20.5 shell脚本中的逻辑判断
格式1:if条件;then语句;fi(其中if和fi相对应)
[root@hyc-01-01 ~]# for i in `seq 1 5`
> do
> echo $i
> done
1
2
3
4
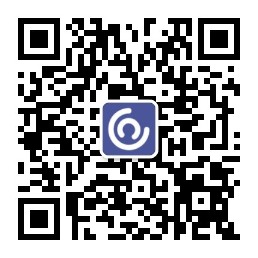
5 [root@hyc-01-01 ~]# for i in `seq 1 5`; do echo $i; done
1
2
3
4
5
用;分隔一次执行中的多条命令
[root@hyc-01-01 ~]# a=5
[root@hyc-01-01 ~]# if [ $a -gt 3 ]
> then
> echo ok
> fi
Ok
[root@hyc-01-01 ~]# a=5;if [ $a -gt 3 ];then echo ok;fi
ok
Shell中符号和词、符号与符号、词与词间需要用空格分隔开;
-gt在shell中表示>(大于号),-lt在shell中表示<(小于号);
-eq在shell中表示=(等于号),-ne在shell中表示不等于;
-ge在shell中表示>=(大于等于),-le在shell中表示<=(小于等于);
编写shell脚本
[root@hyc-01-01 shell]# vim if1.sh
#!/bin/bash
A=5
if
[ $a -gt 3 ]
then
echo ok
fi
格式2:if 条件;then 语句;else 语句;fi
[root@hyc-01-01 shell]# vim if2.sh
#!/bin/bash
a=5
if
[ $a -gt 3 ]
then
echo ok
else
echo not ok
fi
a=5时:
[root@hyc-01-01 shell]# sh if2.sh
ok
a=2时:
[root@hyc-01-01 shell]# sh -x if2.sh 查看执行过程
+ a=2
+ '[' 2 -gt 3 ']'
+ echo not ok
not ok
格式3:if …;then …;elif …;then …;else …;fi
注:if不匹配则顺序执行elif,elif不匹配执行else
[root@hyc-01-01 shell]# vim if3.sh
#!/bin/bash
a=4
if
[ $a -gt 3 ]
then
echo "a>3"
elif
[ $a -lt 6 ]
then
echo "a>=3且a<6"
else
echo not ok
fi
a=4时:
[root@hyc-01-01 shell]# sh -x if3.sh
+ a=4
+ '[' 4 -gt 3 ']'
+ echo 'a>3'
a>3
a=2时:
[root@hyc-01-01 shell]# sh -x if3.sh
+ a=2
+ '[' 2 -gt 3 ']'
+ '[' 2 -lt 6 ']'
+ echo 'a<=3且a<6'
a<=3且a<6
[root@hyc-01-01 shell]# vim if3.sh
#!/bin/bash
a=2
if
(($a>3))
then
echo "a>3"
elif
[ $a -lt 6 ]
then
echo "a<=3且a<6"
else
echo not ok
fi
写法(($a>3))等同于[ $a -gt 3 ];
使用$a>3写法时一定要用两个()括起来;
结合多个条件
&& 表示当前一条命令成功才执行后面的命令
|| 表示当前一条命令不成功时执行后面的命令
20.6 文件目录属性判断
-f 判断是否为文件
[root@hyc-01-01 shell]# vim file.sh
#!/bin/bash
f="/tmp/noexist"
if
[ -f $f ]
then
echo $f is exist
else
touch $f
fi
[root@hyc-01-01 shell]# sh -x file.sh
+ f=/tmp/noexist
+ '[' -f /tmp/noexist ']'
+ touch /tmp/noexist
再次执行
[root@hyc-01-01 shell]# sh -x file.sh
+ f=/tmp/noexist
+ '[' -f /tmp/noexist ']'
+ echo /tmp/noexist is exist
/tmp/noexist is exist
-d 判断文件是否为一个目录
[root@hyc-01-01 shell]# vim file1.sh
