MongoDB概述
Getting started
准备环境
学习之前需要,安装MongoDB,可参考:https://docs.mongodb.com/manual/installation/
MongoDB 客户端可以安装Robo 3T;
插入多个文档
db.collection.insertMany()
命令用于在集合中一次插入多个文档,当未指定MongoDB 的“_id”时,将会自动生成_id字段。
如在inventory
集合中插入多个文档,返回插入文档的id列表:
db.inventory.insertMany([
// MongoDB adds the _id field with an ObjectId if _id is not present
{ item: "journal", qty: 25, status: "A",
size: { h: 14, w: 21, uom: "cm" }, tags: [ "blank", "red" ] },
{ item: "notebook", qty: 50, status: "A",
size: { h: 8.5, w: 11, uom: "in" }, tags: [ "red", "blank" ] },
{ item: "paper", qty: 100, status: "D",
size: { h: 8.5, w: 11, uom: "in" }, tags: [ "red", "blank", "plain" ] },
{ item: "planner", qty: 75, status: "D",
size: { h: 22.85, w: 30, uom: "cm" }, tags: [ "blank", "red" ] },
{ item: "postcard", qty: 45, status: "A",
size: { h: 10, w: 15.25, uom: "cm" }, tags: [ "blue" ] }
]);
db.collection.insertOne()用于插入单个文档。
查询文档
查询所有集合文档
db.collection.find()
用于查询集合所有文档
db.inventory.find( {} )
精确查询key:value
准确查找时,需要指定find()方法中的查询过滤条件,field:value的形式给定,如查询status=“D”文档;
db.inventory.find( { status: "D" } )
嵌入文档查询
通过嵌入的文档作为查询条件,准确查询文档记录,如查询size为{ h: 14, w: 21, uom: "cm" }的文档记录,注意,查询会考虑字段顺序,和{ w: 21, h: 14, uom: "cm" }为两个不同的查询条件:
db.inventory.find( { size: { h: 14, w: 21, uom: "cm" } } )
不同于:
db.inventory.find( { size: { w: 21, h: 14, uom: "cm" } } )
嵌入文档的字段查询
查询tags
数组中包含“red”的所有文档
db.inventory.find( { tags: "red" } )
精准查询tags时,
Match an Array Exactly,通过整个数组作为tags的查询,查询时需要
精确匹配元素顺序
:
db.inventory.find( { tags: ["red", "blank"] } )
不同于:
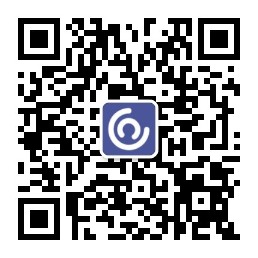
db.inventory.find( { tags: ["blank","red"] } )
二者查询结果不同。
Databases and Collections
mongoDB 数据库中的集合,集合中的记录存储的是BSON文档。
MongoDB数据库
数据库中包含着多个集合(ie,集合可以看做是关系数据库中的表),通过命令use <db>可以选择数据库,如:
use mydb
创建数据库
若数据库不存在,则MongoDB会在第一次插入数据的时候自动创建数据库。所以,可以直接使用一个未创建的数据库,并插入数据, 如:
use myNewDB
db.myNewCollection1.insertOne( { x: 1 } )
如果集合myNewCollection1也不存在,则insertOne在插入数据前,会自动创建集合myNewCollection1。
当然,还有其他操作可以创建数据库及集合,后面将详细阐述。
MongoDB集合
如果集合不存在,则MongoDB在存入数据前,会自动创建集合,如:
db.myNewCollection2.insertOne( { x: 1 } )
db.myNewCollection3.createIndex( { y: 1 } )
insertOne,createIndex均可以自动创建集合。
自定义创建集合
MongoDB提供命令来 db.createCollection()
准确创建集合, 可以设置一些创建参数,如设置集合大小,集合文档的检验等。如果不需要特别指定一些参数,并不需要自定义创建集合,使用自动创建即可。当然可以通过一些其他命令对集合进行修改。
集合创建之后,MongoDB会为该集合分配一个固定唯一的标识,一个UUID字符串,可以通过db.getCollectionInfos()
命令查看,查看所有集合的详细信息。
MongoDB视图
创建视图
MongoDB提供以下创建视图的命令:
db.runCommand( { create: <view>, viewOn: <source>, pipeline: <pipeline> } )
或者指定集合创建:
db.runCommand( { create: <view>, viewOn: <source>, pipeline: <pipeline>, collation: <collation> } )
