搭建一个简单的RESTfull API接口项目
demo目录结构如下:
- spring-boot-starter-web的引入,其依赖包的学习
2.spring-boot-devtools的引入,其依赖包的学习
3.代码的实现
public class User {
private int id;
private String name;
private Date date;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
}
@RestController
@RequestMapping(value = "/index")
public class IndexController {
@RequestMapping
public String index() {
return "hello world";
}
// @RequestParam 简单类型的绑定,可以出来get和post
@RequestMapping(value = "/get")
public HashMap<String, Object> get(@RequestParam String name) {
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("title", "hello world");
map.put("name", name);
return map;
}
// @PathVariable 获得请求url中的动态参数
@RequestMapping(value = "/get/{id}/{name}")
public User getUser(@PathVariable int id, @PathVariable String name) {
User user = new User();
user.setId(id);
user.setName(name);
user.setDate(new Date());
return user;
}
}
4.运行项目
直接运行main方法或者使用maven命令: spring-boot:run
测试: http://localhost:8080/index
扫描二维码关注公众号,回复:
3246774 查看本文章
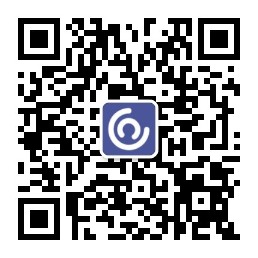
带参数:http://localhost:8080/index/get?name=china
带参数有中文:http://localhost:8080/index/get?name=哈罗
url测试:http://localhost:8080/index/get/1/wyt
5.打包
命令: clean package