof(s.c_str());
扫描二维码关注公众号,回复:
3581086 查看本文章
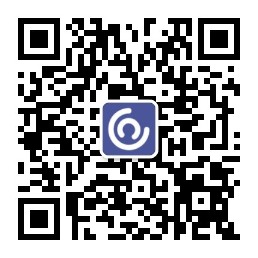