I /O函数
一、fopen()和fclose()
1.fopen():这个函数的作用是打开流。
FILE* fopen( const char* filename, const char* mode);
这个函数有两个参数,第一个参数是需要打开的文件名,例如test.txt;第二个参数是指打开文件时是以什么类型打开的,他的返回类型是一个结构体指针。
打开文件类型:
- w–以只写的类型打开,在已有的内容后面追加。
- r –以只读的类型打开。
- a–以只写的形式的开,再写之前会清空文件。
- wb–以二进制写入的类型打开。
- rb–以二进制读的类型打开。
2.fclose():这个函数的作用是关闭流。
int fclose( FILE* pf);
这个函数的参数只有一个,是一个结构体的指针。返回类型为int。
3.代码演示:
#include<stdio.h>
#include<errno.h>
#include<stdlib>
int main()
{
FILE* pf = fopen("test.txt", "w");//打开流
if(pf==NULL)
{
perror("open file for write")
exit(EXIT_FAILURE):
//写
fclose(pf);//关闭流
pf = NULL;
return 0;
}
创建打开了test.txt文件。
二、字符I\O
(一)、getchar()和putchar()
1.getchar()是字符输入函数,适用于标准输入流(stdin),也就是说只能在键盘上写入,不能写入文件。
2.putchar()是字符输入函数,适用于标准输出流(stdout),也就是说只能输出到屏幕而不能在文件中读取。
3.代码演示:
#include<stdio.h>
int main()
{
char ch = 0;
ch=getchar();
putchar(ch);
return 0;
}
(二)、gtec()和putc()
1.getc()是字符输入函数,适用于所有流。既可以在键盘上输入,同时可以从文件读入。
int getc( FILE* pf);
代码演示:
#include<stdio.h>
#include <errno.h>
#include<stdlib.h>
int main()
{
char ch = 0;
FILE* pf=fopen("test.txt","r");
if (pf==NULL)
{
perror("open file for read");
exit(EXIT_FAILURE);
}
ch = getc(pf);
printf("%c\n", ch);
ch = getc(stdin);
fclose(pf);
pf = NULL;
return 0;
}
我从test.txt读取了a,从键盘上输入了i。
2.putc()是字符输出函数,适用于所有输出流。既可以在屏幕上输出也可以在写入文件。
int putc(int c,FILE* pf);
把c写道文件中去。
代码演示:
#include<stdio.h>
#include <errno.h>
#include<stdlib.h>
int main()
{
char ch = 0;
FILE* pf=fopen("test.txt","w");
if (pf==NULL)
{
perror("open file for write");
exit(EXIT_FAILURE);
}
putc('a',pf);
fclose(pf);
pf = NULL;
return 0;
}
我把字符a即写到了文件中,又输出到了屏幕上。
(三)、fgetc()和fputc()
int fgetc( FILE* pf);
int fputc(int c,FILE* pf);
getc()和fgetc()的功能一样。
putc()和fputc()的功能一样。
这里我就不再举例了。
三、未格式化的行I/O
(一)、gets()和puts()
1.gets():文本行输入函数,只适用于标准输入流。只能从键盘输入而不能从文本读取。
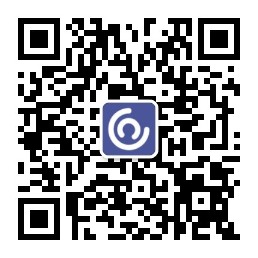
int puts( char* str);
代码演示:
int main()
{
char arr[20];
gets(arr);
printf("%s\n", arr);
return 0;
}
2.puts():文本行输出函数,只适用于标准输出流。只能在屏幕输出,而不能写入文件。
char* puts( const char* str);
代码演示:
int main()
{
char arr[]="hello world";
puts(arr);
return 0;
}
(二)、fgets()和fputs()
1.fgets():文本行输入函数,适用于所有输入流。既能从键盘输入,也能从从文件中读取。
char* fgets( char* str,int num, FILE*pf);
代码演示:
#define _CRT_SECURE_NO_DEPRECATE 1
#include<stdio.h>
#include <errno.h>
#include<stdlib.h>
int main()
{
char str[20];
FILE* pf = fopen("test.txt", "r");
if (pf == NULL)
{
perror("open file for read");
exit(EXIT_FAILURE);
}
fgets(str,10, pf);
printf("%s\n", str);
fgets(str,10,stdin);
fclose(pf);
pf = NULL;
return 0;
}
2.fputs():文本行输出函数,适用于所有输出流。既能在屏幕输出,又可以写入文件。
int fputs(const char* str, FILE* pf);
代码演示:
#define _CRT_SECURE_NO_DEPRECATE 1
