说明:
Python内置的数据类型有:列表(list)、字典(dictionary)、元组(tuple)、字符串(string)、集合(set)
本次重点:
Python操作列表、字典、元组
一、列表(list)
1.特性:list是一种有序的集合,可以随时添加和删除其中的元素。
2.创建:一对方括号“[]”和其包含的元素,单个元素可以不加逗号,同元组一样,可以创建嵌套列表。如:
tempList = ["one","two","three"]
#列表元素可以是不同数据类型的
L = ['Apple', 123, True]
3.基本操作及方法:
总结:
方法 | 描述 |
---|---|
list.append(x) | 把一个元素添加到列表的结尾,相当于 a[len(a):] = [x] |
详解:
(1)访问:
3.1.1 访问一般列表
用索引来访问list中每一个位置的元素,记得索引是从0开始的:
>>> tempList [0]
'one'
>>> tempList [1]
'two'
>>> tempList [2]
'three'
>>> tempList [3]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: list index out of range
当索引超出了范围时,Python会报一个IndexError错误,所以,要确保索引不要越界,记得最后一个元素的索引是len(tempList ) - 1。
如果要取最后一个元素,除了计算索引位置外,还可以用-1做索引,直接获取最后一个元素:
>>> tempList [-1]
'three'
以此类推,可以获取倒数第2个、倒数第3个:
>>> tempList [-2]
'two'
>>> tempList [-3]
'one'
>>> tempList [-4]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: list index out of range
当然,倒数第4个就越界了。
3.1.2 访问嵌套列表
如,列表如下:
>>> s = ['python', 'java', ['asp', 'php'], 'scheme']
>>> len(s)
4
访问:
s[2][1] -> 'php'
(2)遍历
可用for循环遍历列表
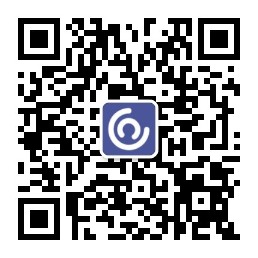
tempList = ["one","two","three"]
#用for循环遍历列表
for element in tempList:
print(element)
>>
one
two
three
(3)求长
len(列表名)可实现求长。
tempList = ["one","two","three"]
#长度
print(len(tempList))
>>
3
(4)打印
print(列表名)
tempList = ["one","two","three"]
#打印列表
print(tempList)
>>
['one', 'two', 'three']
(5)类型
type(列表名)
tempList = ["one","two","three"]
#查看类型
print(type(tempList))
>>
<class 'list'>
(6)追加
往list中追加元素到末尾:
tempList = ["one","two","three"]
#追加
tempList.append("four")
print(tempList)
>>
['one', 'two', 'three', 'four']
把元素插入到指定的位置,比如索引号为1的位置:
tempList = ["one","two","three"]
#把元素插入到指定的位置
tempList.insert(2,"four")
print(tempList)
>>
['one', 'two', 'four', 'three']
(7)替换
把某个元素替换成别的元素,可以直接赋值给对应的索引位置:
tempList = ["one","two","three"]
#替换元素
tempList[1] = "test"
print(tempList)
>>
['one', 'test', 'three']
(8)删除
删除list末尾的元素,用pop()方法:
tempList = ["one","two","three"]
#删除最后一个元素
tempList.pop()
"three"
print(tempList)
>>
['one', 'two']
要删除指定位置的元素,用pop(i)方法,其中i是索引位置:
tempList = ["one","two","three"]
#删除位置2的元素
tempList.pop(1)
"two"
print(tempList)
>>
['one', 'three']
4.高级操作及方法:
(1)产生一个数值递增列表:
-
pList = range(N),产生一个元素值为0~N-1的列表。如:pList = range(10) ->pList = [0,1,2,3,…,9]。
-
pList = range(sn,en),产生一个元素值为sn~en-1的列表。如:pList =
range(1,5) ->pList = [1,2,3,4]。 -
pList =range(sn,en,inc),产生一个元素值以inc递增的列表。如:pList = range(1,10,2) ->pList =
[1,3,5,7,9]
(2)固定值初始化:pList = [value for index in range(N)],产生一个长度为N的列表,元素值都为value.
如:value = “x”,N=10,则pLsit = [“x”,“x”,…,“x”]
更简单的形式:pList = [value]*N。
(3)操作符:
-
“+”:两个列表相加,将合成为一个列表。如 pL1 = [1,2],pL2 = [3,4],pL1+pL2 ->[1,2,3,4]
-
“*”:形式:[value]*N。如value=“a”,N=4,则得到列表[“a”,“a”,“a”,“a”]。
-
del:del pL[index]:删除指定位置的元素。 del pL[sIndex:eIndex]:删除sIndex~eIndex-1位置的元素。
(4)列表复制:
-
pL1 = pL:pL1为pL的别名,即pL1和pL实质为同一个列表,修改其中一个列表,另一个列表也随之变化。
如:pL = [1,2,3],pL1 = pL,pL1[2] = 33,->pL = pL1 = [1,2,33] -
pL2 = pL[:]:pL2为pL的一个克隆(或拷贝),即pL2和pL为不同的列表,修改其中一个列表,另一个不会受影响。
(5)常用方法:
-
L.append(value):向列表末尾插入一个元素
-
L.insert(index,value):向列表的index位置插入一个元素value。
-
L.pop(index): 从列表中删除指定位置index的元素并返回元素值,默认删除最后一个元素。
-
L.remove(value):删除在列表中第一次出现的元素value。如:pList = [1,2,3,2],pList.remove(2) ->pList = [1,3,2]。
-
L.count(value):返回元素value在列表中出现的次数。
-
L.index(value) :该元素第一次出现的的位置,无则抛异常 。
-
L.extend(list/tuple/string) :向列表末尾插入多个元素。
-
L.sort():排序
-
L.reverse():倒序
二、 字典(dictionary)
三、元组(tuple)
1.特性:元祖是一种有序列表。tuple和list非常类似,但是tuple一旦初始化就不能修改。【一旦创建元组,则这个元组就不能被修改,即不能对元组进行更新、增加、删除操作】
tuple的优势: 因为tuple不可变,所以代码更安全。如果可能,能用tuple代替list就尽量用tuple。
2.创建:一对圆括号“()”和其包含的元素(若没有元素,则为空元组),如:
tempTuple = ("one","two","three")