为什么要构造双向链表呢?有什么用呢?《Java数据结构和算法》一书给出了一个例子回答了这两个问题:
“假设一个文本编辑器用链表来存储文本。屏幕上的每一行文字作为一个String对象存储在链结点中。当编辑器用户乡下移动光标时,程序移到下一个链结点操纵或显示新的一行。但是如果用户向上移动光标会发生什么呢?在普通的链表中,需要current变量(或起同等作用的其它变量)调回到表头,再一步一步地向后走到新的当前链结点,这样效率非常低。”
前面在说双端链表的时候,给出了从后面遍历结点一直到first即反向遍历的方法,效率低下,并不是我们很想要的,今天学习的双向链表就很好的解决了这个问题。双向链表即允许向前遍历,也允许向后遍历整个链表。诀窍就在于每个链结点都有两个指向其它链结点的引用,而不是一个。第一个像单链表一样指向下一个链结点。第二个指向前一个链结点。
其实双向链表实在单链表的基础上进行的,我们最主要的就是理解并且掌握一条线、两条线或者四条线的思想
一条线的情况和单链表是一样的,即first指向第一个结点,它前面没有其它的结点,这就是所谓的一条线
下图所示的箭头就是所谓的两条线、四条线:
两条线的情况:
两条线的情况:
理清了上述线的关系,双向链表的构造就会让你游刃有余了,其实在学习Java容器的时候,里面的LinkList就是双向链表来实现的,可以自行Google该容器,也可以看我博客中的另一篇文章—自定义LinkedList类,其实把这篇文章看懂了,LinkList想必也不成问题了。 自定义LinkedList博文地址:https://blog.csdn.net/szlg510027010/article/details/81905806
在双向链表中我们要比普通的链表即单链表要多一种东西即指向上一个结点的previous,即在定义Link类的时候我们需要多定义Link previous,完整地Link定义代码如下:(只是加了一条语句而已)
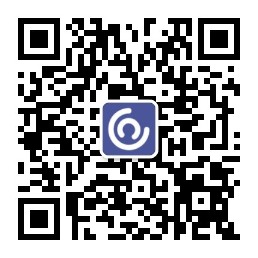
/**
* 定义一个结点类
* @author Administrator
*
*/
public class Link {
public int data; //数据域
public Link next; //指向下一个结点
public Link previous;
public Link(int data) {
super();
this.data = data;
}
//打印结点的数据域
public void displayLink() {
System.out.print("{"+ data + "} ");
}
}
双向链表的缺点就是每次插入或删除一个链结点的时候,要处理四个链结点的引用,而不是两个,也就是上面说过的四条线,这四条线分别为:newNode.previous(新结点的上一个结点)和上一个结点的(我们这里取名为current)current.next(当前结点的下一个结点),还有newNode.next(新结点的下一个结点)以及current.next.previous(当前结点下一个结点的previous域),很绕,可以结合上面所给的图来看,就会简单的多。
删除图,对照代码段deleteAfter来看:
完整代码:
/**
* 自定义一个双向链表
* @author Administrator
*
*/
public class DoublyLinkList {
private Link first;
private Link last;
public DoublyLinkList() {
first = null;
last = first;
}
//头部插入
public void insertFirst(int data) {
Link newNode = new Link(data);
if(isEmpty()) {
last = newNode;
}else {//如果不是第一个结点的情况
first.previous = newNode; //将还没插入新结点之前链表的第一个结点的previous指向newNode
}
newNode.next = first; //将新结点的next指向first
first = newNode; //将新结点赋给first(链接)成为第一个结点
}
//尾部插入
public void insertLast(int data) {
Link newNode = new Link(data);
if(isEmpty()) {
first = newNode; //若链表为空,则将first指向新的结点(newNode)
}else {
newNode.previous = last;//将last的previous指向last(last永远指向的是最后一个结点)【此时还没有插入新的结点newNode,所以last指向的是当前链表的最后一个结点】
last.next = newNode; //将last.next(当前链表最后一个结点的next域)指向新的结点newNode
}
last = newNode; //由于插入了一个新的结点,又因为是尾部插入,所以将last指向newNode
}
//某个结点的后部插入
public void insertAfter(int key,int data) {
Link newNode = new Link(data);
Link current = first;
while((current!=null)&&(current.data!=key)) {
current = current.next;
}
//若当前结点current为空
if(current==null) { //current为null有两种情况 一种是链表为空,一种是找不到key值
if(isEmpty()) { //1、链表为空
first = newNode; //则插入第一个结点(其实可以调用其它的Insert方法)
last = newNode; //first和last均指向该结点(第一个结点)
}else {
last.next = newNode; //2、找不到key值
newNode.previous = last; //则在链表尾部插入一个新的结点
last = newNode;
}
}else {
if(current==last) { //第三种情况,找到了key值,分两种情况 两
newNode.next = null; //1、key值与最后结点的data相等 条
last = newNode; //由于newNode将是最后一个结点,则将last指向newNode 线