#!/bin/bash
f="/tmp/directory"
if
[ -d $f ]
then
echo $f is exist
else
mkdir $f
fi
[root@hyc-01-01 shell]# sh -x file1.sh
+ f=/tmp/directory
+ '[' -d /tmp/directory ']'
+ mkdir /tmp/directory
[root@hyc-01-01 shell]# sh -x file1.sh
+ f=/tmp/directory
+ '[' -d /tmp/directory ']'
+ echo /tmp/directory is exist
/tmp/directory is exist
-e 判断一个文件或目录是否存在,! –e判断文件或目录不存在
[root@hyc-01-01 shell]# vim file1.sh
#!/bin/bash
f="/tmp/directory"
if
[ -e $f ]
then
echo $f is exist
else
touch $f
fi
[root@hyc-01-01 shell]# ls /tmp/directory
ls: 无法访问/tmp/directory: 没有那个文件或目录
[root@hyc-01-01 shell]# sh -x file1.sh
+ f=/tmp/directory
+ '[' -e /tmp/directory ']'
+ touch /tmp/directory
[root@hyc-01-01 shell]# ls -l /tmp/directory
-rw-r--r-- 1 root root 0 9月 19 23:19 /tmp/directory
[root@hyc-01-01 shell]# sh -x file1.sh
+ f=/tmp/directory
+ '[' -e /tmp/directory ']'
+ echo /tmp/directory is exist
/tmp/directory is exist
touch
使用touch命令时,若文件或目录不存在,将创建该文件;
若文件或目录存在,将更新文件或目录的atime、mtime、ctime;
[root@hyc-01-01 shell]# vim file1.sh
#!/bin/bash
f="/tmp/directory"
if
[ -e $f ]
then
echo "$f is exist" && touch $f
else
touch $f
fi
[root@hyc-01-01 shell]# ls -ld /tmp/directory/
drwxr-xr-x 2 root root 6 9月 19 23:34 /tmp/directory/
[root@hyc-01-01 shell]# sh -x file1.sh
+ f=/tmp/directory
+ '[' -e /tmp/directory ']'
+ echo '/tmp/directory is exist'
/tmp/directory is exist
+ touch /tmp/directory
[root@hyc-01-01 shell]# ls -ld /tmp/directory/
drwxr-xr-x 2 root root 6 9月 19 23:35 /tmp/directory/
-r 文件是否可读
[hyc@hyc-01-01 ~]$ whoami
hyc
[hyc@hyc-01-01 ~]$ vim file.sh
#!/bin/bash
f="/home/hyc/test"
if
[ -r $f ]
then
echo "$f is readable"
else
chmod a+r $f
fi
[hyc@hyc-01-01 ~]$ ls -l test
--w--w---- 1 hyc hyc 0 9月 19 23:57 test
[hyc@hyc-01-01 ~]$ sh -x file.sh
+ f=/home/hyc/test
+ '[' -r /home/hyc/test ']'
+ chmod a+r /home/hyc/test
[hyc@hyc-01-01 ~]$ sh -x file.sh
+ f=/home/hyc/test
+ '[' -r /home/hyc/test ']'
+ echo '/home/hyc/test is readable'
/home/hyc/test is readable
-w 文件是否可写
-x 文件是否可执行
!
