插入:
扫描二维码关注公众号,回复:
3242200 查看本文章
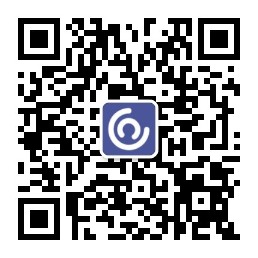
去掉字符串两端的空白:
DTString.h更新如下:
1 #ifndef DTSTRING_H 2 #define DTSTRING_H 3 4 #include "Object.h" 5 6 namespace DTLib 7 { 8 9 class String : Object 10 { 11 protected: 12 char* m_str; 13 int m_length; 14 15 void init(const char* s); 16 bool equal(const char* l, const char* r, int len) const; 17 public: 18 String(); 19 String(char c); 20 String(const char* s); 21 String(const String& s); 22 23 int length() const; 24 const char* str() const; 25 26 bool startWith(const char* s) const; 27 bool startWith(const String& s) const; 28 bool endOf(const char* s) const; 29 bool endOf(const String& s) const; 30 31 String& insert(int i, const char* s); 32 String& insert(int i, const String& s); 33 34 String& trim(); 35 36 char& operator [] (int i); 37 char operator [] (int i) const; 38 39 bool operator == (const String& s) const; 40 bool operator == (const char* s) const; 41 42 bool operator != (const String& s) const; 43 bool operator != (const char* s) const; 44 45 bool operator > (const String& s) const; 46 bool operator > (const char* s) const; 47 48 bool operator < (const String& s) const; 49 bool operator < (const char* s) const; 50 51 bool operator >= (const String& s) const; 52 bool operator >= (const char* s) const; 53 54 bool operator <= (const String& s) const; 55 bool operator <= (const char* s) const; 56 57 String operator + (const String& s) const; 58 String operator + (const char* s) const; 59 String operator += (const String& s); 60 String operator += (const char* s); 61 62 String& operator = (const String& s); 63 String& operator = (const char* s); 64 String& operator = (char c); 65 66 ~String(); 67 }; 68 69 } 70 71 72 #endif // DTSTRING_H
DTString.cpp更新如下:
1 #include <cstring> 2 #include <cstdlib> 3 #include "DTString.h" 4 #include "Exception.h" 5 6 using namespace std; 7 8 namespace DTLib 9 { 10 11 void String::init(const char* s) 12 { 13 m_str = strdup(s); 14 15 if( m_str ) 16 { 17 m_length = strlen(m_str); 18 } 19 else 20 { 21 THROW_EXCEPTION(NoEnoughMemoryException, "No memory to create string object ..."); 22 } 23 } 24 25 bool String::equal(const char* l, const char* r, int len) const 26 { 27 bool ret = true; 28 29 for(int i = 0; i < len && ret; i++) 30 { 31 ret = ret && (l[i] == r[i]); 32 } 33 34 return ret; 35 } 36 37 String::String() 38 { 39 init(""); 40 } 41 42 String::String(const char* s) 43 { 44 init(s ? s : ""); //空指针就转换成空字符串 45 } 46 47 String::String(const String& s) 48 { 49 init(s.m_str); 50 } 51 52 String::String(char c) 53 { 54 char s[] = {c, '\0'}; 55 56 init(s); 57 } 58 59 int String::length() const 60 { 61 return m_length; 62 } 63 64 const char* String::str() const 65 { 66 return m_str; 67 } 68 69 bool String::startWith(const char* s) const 70 { 71 bool ret = ( s != NULL ); 72 73 if( ret ) 74 { 75 int len = strlen(s); 76 77 ret = (len < m_length) && equal(m_str, s, len); 78 79 } 80 81 return ret; 82 } 83 84 bool String::startWith(const String& s) const 85 { 86 return startWith(s.m_str); 87 } 88 89 bool String::endOf(const char* s) const 90 { 91 bool ret = ( s != NULL ); 92 93 if( ret ) 94 { 95 int len = strlen(s); 96 97 char* str = m_str + (m_length - len); 98 99 ret = (len < m_length) && equal(str, s, len); 100 101 } 102 103 return ret; 104 } 105 106 bool String::endOf(const String& s) const 107 { 108 return endOf(s.m_str); 109 } 110 111 String& String::insert(int i, const char* s) 112 { 113 if( (0 <= i) && (i <= m_length) ) 114 { 115 if( ( s != NULL) && ( s[0] != '\0' ) ) 116 { 117 int len = strlen(s); 118 char* str = reinterpret_cast<char*>(malloc(m_length + len + 1)); 119 120 if( str != NULL ) 121 { 122 strncpy(str, m_str, i); 123 strncpy(str + i, s, len); 124 strncpy(str + i + len, m_str + i, m_length - i); 125 126 str[m_length + len] = '\0'; 127 128 free(m_str); 129 130 m_str = str; 131 m_length = m_length + len; 132 } 133 else 134 { 135 THROW_EXCEPTION(NoEnoughMemoryException, "No memory to create str object..."); 136 } 137 } 138 } 139 else 140 { 141 THROW_EXCEPTION(IndexOutOfBoundsException, "parameter i is invalid..."); 142 } 143 144 return *this; 145 } 146 