版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/chenlinIT/article/details/80707352
CommonJS最初是在服务器端实现,Node.js就是基于CommonJS规范开发的,将CommonJS运用在浏览器端需要使用Browserify进行编译打包。让我们来学习一下如何使用CommonJS规范在服务器端(Node.js)和浏览器端进行模块化开发。
在node中使用
//文件目录
|-commonjs_in_node
|-modules
|-module1.js
|-module2.js
|-module3.js
|-module4.js
|-app.js
|-package.json
暴露模块
1、module.exports = value
//module1.js
//定义一个模块,向外面暴露一个对象,module.exports={}
module.exports = {
msg: '模块1',
arr: [1,5,3,2,6,2,3,6,7,9,4],
fun: function(){
console.log(this.msg);
}
};
//module2.js
//定义一个模块直接暴露一个方法
module.exports = function () {
console.log('模块2');
};
2、exports.xxx = value
//module3.js
//使用exports.xxx = value的方式暴露接口,实际上是为exports添加属性,exports为一个对象。
exports.foo = function(){
console.log('模块3 foo()')
}
exports.bar = function(){
console.log('模块3 bar()')
}
引入模块,依赖
再定义一个模块4,依赖模块1
//引入依赖模块 module1
var module1 = require('./module1')
//module4中的run方法依赖module1中的arr属性。通过传入一个方法参数对arr进行操作。
exports.run = function(f){
var _arr = f(module1.arr);
console.log(_arr);
}
使用自定义模块及第三方模块
安装第三方模块
使用npm安装第三方包uniq,这个包的功能是对数组进行去重并排序。
//命令行
cd commonjs_in_node
//创建package.json
npm init
//安装uniq包
npm install uniq --save
//package.json
{
"name": "commonjs_in_node",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"uniq": "^1.0.1"
}
}
使用模块
//app.js
//引入第三方模块
var uniq = require('uniq');
//引入自定义模块
var module1 = require('./modules/module1');
var module2 = require('./modules/module2');
var module3 = require('./modules/module3');
var module4 = require('./modules/module4');
module1.fun();//module1暴露的是对象,调用需要用 obj.xx 的方式调用
module2();//module2暴露的是一个方法,可以直接调用
//module3本质上也是暴露的对象。
module3.foo();
module3.bar();
module4.run(uniq);
console.log(uniq(module1.arr))
引入第三方模块建议写在自定义模块上面。只需要require('包名')
,引入自定义模块需要require('模块路径')
在node中使用方法总结
在node中使用就这么简单,使用module.exprots = value
或者exprots.xxx = value
暴露模块,然后使用require('模块路径')
(自定义模块)或者require('包名')
(第三方模块)引入模块。使用的话要具体看模块暴露出来的value是什么类型。
在浏览器端使用
//文件目录
|-commonjs_in_browser
|-js
|-dist //打包生成的文件目录
|-src //源码目录
|-module1.js
|-module2.js
|-module3.js
|-module4.js
|-app.js
|-index.html
|-package.json
定义的方法和在node中是相同的,我们把上面的几个模块的路径修改一下。
//module1.js
module.exports = {
msg: '模块1',
arr: [1,5,3,2,6,2,3,6,7,9,4],
fun: function(){
console.log(this.msg);
}
};
//module2.js
module.exports = function () {
console.log('模块2');
};
//module3.js
exports.foo = function(){
console.log('模块3 foo()')
}
exports.bar = function(){
console.log('模块3 bar()')
}
//module4.js
var module1 = require('./module1')
exports.run = function(f){
var _arr = f(module1.arr);
console.log(_arr);
}
//app.js
var uniq = require('uniq');
//引入自定义模块,主要这里的路径和上面是不同的。
var module1 = require('./module1');
var module2 = require('./module2');
var module3 = require('./module3');
var module4 = require('./module4');
module1.fun();
module2();
//module3本质上也是暴露的对象。
module3.foo();
module3.bar();
module4.run(uniq);
console.log(uniq(module1.arr))
安装第三方包的方法是相同的,在浏览器中使用需要先用browserify将代码编译打包然后再在html中引用。安装browserify包要注意,需要全局安装然后再局部安装,需要安装两次。
//安装uniq
npm i uniq --save
//安装browserify(全局)
npm i browserify -g
//安装browserify
npm i browserify --save-dev
安装完后使用browserify 命令进行打包编译。
//语法:browserify 入口文件路径 -o 输出路径,bundle.js为输出文件的名称,可以随便定义。
browserify js/src/app.js -o js/dist/bundle.js
在html中引入打包后的js文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script src="./js/dist/bundle.js"></script>
</body>
</html>
在浏览器中打开,开发者工具中console中查看结果。
在浏览器中使用方法总结
浏览器中使用和node中使用相比就是多了一个编译打包的步骤,使用browserify的方法是 browserify 入口文件路径 -o 输出路径
。
扫描二维码关注公众号,回复:
3381039 查看本文章
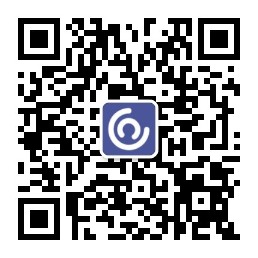