Given an array, rotate the array to the right by k steps, where k is non-negative.
Example 1:
Input:[1,2,3,4,5,6,7]
and k = 3 Output:[5,6,7,1,2,3,4]
Explanation: rotate 1 steps to the right:[7,1,2,3,4,5,6]
rotate 2 steps to the right:[6,7,1,2,3,4,5]
rotate 3 steps to the right:[5,6,7,1,2,3,4]
Example 2:
Input: [-1,-100,3,99]
and k = 2
Output: [3,99,-1,-100]
Explanation:
rotate 1 steps to the right: [99,-1,-100,3]
rotate 2 steps to the right: [3,99,-1,-100]
Note:
- Try to come up as many solutions as you can, there are at least 3 different ways to solve this problem.
- Could you do it in-place with O(1) extra space?
给定一个数组,将数组中的元素向右移动 k 个位置,其中 k 是非负数。
示例 1:
输入:[1,2,3,4,5,6,7]
和 k = 3 输出:[5,6,7,1,2,3,4]
解释: 向右旋转 1 步:[7,1,2,3,4,5,6]
向右旋转 2 步:[6,7,1,2,3,4,5]
向右旋转 3 步:[5,6,7,1,2,3,4]
示例 2:
输入: [-1,-100,3,99]
和 k = 2
输出: [3,99,-1,-100]
解释:
向右旋转 1 步: [99,-1,-100,3]
向右旋转 2 步: [3,99,-1,-100]
说明:
- 尽可能想出更多的解决方案,至少有三种不同的方法可以解决这个问题。
- 要求使用空间复杂度为 O(1) 的原地算法。
1 class Solution { 2 func rotate(_ nums: inout [Int], _ k: Int) { 3 let numsCount = nums.count 4 if numsCount == 0 || k <= 0 5 { 6 return 7 } 8 var numsCopy = nums 9 for i in 0..<numsCount 10 { 11 numsCopy[i] = nums[i] 12 } 13 for j in 0..<numsCount 14 { 15 nums[(j + k)%numsCount] = numsCopy[j] 16 } 17 } 18 }
16ms
1 class Solution { 2 func rotate(_ nums: inout [Int], _ k: Int) { 3 let index = nums.count - 1 - (k % nums.count) 4 nums += nums[0...index] 5 nums.removeSubrange((0...index)) 6 } 7 }
20ms
1 class Solution { 2 func rotate(_ nums: inout [Int], _ k: Int) { 3 let realK = k % nums.count 4 let sourceNums = nums 5 for i in 0 ..< sourceNums.count { 6 nums[(i+k) % sourceNums.count] = sourceNums[i] 7 } 8 } 9 }
12ms
扫描二维码关注公众号,回复:
3415533 查看本文章
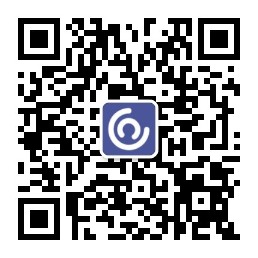
1 class Solution { 2 func rotate(_ nums: inout [Int], _ k: Int) { 3 guard nums.count > 1 else { 4 return 5 } 6 7 guard k > 0 else { 8 return 9 } 10 11 let k = k % nums.count 12 nums = Array(nums[(nums.count - k)..<nums.count]) + Array(nums[0..<(nums.count - k)]) 13 14 // O(k % nums.count) extra space 15 /* 16 let shiftOffset = k % nums.count 17 let temp = Array(nums[(nums.count - shiftOffset)..<nums.count]) 18 for i in (shiftOffset..<nums.count).reversed() { 19 nums[i] = nums[i - shiftOffset] 20 } 21 22 for i in 0..<shiftOffset { 23 nums[i] = temp[i] 24 }*/ 25 26 } 27 }