版权声明:本文为博主原创文章,未经博主允许不得转载。有事联系:[email protected] https://blog.csdn.net/qq_17550379/article/details/82927028
给定字符串 s 和 t ,判断 s 是否为 t 的子序列。
你可以认为 s 和 t 中仅包含英文小写字母。字符串 t 可能会很长(长度 ~= 500,000),而 s 是个短字符串(长度 <=100)。
字符串的一个子序列是原始字符串删除一些(也可以不删除)字符而不改变剩余字符相对位置形成的新字符串。(例如,"ace"
是"abcde"
的一个子序列,而"aec"
不是)。
示例 1:
s = "abc"
, t = "ahbgdc"
返回 true
.
示例 2:
s = "axc"
, t = "ahbgdc"
返回 false
.
后续挑战 :
如果有大量输入的 S,称作S1, S2, … , Sk 其中 k >= 10亿,你需要依次检查它们是否为 T 的子序列。在这种情况下,你会怎样改变代码?
解题思路
这个问题同样非常简单,我们只需要建立两个指针,一个指向s_p
,一个指向t_p
。
扫描二维码关注公众号,回复:
3427918 查看本文章
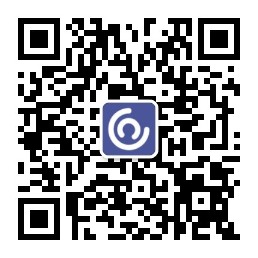
s: a b c
|
s_p
t: a h b g d c
|
t_p
然后我们不断挪动t_p
,看t_p
指向的元素和s_p
指向的元素是否相等,如果不等的话继续移动t_p
;否则,如果相等的话,我们挪动t_p
的同时也挪动s_p
,直到t_p
到了t
的边界。在此期间,如果s_p
到了s
的边界的话,我们直接返回True
了。如果整个循环结束,也就是t
遍历完都没有返回True
的话,说明不存在,我们返回False
就可以了。
class Solution:
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if s == None or t == None:
return False
len_s = len(s)
len_t = len(t)
if len_t < len_s:
return False
if len_s == 0:
return True
j = 0
for i in range(len_t):
if s[j] == t[i]:
j += 1
if j == len_s:
return True
return False
实际上,上述过程可以更加的简洁。我们使用内置函数find
,就可以快速定位字符的位置。
class Solution:
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
for per_s in s:
s_index_t = t.find(per_s)
if s_index_t == -1:
return False
if s_index_t == len(t) - 1:
t = str()
else:
t = t[s_index_t+1:]
return True
我将该问题的其他语言版本添加到了我的GitHub Leetcode
如有问题,希望大家指出!!!