或者通过新版视图创建命令:
db.createView(<view>, <source>, <pipeline>, <collation> )
视图特性
只读:视图是只读的,对视图进行写操作将会报错,视图支持以下操作:
db.collection.find()
db.collection.findOne()
db.collection.aggregate()
db.collection.count()
db.collection.distinct()
索引及排序:
- 视图使用集合的索引;
- 视图不可创建,删除,或者重建索引;
- 视图名字固定,不可以重命名;
- 不能为视图指定自然排序,a
$natural
sort ,以下操作是无效的;
db.view.find().sort({$natural: 1})
视图支持的操作:
定容集合(Capped collections)
定容集合是固定大小的集合,支持高吞吐量操作,如基于存储数据顺序的插入数据和获取数据操作。定容集合工作方法类似循环缓存(circular buffers):当所分配的存储满时,将自动覆盖最早的文档,以此为新写入的文档提供存储空间。
特性
insertion order
定容集合保证插入顺序(insertion order),所以当按插入顺序查询文档时,不需要额外的索引。没有了这项索引的开销,定容集合自然可以支持更高的存储吞吐量。
自动清理最早的文档
定容集合将自动覆盖最早的文档,以此为新写入的文档提供存储空间,不需要额外的执行脚本辅助。
例如在以下场景可以考虑使用定容集合:
- 存储高通量系统日志。插入log文档而无需索引,将直接提高写日志速度;先入先出(first-in-first-out)特性可以维持事件发生的顺序;
- 缓存定容集合少量数据。对读多写少的缓存操作,可缓存定容集合数据至缓存,而不必确保每次查询MongoDB数据库,或者建立额外的索引保证读写速度。
- 默认建立_id索引;
限制和建议
更新
如果需要更新定容集合,则考虑要建立索引,以保证该更新操作不会扫库(collection scan);
如果更新或者替换操作改变了文档大小, 操作将会失败。
删除
不可以对定容集合中的文档进行删除,但可以通过drop()函数移除定容集合或者集合下所有文档。
分片
不可为定容集合分片;
Procedures
创建定容集合
通过db.createCollection()
命令创建定容集合,创建时需要指定集合最大空间大小(size),单位bytes:
db.createCollection( "log", { capped: true, size: 100000 } )
如果小于4096,则默认为4096 bytes。原文:If the size
field is less than or equal to 4096, then the collection will have a cap of 4096 bytes. Otherwise, MongoDB will raise the provided size to make it an integer multiple of 256。
此外还需要指定最大的文档数量(max),如:
db.createCollection("log", { capped : true, size : 5242880, max : 5000 } )
注意:size是必须指定的,当超过size大小时,即使没有超过最大文档数max,MongoDB也会自动移除最早的文档,为新插入文档提供可用空间。
定容集合查询
若通过find()查询时未指定排序字段,则按照存储顺序(insertion order)排序。如果想要按照存储顺序逆序排序,则需要指定自然排序$natural参数值为-1, 如:
db.cappedCollection.find().sort( { $natural: -1 } )
判断集合是否为定容集合
判断一个集合是否为定容集合,可通过isCapped()函数查询:
db.collection.isCapped()
普通集合转为定容集合
通过runCommand方法执行convertToCapped命令,将非定容集合转为定容集合:
db.runCommand({"convertToCapped": "mycoll", size: 100000});
该操作将会对集合加锁,其他需要加锁的操作将堵塞直到转换操作完成。
具体期限后自动移除文档
As an alternative to capped collections, consider MongoDB’s TTL (“time to live”) indexes. As described in Expire Data from Collections by Setting TTL, these indexes allow you to expire and remove data from normal collections based on the value of a date-typed field and a TTL value for the index.
TTL indexes are not compatible with capped collections.
Tailable Cursor
You can use a tailable cursor with capped collections. Similar to the Unix tail -f
command, the tailable cursor “tails” the end of a capped collection. As new documents are inserted into the capped collection, you can use the tailable cursor to continue retrieving documents.
以上为本人对官网手册的理解,若有疑问可直接参考官方手册: