1 .snprintf()
函数原型如下:
int _snprintf(char *buffer, size_t count,const char *format [,argument] ... );
用于将格式化的数据写入字符串,
If len < count, then len characters are stored in buffer, a null-terminator is appended, and len is returned.
If len = count, then len characters are stored in buffer, no null-terminator is appended, and len is returned.
If len > count, then count characters are stored in buffer, no null-terminator is appended, and a negative value is returned.
for example:char sa[256];
sa[sizeof(sa)-1]=0;
snprintf(sa,sizeof(sa),"%s",sb);
if(sa[sizeof(sa)-1]!=0)
{
printf("warning:string will be truncated");
sa[sizeof(sa)-1]=0;
}
2. fwrite()
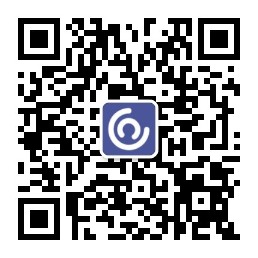
函数原型如下:
size_t fwrite(const void *buffer, size_t size, size_t count , FILE *stream)
buffer: 指向被写入的数据源指针
size: 每次写入的字节数大小
count : 执行一次fwrite函数,最大可写入 count个size大小的字节数
stream 指向等待写入数据的文件指针,即文件被写入的地址
如果写入成功则返回count值。
fread()
函数原型如下:
size_t fread(void *buffer, size_t size, size_t count, FILE *stream);
buffer : 数据存储的地址
size : 要读取的字节的大小
count : 要读取多少个size大小
stream : 等待被读取的数据源,它是一个指向FILE结构的文件指针
fread 如果读取成功,则返回count的大小,如果还没有读取count个size大小的数据时,就以及读取完了整个文件,到了文件结尾的地方了,此时返回的值就要必count小,或者在读取的过程出错的话,返回的值也必count小,所以如果返回的值比count小时,可以通过feof()函数或ferror函数来判断到底是读取过程中出错了还是已经读取到了文件结尾的部分了。 如果size 或count 本身被设置为0, 则fread不做任何操作。
注:fread 函数的功能是从输入流(input stream)中读取count个size大小的字节数存储到buffer中,关联输入流的文件指针的位置会随着字节数的读取而向前移动。如果输入流是以文本模式打开的,那么单个换行符将会被替换成回车换行符,换行符的替换,不会影响到文件指针和返回值。如果遇到了错误,那么文件指针的当前位置和已读取的不部分值都是不确定的。
void main( void )
{
FILE *stream;
char list[30];
int i, numread, numwritten;
/* Open file in text mode: */
if( (stream = fopen( "fread.out", "w+t" )) != NULL )
{
for ( i = 0; i < 25; i++ )
list[i] = (char)('z' - i);
/* Write 25 characters to stream */
numwritten = fwrite( list, sizeof( char ), 25, stream );
printf( "Wrote %d items\n", numwritten );
fclose( stream );
}
else
printf( "Problem opening the file\n" );
if( (stream = fopen( "fread.out", "r+t" )) != NULL )
{
/* Attempt to read in 25 characters */
numread = fread( list, sizeof( char ), 25, stream );
printf( "Number of items read = %d\n", numread );
printf( "Contents of buffer = %.25s\n", list );
fclose( stream );
}
else
printf( "File could not be opened\n" );
}
3. fprintf()
函数原型如下:
int fprintf(FILE *stream, const char *format, ...)
fprintf()会根据参数format 字符串来转换并格式化数据, 然后将结果输出到参数stream 指定的文件中, 直到出现字符串结束('\0')为止。
成功返回实际输出的字符数,失败返回-1
4. fseek()
int fseek(FILE * stream, long offset, int whence);
参数stream 为已打开的文件指针,
参数offset 为根据参数whence 来移动读写位置的位移数。参数 whence 为下列其中一种:
SEEK_SET 从距文件开头offset 位移量为新的读写位置. SEEK_CUR 以目前的读写位置往后增加offset 个位移量.
SEEK_END 将读写位置指向文件尾后再增加offset 个位移量. 当whence 值为SEEK_CUR 或
SEEK_END 时, 参数offset 允许负值的出现.
for example:
1) 欲将读写位置移动到文件开头时:fseek(FILE *stream, 0, SEEK_SET);
2) 欲将读写位置移动到文件尾时:fseek(FILE *stream, 0, 0SEEK_END);
返回值:当调用成功时则返回0, 若有错误则返回-1, errno 会存放错误代码.
附加说明:fseek()不像lseek()会返回读写位置, 因此必须使用ftell()来取得目前读写的位置.
FILE * stream;
long offset;
fpos_t pos;
stream = fopen("/etc/passwd", "r");
fseek(stream, 5, SEEK_SET);
printf("offset = %d\n", ftell(stream));
rewind(stream);
fgetpos(stream, &pos);
printf("offset = %d\n", pos); // windows 下的情况
pos = 10;
fsetpos(stream, &pos);
printf("offset = %d\n", ftell(stream));
fclose(stream);
}
执行
offset = 5
offset = 0
offset = 10
在linux下,fpos_t是一个结构体,定义为:
typedefstruct
{
__off_t __pos;// 双下划线
__mbstate_t __state;
}fpos_t;
fpos_t.__pos才是指向当前位置的整数,可以进行赋值,如 fpos_t.__pos = 12。
在linux下,不能这样定义pos:
fpos_t * pos;
fgetpos(fp,pos);
必须这样定义:
fpos_t pos;
fgetpos(fp,&pos);
因为pos必须是一个分配好内存空间的参数。第一种方式仅仅是定义了一个指针,并没有分配空间。如果改为下面的定义方式,则可以编译通过:
fpos_t * pos;
pos = (fpos_t)malloc(12);
fgetpos(fp,pos);
rewind()函数用于将文件指针重新指向文件的开头,同时清除和文件流相关的错误和eof标记,相当于调用fseek(stream, 0, SEEK_SET),其原型如下:
void rewind(FILE * stream);
文件指针FILE *stream中,包含一个读写位置指针char *_nextc,它指向下一次文件读写的位置。其结构如下:
typedefstruct
{
int _fd;// 文件号
int _cleft;// 缓冲区中剩下的字节数
int _mode;// 文件操作模式
char* _nextc;// 下一个字节的位置
char* _buff;// 文件缓冲区位置
}FILE;
每当进行一次读写后,该指针自动指向下一次读写的位置。当文件刚打开或创建时,该指针指向文件的开始位置。可以用函数ftell()获得当前的位置指针,也可以用rewind()/fseek()函数改变位置指针,使其指向需要读写的位置。
ftell()用来获取文件的当前读写位置
函数原型: long ftell(FILE *fp)
函数功能:得到流式文件的当前读写位置,其返回值是当前读写位置偏离文件头部的字节数.