#include<stdio.h>
#include <errno.h>
#include<stdlib.h>
int main()
{
char str[]="gsdsdysgusy";
FILE* pf = fopen("test.txt", "w");
if (pf == NULL)
{
perror("open file for write");
exit(EXIT_FAILURE);
}
fputs(str,pf);
fputs(str,stdout);
printf("\n");
fclose(pf);
pf = NULL;
return 0;
}
四、格式化的行I\O
(一)、scanf()和printf()
他们两个很常见,是格式化输入/输出函数。适用于标准输入/输出流,就不再介绍了。
int scanf(char* format, ...);
int printf( char* format, ...);
(二)、fscanf()和fprintf()
1.fscanf():格式化输入函数,适用于所有输入流。既可以在键盘输入,又可以从文件读取。
int fscanf( FILE* pf , char* format, ...);
代码演示:
#define _CRT_SECURE_NO_DEPRECATE 1
#include "errno.h"
#include "stdlib.h"
#include<stdio.h>
int main()
{
char str[100];
int m = 0;
int n = 0;
FILE* pf = fopen("test.txt", "r+");
if (pf == NULL)
{
perror("open file for read");
exit(EXIT_FAILURE);
}
fscanf(pf, "%s", str);
fscanf(pf, "%d", &m);
printf("%s %d ", str, m);
printf("\n");
fscanf(stdin, "%d", &n);
fclose(pf);
pf = NULL;
return 0;
}
2.fprintf():格式化输出函数,适用于所有输出流。既能在屏幕输出,又能写入文件。
int fprintf(FILE* pf,char* format,...);
代码演示:
#define _CRT_SECURE_NO_DEPRECATE 1
#include "errno.h"
#include "stdlib.h"
#include<stdio.h>
int main()
{
char str[]="hello world";
int m = 100;
char c = 'a';
FILE* pf = fopen("test.txt", "w+");
if (pf == NULL)
{
perror("open file for write");
exit(EXIT_FAILURE);
}
fprintf(pf, "%s %d %c", str, m, c);
fprintf(stdout, "%s\n%d\n%c\n", str, m, c);
fclose(pf);
pf = NULL;
return 0;
}
还有两个函数和他们挺形似:sscanf()和sprintf()
这两个是内存函数,下个博客在细说。
sscanf和sprintf函数的使用
四、二进制I/O
fread():二进制输入,只适用于文件。
fwrite():二进制输入,只适用于文件。
size_t fread( void* str, size_t size, size_t count,pf);
size_t fwrite( const void* str, size_t size, size_t count,pf);
代码演示:
#include<stdio.h>
#include<stdlib.h>
#include "string.h"
int main()
{
FILE* pf = fopen("test.txt", "wb");
if (pf == NULL)
{
perror("open file for write");
exit(EXIT_FAILURE);
}
typedef struct Stu
{
char name[25];
int age;
char tel[12];
}Stu ;
Stu stu;
strcpy(stu.name, "zhangsan");
stu.age = 10;
strcpy(stu.tel, "123456");
fwrite(stu.name, sizeof(stu.name), 1, pf);
fwrite(&stu.age, sizeof(stu.age), 1, pf);
fwrite(stu.tel, sizeof(stu.tel), 1, pf);
fclose(pf);
pf = NULL;
return 0;
}
#include<stdio.h>
#include<stdlib.h>
int main()
{
FILE* pf = fopen("test.txt", "rb");
if (pf == NULL)
{
perror("open file for read");
exit(EXIT_FAILURE);
}
typedef struct Stu
{
char name[25];
int age;
char tel[12];
}Stu;
Stu stu = { 0 };
fread(stu.name, sizeof(stu.name), 1, pf);
fread(&stu.age, sizeof(stu.age), 1, pf);
fread(stu.tel, sizeof(stu.tel), 1, pf);
printf("%s\n", stu.name);
printf("%d\n", stu.age);
printf("%s\n", stu.tel);
fclose(pf);
pf = NULL;
return 0;
}