The problem:
Given an array of 2n integers, your task is to group these integers into n pairs of integer, say (a1, b1), (a2, b2), ..., (an, bn) which makes sum of min(ai, bi) for all i from 1 to n as large as possible.
Example 1:
Input: [1,4,3,2]
Output: 4
Explanation: n is 2, and the maximum sum of pairs is 4 = min(1, 2) + min(3, 4).
Note:
- n is a positive integer, which is in the range of [1, 10000].
- All the integers in the array will be in the range of [-10000, 10000].
My method: I use the quickSort() when the nums are huge, and insertSort() when the numsSize are small. And make some optimizations
void swap(int *nums, int i, int j) {
int tmp;
tmp = nums[i];
nums[i] = nums[j];
nums[j] = tmp;
}
int find_prov(int *nums, int low, int high) {
int m = low + (high - low)/2; //Try to find a middle value from {nums[low], nums[m], nums[high]}
int key;
if (nums[low] > nums[high]) {
swap(nums, low, high);
}
if (nums[high] < nums[m]) {
swap(nums, high, m);
}
if (nums[m] > nums[low]) {
swap(nums, m, low);
}
key = nums[low]; //keep nums[low] is the middle value.
while (low < high) {
while (low < high && nums[high] >= key) {
high--;
}
if (low < high) {
nums[low++] = nums[high];
}
while (low < high && nums[low] <= key) {
low++;
}
if (low < high) {
nums[high--] = nums[low];
}
}
nums[low] = key;
return low;
}
void quickSort(int* nums, int low, int high) {
int prov;
if (low < high) {
prov = find_prov(nums, low, high);
quickSort(nums, low, prov - 1);
quickSort(nums, prov + 1, high);
}
}
void insertSort(int* nums, int len) {
int i, j;
int key;
for (i = 1; i < len; i++) {
key = nums[i];
for (j = i - 1; j >= 0 && (nums[j] >= key); j--) {
nums[j + 1] = nums[j];
}
nums[j + 1] = key;
}
}
int arrayPairSum(int* nums, int numsSize) {
int i, j, k;
int sum = 0;
if (numsSize < 1 || numsSize > 20000) {
printf("error, pls ensure numsSize in the range of [1, 10000].\n");
return 0;
}
int arr1[20000];
int arr2[20000];
for (i = 0, j = 0, k = 0; i < numsSize; i++) {//Split two parts: negative and non-negative
if (nums[i] < 0) {
arr1[j++] = nums[i];
} else {
arr2[k++] = nums[i];
}
}
if (numsSize > 9) { //For large arr, use quickSort; instead use insertSort
quickSort(arr1, 0, j - 1);
quickSort(arr2, 0, k - 1);
} else {
insertSort(arr1, j);
insertSort(arr2, k);
}
for (i = 0; i < j; i+=2) {
sum += arr1[i];
}
for (i = k - 2; i >= 0; i-=2) {
sum += arr2[i];
}
return sum;
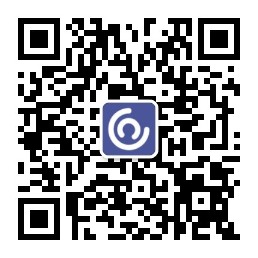
}
Test results:
Submission Detail
81 / 81 test cases passed. |
Status: Accepted |
Runtime: 28 ms |