特征的匹配大致可以分为3个步骤:
- 特征的提取
- 计算特征向量
- 特征匹配
对于3个步骤,在OpenCV2中都进行了封装。所有的特征提取方法都实现FeatureDetector接口,DescriptorExtractor接口则封装了对特征向量(特征描述符)的提取,而所有特征向量的匹配都继承了DescriptorMatcher接口。
简单的特征匹配
int main()
{
const string imgName1 = "x://image//01.jpg";
const string imgName2 = "x://image//02.jpg";
Mat img1 = imread(imgName1);
Mat img2 = imread(imgName2);
if (!img1.data || !img2.data)
return -1;
//step1: Detect the keypoints using SURF Detector
int minHessian = 400;
SurfFeatureDetector detector(minHessian);
vector<KeyPoint> keypoints1, keypoints2;
detector.detect(img1, keypoints1);
detector.detect(img2, keypoints2);
//step2: Calculate descriptors (feature vectors)
SurfDescriptorExtractor extractor;
Mat descriptors1, descriptors2;
extractor.compute(img1, keypoints1, descriptors1);
extractor.compute(img2, keypoints2, descriptors2);
//step3:Matching descriptor vectors with a brute force matcher
BFMatcher matcher(NORM_L2);
vector<DMatch> matches;
matcher.match(descriptors1, descriptors2,matches);
//Draw matches
Mat imgMatches;
drawMatches(img1, keypoints1, img2, keypoints2, matches, imgMatches);
namedWindow("Matches");
imshow("Matches", imgMatches);
waitKey();
return 0;
}
- 实例化了一个特征提取器SurfFeatureDetector,其构造函数参数(minHessian)用来平衡提取到的特征点的数量和特征提取的稳定性的,对于不同的特征提取器改参数具有不同的含义和取值范围。
- 对得到的特征点提取特征向量(特征描述符)
- 匹配,上面代码使用了暴力匹配的方法,最后的匹配结果保存在vector<DMatch>中。
DMatch用来保存匹配后的结果
struct DMatch
{ //三个构造函数
DMatch() :
queryIdx(-1), trainIdx(-1), imgIdx(-1), distance(std::numeric_limits<float>::max()) {}
DMatch(int _queryIdx, int _trainIdx, float _distance) :
queryIdx(_queryIdx), trainIdx(_trainIdx), imgIdx(-1), distance(_distance) {}
DMatch(int _queryIdx, int _trainIdx, int _imgIdx, float _distance) : queryIdx(_queryIdx), trainIdx(_trainIdx), imgIdx(_imgIdx), distance(_distance) {}
int queryIdx; //此匹配对应的查询图像的特征描述子索引
int trainIdx; //此匹配对应的训练(模板)图像的特征描述子索引
int imgIdx; //训练图像的索引(若有多个)
float distance; //两个特征向量之间的欧氏距离,越小表明匹配度越高。
bool operator < (const DMatch &m) const;
};
扫描二维码关注公众号,回复:
3513041 查看本文章
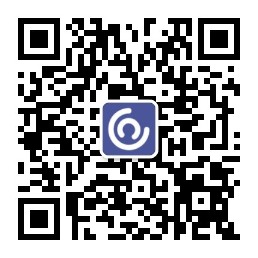
然后使用drawMatches方法可以匹配后的结构保存为Mat