[root@hyc-01-01 shell]# vim file.sh
#!/bin/bash
f="/tmp/noexist"
if
[ ! -f $f ] 若判断文件不存在,执行then,否则执行else
then
echo $f is not exist
else
echo $f is exist
fi
-r/-w/-x这三个判断用于均判断当前用户下是否可读、可写、可执行
20.7
-n与-z是两个相对的判断:
-z 当变量的值为空时怎样
-n 当变量的值非空时怎样
-z
[root@hyc-01-01 ~]# cat /tmp/test|wc -l
704
[root@hyc-01-01 ~]# vim zn.sh
#!/bin/bash
n=`cat /tmp/test|wc -l`
if
[ -z "$n" ] 一般使用-n或-z判断时最好给$n等变量加“”(双引号)
then
echo error
exit
elif
[ $n -gt 100 ]
then
echo "n>100"
else
echo "n<=100"
fi
[root@hyc-01-01 ~]# sh -x zn.sh
++ cat /tmp/test
++ wc -l
+ n=704
+ '[' -z 704 ']'
+ '[' 704 -gt 100 ']'
+ echo 'n>100'
n>100
经证实,-n和-z只能作用于变量,无法作用于文件
-n
逻辑判断时用命令的结果作为判断条件:
判断系统用户中有user1用户:
[root@hyc-01-01 ~]# vim user1.sh
#!/bin/bash
if
grep -wq 'user1' /etc/passwd
then
echo "user1 exist"
else
echo "no user1"
fi
[root@hyc-01-01 ~]# sh -x user1.sh
+ grep -wq user1 /etc/passwd
+ echo 'no user1'
no user1
判断系统用户中没有user1用户:
[root@hyc-01-01 ~]# vim user1.sh
#!/bin/bash
if
! grep -wq 'user1' /etc/passwd
then
echo "no user1"
else
echo "user1 exist"
fi
[root@hyc-01-01 ~]# sh -x user1.sh
+ grep -wq user1 /etc/passwd
+ echo 'no user1'
no user1
[]不能使用<,>,==,!=,>=.<=这样的符号
一个=为赋值,==才表示等于
20.8 case判断(上)
在/etc/init.d/network中包含case语句
[root@hyc-01-01 ~]# /etc/init.d/network start
以上命令中start为参数
[root@hyc-01-01 ~]# less /etc/init.d/network
…
case "$1" in $1表示第一个参数
start) 判断当第一个参数为start时执行以下脚本
[ "$EUID" != "0" ] && exit 4
rc=0
# IPv6 hook (pre IPv4 start)
if [ -x /etc/sysconfig/network-scripts/init.ipv6-global ]; then
/etc/sysconfig/network-scripts/init.ipv6-global start pre
fi
apply_sysctl
…
restart|reload|force-reload)
当判断参数为这三个其中一个时执行以下脚本;
case程序中可以使用|表示或者;
cd "$CWD"
$0 stop
$0 start
rc=$?
;; 双分号表示以上判断结束
…
*) 当出现以上case不包含的参数时执行以下脚本
echo $"Usage: $0 {start|stop|status|restart|reload|force-reload}"
exit 2
esac
exit $rc
编写一个case的shell脚本:
[root@hyc-01-01 ~]# vim case.sh
#!/bin/bash
read -p "Please input a number: " n
让用户输入值并捕获这个值,将该值赋给变量n
if [ -z "$n" ]
-z判断变量n为空,说明用户没有输入任何值
then
echo "Please input a number."
exit 1
每条语句执行结束都会返回一个值,exit 1表示执行结束返回的值为1,此时echo $?获得的值为1,若不执行该命令,则echo $?获得的值为0
fi
n1=`echo $n|sed 's/[0-9]//g'`
判断用户输入的字符串是否为纯数字,将用户输入的变量n中的数字部分清空
if [ -n "$n1" ]
若清空数字后的变量n1不为空,则执行以下操作
then
echo "Please input a number."
exit 1
返回状态码1并退出
fi
if [ $n -lt 60 ] && [ $n -ge 0 ]
如果分属小于60大于等于0
then
tag=1 给tag赋值1
elif [ $n -ge 60 ] && [ $n -lt 80 ]
then
tag=2
elif [ $n -ge 80 ] && [ $n -lt 90 ]
then
tag=3
elif [ $n -ge 90 ] && [ $n -le 100 ]
then
tag=4
else
tag=0
fi
case $tag in
1)
echo "不及格"
;;
2)
echo "及格"
;;
3)
echo "良好"
;;
4)
echo "优秀"
;;
*)
echo "值的范围是0-100."
;;
Esac
关于case脚本:
read -p
[root@hyc-01-01 ~]# read -p "Please input a number: " n
Please input a number: 233
[root@hyc-01-01 ~]# echo $n
233
[root@hyc-01-01 ~]# vim test.sh
#!/bin/bash
read -p "Please input a number: " n
if [ -z "$n" ]
then
echo "Please input a number."
fi
[root@hyc-01-01 ~]# sh test.sh
Please input a number: 66
[root@hyc-01-01 ~]# echo $?
0
当命令被成功执行时,echo $?返回的值为0,否则返回除0以外其他的值
20.9 case判断(下)