目录:
一 、json简介
1.JSON来历:JSON(JavaScript Object Notation, JS 对象标记) 是一种轻量级的数据交换格式。它基于 ECMAScript (w3c制定的js规范)的一个子集,采用完全独立于编程语言的文本格式来存储和表示数据。简洁和清晰的层次结构使得 JSON 成为理想的数据交换语言。
2.JSON作用:JSON对象作为一种通用的中间层,用于跨平台跨语言传输数据
3.JSON语法规范:JSON种有四种基础类型(string,boolean,int,null),有两种复杂类型(array,Object),由于不同平台不同语言的差异性,在各自语言有各自对JSON的理解,Object类型可以理解为对象,也可以认为是哈希表,键值对等。复杂类型之间可以互相嵌套,比如array中含有多个Object,而其中的Object又含有array.
4.Linux下C++中使用JSON:有多种方案,这里使用jsoncpp开源跨平台框架, jsoncpp的安装参考如下链接:linux下正确安装jsoncpp框架,或者自己搜索相关博客安装
5.使用JSONCPP框架: 参考上述链接将其安装后,其动态链接库libjson.so已在系统默认库中(/lib/),相关头文件也在默认系统头文件库中( /usr/include/),此时只需在代码中保护相应头文件,如#include < json/json.h>,在链接时加上-ljson参数链接上libjson.so库即可,例如
g++ -c main.cpp -o main.o #编译
g++ -o main main.o -ljson #链接
./main #运行
二 、直接赋值产生json对象
jsoncpp中使用JSON::Value类型来包含Json对象,如下产生简单的Json对象
#include <iostream>
#include <cstdio>
#include <json/json.h>
using namespace std;
int main()
{
Json::Value a,b,c,d,e;
a = 12; //int
b = "hello";//string
c = false; //boolean
d = true; //boolean
//eΪnull
cout << "First" << endl;
cout << a.asInt() << endl;
cout << b.asString() << endl;
cout << c.asString() << endl;
cout << d.asString() << endl;
cout << e.asString() << endl;
//也可以使用复制构造函数来初始化生成JSON对象
a = Json::Value(13);
b = Json::Value("hello world");
c = Json::Value(false);
d = Json::Value(true);
e = Json::Value();
cout << "Second" << endl;
cout << a.asInt() << endl;
cout << b.asString() << endl;
cout << c.asString() << endl;
cout << d.asString() << endl;
cout << e.asString() << endl;
return 0;
}
运行结果如下所示:
三 、从JSON字符流中读取json对象
使用过JSON都知道,JSON对象传输时的形态为字符流,从JSON字符流中取出JSON对象具有重要应用意义
jsoncpp中使用Json::Reader类型来读取字符流中的数据,如下
#include <iostream>
#include <string>
#include <json/json.h>
using namespace std;
int main()
{
string message = "{ \"data\" : { \"username\" : \"test\" }, \"type\" : 6 }";
Json::Reader reader;
Json::Value value;
//如果message符合Json对象语法,则取出该对象存在value中
if(reader.parse(message.c_str(), value))
{
Json::Value temp = value["data"]; //取出value对象中的data对象,对象嵌套的一种方式
cout << "username:" << temp["username"].asString() << endl;
cout << "type:" << value["type"].asInt() << endl;
cout << "id:" << value["id"].asString() << endl; //取出null
}
return 0;
}
结果如下所示:
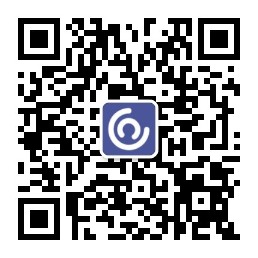
四 、将json对象转为字符流
将json对象转为字符流才能用于传输交互,代码如下
采用Json::Value对象自带的toStyledString()转变成带有格式的JSON字符流
或者
使用Json::FastWriter类型进行操作,将Json对象写为字符流
#include <iostream>
#include <json/json.h>
#include <string>
using namespace std;
int main()
{
Json::Value value;
//使用赋值的方式产生JSON对象
value["name"] = "kangkang";
Json::Value data;
data["id"] = "20140801"; //账号
data["pwd"] = "123456"; //密码
value["data"] = data; //对象嵌套
//使用toStyledString()函数直接将value对象转为JSON字符流
cout << "toStyledString()" << endl;
cout << value.toStyledString() << endl;
//使用Json::FastWriter类型
Json::FastWriter fw;
cout << "Json::FastWriter()" << endl;
cout << fw.write(value) << endl;
return 0;
}
结果如下
通常Json::FastWriter产生的格式才是我们传输过程中使用的格式。