1、打印
fmt.Println("asd")
fmt.Printf("asd\n")
fmt.Printf("asd is %s\n","aaa")
2、string和int互转
strconv.Itoa(1) //转字符串
strconv.Atoi("1") //转int
3、格式化日期
time.Now().Format("20060102150405")
4、睡眠
time.Sleep(time.Duration(waitTime)*time.Second)
5、随机数
"math/rand"
r:= rand.New(rand.NewSource(time.Now().UnixNano()))
r.Intn(100) //0-100随机数
6、tcp连接
"net"
...
conn, err := net.Dial("tcp", "ipport")
if err != nil {
fmt.Println("连接服务端失败:", err.Error())
return
}
fmt.Println("连接成功")
defer conn.Close()
Client(conn, tcpData)
...
func Client(conn net.Conn, sms string) {
fmt.Println("要发送的消息:"+sms)
conn.Write([]byte(sms))
buf:= make([]byte, 2)
c, err:= conn.Read(buf)
if err != nil {
fmt.Println("读取服务器数据异常:", err.Error())
}
fmt.Println("服务返回:"+string(buf[0:c]))
}
}
7、字符串分割
"strings"
strings.FieldsFunc(TOPIC["data"], split)
...
func split(s rune) bool {
if s == ',' {
return true
}
return false
}
8、字符串包含
扫描二维码关注公众号,回复:
3425557 查看本文章
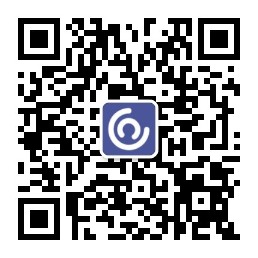
"strings"
...
fmt.Println(Strings.Contains("seafood", "foo")) //true
9、路径
wd, err:= os.Getwd()
这是定位到源码的project级别,编译完成后也是执行所在目录,这是相对路径的位置
10、多goroutine间通信,channel管道使用
package main
import (
"fmt"
"time"
)
func Producer(queue chan<- int) {
for i:=0; i<10; i++ {
queue <- i //写入
fmt.Println("create:", i)
}
}
func Consumer(queue <-chan int) {
for i:=0; i< 10; i++ {
v:= <- queue //读取
fmt.Println("receive:", v)
}
}
func main(){
queue:= make(chan int, 88) //创建管道
go Producer(queue) //创建协程
go Consumer(queue)
time.Sleep(1e9)
}
11、异常捕获
timeStart:= time.Now()
c:= make(chan os.Signal,1)
signal.Notify(c, os.Interrupt, os.Kill)
s:= <-c
timeEnd:= time.Now().Sub(timeStart)
fmt.Println("耗时:", timeEnd.Seconds())
fmt.Println("Got signal:", s)