}else {
newNode.next = current.next; //2、两结点中间插入 四
current.next.previous = newNode; //将current当前结点的下一个结点赋给newNode.next 条
} //将current下一个结点即current.next的previous域指向current 线
current.next = newNode; //将当前结点的next域指向newNode
newNode.previous = current; //将新结点的previous域指向current(current在newNode前面一个位置)
}
}
//检查链表是否为空
public boolean isEmpty() {
return (first==null);
}
//从头部删除结点
public Link deleteFirst() {
Link temp = first;
if(first.next==null) { //若链表只有一个结点,删除后链表为空,将last指向null
last = null;
}else {
first.next.previous = null; //若链表有两个(包括两个)以上的结点 ,因为是头部插入,则first.next将变成第一个结点,其previous将变成null
}
first = first.next; //将first.next赋给first
return temp; //返回删除的结点
}
//从尾部删除结点
public Link deleteLast() {
Link temp = last;
if(first.next == null) { //如果链表只有一个结点,则删除以后为空表,last指向null
first = null;
}else {
last.previous.next = null; //将上一个结点的next域指向null
}
last = last.previous; //上一个结点称为最后一个结点,last指向它
return temp; //返回删除的结点
}
//按值删除
public Link deleteKey(int key) {
Link current = first;
while(current!=null&¤t.data!=key) { //遍历链表寻找该值所在的结点
current = current.next;
}
if(current==null) { //若当前结点指向null则返回null,
return null; //两种情况当前结点指向null,一是该链表为空链表,而是找不到该值
}else {
if(current==first) { //如果current是第一个结点
first = current.next; //则将first指向它,将该结点的previous指向null,其余不变
current.next.previous = null;
}else if(current==last){ //如果current是最后一个结点
last = current.previous; //将last指向当前结点的上一个结点(我们将当前结点除名了以后它便不再是最后一个了)
current.previous.next = null; //相应的要删除结点的上一个结点的next域应指向null
}else {
current.previous.next = current.next; //当前结点的上一个结点的next域应指向当前的下一个结点
current.next.previous =current.previous; //当前结点的下一个结点的previous域应指向当前结点的上一个结点
}
}
return current; //返回
}
//从头部开始演绎
public void displayForward() {
System.out.print("List(first--->last): ");
Link current = first;
while(current!=null) {
current.displayLink();
current = current.next;
}
System.out.println();
}
//从尾部开始演绎
public void displayBackward() {
System.out.print("List(last--->first): ");
Link current = last;
while(current!=null) {
current.displayLink();
current = current.previous;
}
System.out.println();
}
}
测试代码:
/**
* 测试双向链表
* @author Administrator
*
*/
public class TestDoublyLinkList {
public static void main(String[] args) {
DoublyLinkList theList = new DoublyLinkList();
theList.insertFirst(20);
theList.insertFirst(40);
theList.insertFirst(60);
theList.insertLast(10);
theList.insertLast(30);
theList.insertLast(50);
theList.displayForward();
theList.displayBackward();
theList.deleteFirst();
theList.deleteLast();
theList.deleteKey(10);
theList.displayForward();
theList.insertAfter(20, 70);
theList.insertAfter(30, 80);
theList.displayForward();
theList.displayBackward();
}
}
测试结果如下:
List(first--->last): {60} {40} {20} {10} {30} {50}
List(last--->first): {50} {30} {10} {20} {40} {60}
List(first--->last): {40} {20} {30}
List(first--->last): {40} {20} {70} {30} {80}
List(last--->first): {80} {30} {70} {20} {40}