Tasks:
1. Install Junit(4.12),Hamcrest(1.3) with Eclipse
2. Install Eclemmawith Eclipse
3. Write a javaprogram for the triangle problem and test the program with Junit.
a) Description oftriangle problem:
Functiontriangle takes three integers a,b,cwhich are length of triangle sides; calculates whether the triangle isequilateral, isosceles, or scalene.
Requirements for theexperiment:
1. Finish the tasksabove individually.
2. Check in yourjava code and junit test program to github and send the URL to [email protected]
3. Post yourexperiment report to your blog and send the URL to [email protected] , the following information should beincluded in your report:
a) The brief descriptionthat you install junit, hamcrest and eclemma.
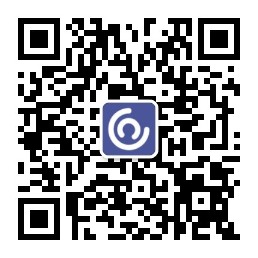
b) The test resultand coverage report (print screen) of your tests on triangle problem.
一、使用eclips,配置Junit4.12,hamcrest-all-1.3
下载这两个jar包,在src文件目录下新建文件夹lib,将两个jar包复制进去。打开eclips项目,右键分别点击两个jar包,选择build path.
二、安装Eclemmawith插件
下载Eclemmawith-2.3.3,在eclipse中Help-中选择install new software,选择压缩包即可安装成功,重新启动eclipse,会出现最左面的图标
三、编写Triangle()函数
package lab1.tr;
public class Triangle {
public String triangle(int a,int b,int c)
{
if(a==b && b==c)
return "Equilateral triangle";
else if(a==b || b==c || a==c)
return "Isosceles triangle";
else if(a+b<c || a+c<b || b+c<a)
return "Not triangle";
return "Scalene triangle";
}
}
四、新建测试类TestTriangle.java,编写Junit代码
先逐一测试了各个输出。
最后使用了Parameter的参数化测试,将所有测试用例放到了一起。
package lab1.tr;
import static org.junit.Assert.*;
import java.util.Collection;
import java.util.Arrays;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import org.junit.runners.Parameterized.Parameters;
@RunWith(Parameterized.class)
public class TestTriangle {
private Triangle tri;
private int a,b,c;
private String s;
public TestTriangle(int a,int b,int c,String s){
this.a=a;
this.b=b;
this.c=c;
this.s=s;
}
@Before
public void setup(){
tri=new Triangle();
}
@Test
public void testEquilateral(){
assertEquals("Equilateral triangle",tri.triangle(3,3,3));
}
@Test
public void testIsosceles(){
assertEquals("Isosceles triangle",tri.triangle(3,3,4));
}
@Test
public void testScalene(){
assertEquals("Scalene triangle",tri.triangle(3,4,5));
}
@Test
public void testNotTriangle(){
assertEquals("Not triangle",tri.triangle(3, 4, 8));
}
//参数化测试
@Parameters
public static Collection<Object[]> getResult(){
return Arrays.asList(new Object[][]{
{3,3,3,"Equilateral triangle"},
{3,3,4,"Isosceles triangle"},
{3,4,5,"Scalene triangle"},
{3,4,8,"Not triangle"}
});
}
@Test
public void testTriangle(){
assertEquals(this.s,tri.triangle(a, b, c));
}
}
五、覆盖结果