版权声明:共享知识,欢迎转载 https://blog.csdn.net/kakiebu/article/details/82983692
1. 结构指针
在C语言中传递结构变量总是传递地址
- const date* p表示通过p并不会修改它所指向的数据,但并不限制别人或者别的方法修改
- data const* p 表示通过p并不会修改它所指向的数据,但并不限制别人或者别的方法修改
#include <stdio.h>
typedef unsigned short int uint16;
typedef struct date{
uint16 year;
uint16 mouth;
uint16 day;
}date;
void print(date const* p){
//printf("%d年%d月%d日\n", (*p).year, (*p).mouth, (*p).day);
printf("%d年%d月%d日\n", p->year, p->mouth, p->day);//简化写法
}
int main(){
date a[3] = {{2018,5,1}, {2018,7,9}, {2018,10,18}};
int i;
for (i=0; i<3; i++){
print(&a[i]);
}
getchar();
return 0;
}
2. 通过指针访问堆区的空间(内存分配与释放)
堆 heap
要去访问请求堆区的内存空间,这样的内存成为动态内存,他对内存不是作用范围的限制,申请之后可以一直用,不论你在这个函数还是出这个函数,都一样用
void* callod(元素个数,每个元素的大小) 分配内存空间,大小是字节数,,返回他的开始地址,不成功返回null,分配后内存值置为0。
void* malloc(size_t bytes)分配内存空间,大小是字节数,,返回他的开始地址,不成功返回null,分配后内存值并不置为0。
void free(首地址) 释放内存,必须是堆空间的地址,或者说必须是 callod malloc realloc返回的地址,如果借了不还会造成内存泄漏,如果不释放会在程序结束后操作系统释放空间。
memcyp(输出内存地址,输入内存地址,复制内存大小 bytes)内存复制
memset(首地址,值,长度),内存清零
void* realloc(旧内存地址,新内存大小)把旧空间释放掉,申请一个新空间,可以保证旧空间数据可以复制到新空间中(要求新空间地址比旧空间大)。但是加长的那部分空间不保证清零。
#include <stdio.h>
#include <stdlib.h>
int main(){
double* p = (double*)malloc(sizeof(double));
int* a = (int*) calloc(5, sizeof(int));
int i;
//测试:虽然是同事申请的内存,但地址并不连续
printf("p=%p, a=%p\n", p, a);
*p = 1.23;
for (i=0; i<5; i++) {
a[i] = i+10; }
printf("%g\n", *p);
for (i=0; i<5; i++) {
printf("%d ", a[i]); }
printf("\n ");
free(p);
//测试 realloc 后数据都在
realloc(a, sizeof(int)*10);
for (i=0; i<10; i++) {
printf("%d ", a[i]); }
printf("\n ");
//a被释放了
a = (int*)realloc(a, 0);
printf("a=%p\n", a);
getchar();
return 0;
}
扫描二维码关注公众号,回复:
3571843 查看本文章
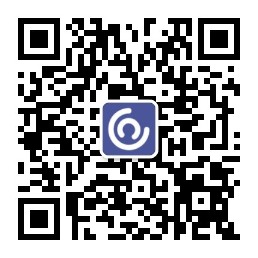