147 String& String::insert(int i, const String& s) 148 { 149 return insert(i, s.m_str); 150 } 151 152 String& String::trim() 153 { 154 int b = 0; 155 int e = m_length - 1; 156 157 while( m_str[b] == ' ')b++; 158 while( m_str[e] == ' ')e--; 159 160 if( b == 0 ) 161 { 162 m_str[e + 1] = '\0'; 163 164 m_length = e + 1; 165 } 166 else 167 { 168 for(int i = 0,j = b; j <= e; i++, j++) 169 { 170 m_str[i] = m_str[j]; 171 } 172 173 m_str[e - b + 1] = '\0'; 174 m_length = e - b + 1; 175 } 176 177 return *this; 178 } 179 180 char& String::operator [] (int i) 181 { 182 if( (0 <= i) && (i < m_length) ) 183 { 184 return m_str[i]; 185 } 186 else 187 { 188 THROW_EXCEPTION(IndexOutOfBoundsException, "parameter i is invalid ..."); 189 } 190 } 191 192 char String::operator [] (int i) const 193 { 194 return (const_cast<String&>(*this))[i]; 195 } 196 197 bool String::operator == (const String& s) const 198 { 199 return ( strcmp(m_str, s.m_str) == 0 ); 200 } 201 202 bool String::operator == (const char* s) const 203 { 204 return ( strcmp(m_str, s ? s : "") == 0 ); 205 } 206 207 bool String::operator != (const String& s) const 208 { 209 return !(*this == s); 210 } 211 212 bool String::operator != (const char* s) const 213 { 214 return !(*this == s); 215 } 216 217 bool String::operator > (const String& s) const 218 { 219 return (strcmp(m_str, s.m_str) > 0); 220 } 221 222 bool String::operator > (const char* s) const 223 { 224 return (strcmp(m_str, s ? s : "") > 0); 225 } 226 227 bool String::operator < (const String& s) const 228 { 229 return (strcmp(m_str, s.m_str) < 0); 230 } 231 232 bool String::operator < (const char* s) const 233 { 234 return (strcmp(m_str, s ? s : "") < 0); 235 } 236 237 bool String::operator >= (const String& s) const 238 { 239 return (strcmp(m_str, s.m_str) >= 0); 240 } 241 242 bool String::operator >= (const char* s) const 243 { 244 return (strcmp(m_str, s ? s : "") >= 0); 245 } 246 247 bool String::operator <= (const String& s) const 248 { 249 return (strcmp(m_str, s.m_str) <= 0); 250 } 251 252 bool String::operator <= (const char* s) const 253 { 254 return (strcmp(m_str, s ? s : "") <= 0); 255 } 256 257 String String::operator + (const String& s) const 258 { 259 return (*this + s.m_str); 260 } 261 262 String String::operator + (const char* s) const 263 { 264 String ret; 265 266 int len = m_length + strlen(s ? s : ""); 267 268 char* str = reinterpret_cast<char*>(malloc(len + 1)); 269 270 if( str ) 271 { 272 strcpy(str, m_str); 273 strcat(str, s ? s : ""); 274 275 free(ret.m_str); 276 277 ret.m_str = str; 278 ret.m_length = len; 279 } 280 else 281 { 282 THROW_EXCEPTION(NoEnoughMemoryException, "No memory to create str object..."); 283 } 284 285 return ret; 286 } 287 String String::operator += (const String& s) 288 { 289 return (*this = *this + s.m_str); 290 } 291 292 String String::operator += (const char* s) 293 { 294 return (*this = *this + s); 295 } 296 297 String& String::operator = (const String& s) 298 { 299 return (*this = s.m_str); 300 } 301 302 String& String::operator = (const char* s) 303 { 304 if( m_str != s ) 305 { 306 char* str = strdup(s ? s: ""); 307 308 if( str ) 309 { 310 free(m_str); 311 312 m_str = str; 313 m_length = strlen(m_str); 314 } 315 else 316 { 317 THROW_EXCEPTION(NoEnoughMemoryException, "No memory to create str object..."); 318 } 319 } 320 321 return *this; 322 } 323 324 String& String::operator = (char c) 325 { 326 char s[] = {c, '\0'}; 327 328 return (*this = s); 329 } 330 331 String::~String() 332 { 333 free(m_str); 334 } 335 336 }
测试程序如下:
1 #include <iostream> 2 #include "DTString.h" 3 4 using namespace std; 5 using namespace DTLib; 6 7 8 9 int main() 10 { 11 String s = "D.T.Software"; 12 13 cout << s.startWith("D.T.") << endl; 14 cout << s.endOf("Software") << endl; 15 16 for(int i = 0; i < s.length(); i++) 17 { 18 cout << s[i] << endl; 19 } 20 21 String s1 = ""; 22 s1.insert(0, "D.T."); 23 s1.insert(4, "Software"); 24 25 cout << s1.str() << endl; 26 27 String s2 = " abc "; 28 29 if( s2.trim().insert(0, "D.T.").endOf("abc") && s2.startWith("D.T.") ) 30 { 31 cout << s2.str() << endl; 32 } 33 34 35 return 0; 36 }
运行结果如下: