目录
3.1创建applicationContext-solr.xml
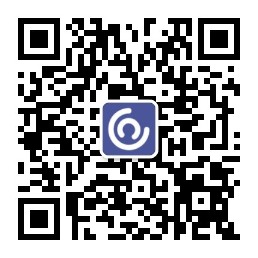
4.2添加taotao-search-interface的依赖
1.功能分析
在schema.xml中定义以下业务域(已经定义好):
商品Id
商品标题
商品卖点
商品价格
商品图片
分类名称
商品描述
需要从tb_item, tb_item_cat, tb_item_desc表中查询status=1的数据(商品状态,1-正常,2-下架,3-删除)。
SELECT
a.id,
a.title,
a.sell_point,
a.price,
a.image,
b. NAME category_name,
c.item_desc
FROM
tb_item a,
tb_item_cat b,
tb_item_desc c
WHERE
a.cid = b.id
AND a.id = c.item_id
AND a.`status` = 1;
在taotao-manager-web后台系统中 做一个导入索引库的功能界面(例如:有个按钮,点击即可将数据从数据库中导入到索引库)。
比如:管理员点击 “一键导入商品数据到索引库”,后端从数据库中读取商品数据,导入solr索引库。
solr索引库中存在商品数据
业务逻辑:
1.点击按钮,表现层调用服务层的工程的导入索引库的方法
2.服务层实现 调用mapper接口的方法查询所有的商品的数据
3.将数据一条条添加到solrinputdocument文档中
4.将文档添加到索引库中
5.提交,并返回导入成功即可
2.dao层
2.1创建pojo
我们查看从tb_item, tb_item_cat, tb_item_desc表中查询status=1的数据。
针对来自三张表的数据,我们需要使用一个pojo来接收这些数据,而且这个pojo还会作为查询结果的载体,因此服务层和表现层都会用到这个pojo,我们最好把它放到taotao-common工程的pojo目录下。我们新建SearchItem类(记得要实现序列化,因为要进行网络传输)
package com.taotao.common.pojo;
import java.io.Serializable;
/**
* 搜索的商品数据POJO
*/
public class SearchItem implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
private Long id;//商品的id
private String title;//商品标题
private String sell_point;//商品卖点
private Long price;//价格
private String image;//商品图片的路径
private String category_name;//商品分类名称
private String item_desc;//商品的描述
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getSell_point() {
return sell_point;
}
public void setSell_point(String sell_point) {
this.sell_point = sell_point;
}
public Long getPrice() {
return price;
}
public void setPrice(Long price) {
this.price = price;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
public String getCategory_name() {
return category_name;
}
public void setCategory_name(String category_name) {
this.category_name = category_name;
}
public String getItem_desc() {
return item_desc;
}
public void setItem_desc(String item_desc) {
this.item_desc = item_desc;
}
}
2.2创建mapper接口
由于设置及到tb_item, tb_item_cat, tb_item_desc三张表,mybatis的逆向工程就不能用了,我们需要自己编写mapper接口和mapper映射文件。由于搜索商品功能只有taotao-search工程才会用到,所以我们可以直接将mapper接口和mapper映射文件放到taotao-search-servcie。
先编写mapper接口
在taotao-search-service工程下新建一个"com.taotao.search.mapper"包,并在该包下新建SearchMapper接口类,在接口中添加一个接口getSearchItemList
package com.taotao.search.mapper;
import java.util.List;
import com.taotao.common.pojo.SearchItem;
public interface SearchItemMapper {
/**
* 查询所有SearchItem
* @return
*/
public List<SearchItem> getSearchItemList();
}
2.3创建Mapper映射文件
要使用mybatis的mapper接口,就需要编写mapper.xml映射文件
将mapper.xml放到com.taotao.search.mapper中
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.taotao.search.mapper.SearchItemMapper" >
<select id="getSearchItemList" resultType="com.taotao.common.pojo.SearchItem">
SELECT
a.id,
a.title,
a.sell_point,
a.price,
a.image,
b.NAME category_name,
c.item_desc
FROM
tb_item a,
tb_item_cat b,
tb_item_desc c
WHERE a.cid = b.id
AND a.id = c.item_id
AND a.status = 1
</select>
</mapper>
由于maven发布代码和配置文件时,在src/main/java下,maven只会将*.java文件编译成*.class文件发布到classpath下,对于*.xml文件等,maven是不会理会的,所以我们需要配置maven扫描src/main/java下的*.xml文件。但是又不能影响maven扫描src/main/resources下面的*.xml、*.properties文件,所以我们需要在taotao-search-service中这样配置,如图所示:
<!-- 如果不添加此节点mybatis的mapper.xml文件都会被漏掉。 -->
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<!-- 如果没有此节点,src/main/resources目录下的配置文件将被忽略 -->
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
3.service层
参数:无
业务逻辑:taotao-search中实现
1、查询所有商品数据。
2、创建一个SolrServer对象。(solrServer对象交给spring管理)
3、为每个商品创建一个SolrInputDocument对象。
4、为文档添加域
5、向索引库中添加文档。
6、返回TaotaoResult。
3.1创建applicationContext-solr.xml
由于我们将solrServer对象交给spring管理,所以需要创建applicationContext-solr.xml专门管理关于solr的对象
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context" xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.2.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.2.xsd">
<bean id="httpSolrServer" class="org.apache.solr.client.solrj.impl.HttpSolrServer">
<constructor-arg name="baseURL"
value="http://192.168.25.154:8080/solr" />
</bean>
</beans>
3.2创建service接口
在taotao-search-interface中创建“com.taotao.search.service”包,在里面创建SearchService接口用于实现搜索相关的业务接口。
package com.taotao.search.service;
import com.taotao.common.pojo.TaotaoResult;
/**
* 搜索业务逻辑
* @author Administrator
*
*/
public interface SearchService {
/**
* 导入所有searchItem到solr索引库中
* @return
* @throws Exception
*/
TaotaoResult importAllItems() throws Exception;
}
3.3service实现类
由于要使用mybatis访问数据库、导入索引库等,所有要导入mybatis依赖、solrj等
<!-- Mybatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
</dependency>
<!-- 分页插件pagehelper -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
</dependency>
<!-- MySql -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- 连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
</dependency>
<!-- solr客户端 -->
<dependency>
<groupId>org.apache.solr</groupId>
<artifactId>solr-solrj</artifactId>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
</dependency>
在taotao-search-service的“com.taotao.search.service.impl”中编写实现类,查询所有searchItem,导入索引库
要注意注入mapper接口和solrServer的实现类
package com.taotao.search.service.impl;
import java.util.List;
import org.apache.solr.client.solrj.SolrServer;
import org.apache.solr.common.SolrInputDocument;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.taotao.common.pojo.SearchItem;
import com.taotao.common.pojo.TaotaoResult;
import com.taotao.search.service.SearchService;
import com.taotao.search.mapper.SearchItemMapper;
@Service
public class SearchServiceImpl implements SearchService {
@Autowired
private SearchItemMapper SearchItemMapper;
@Autowired
private SolrServer solrServer;
/**
* 导入所有searchItem到solr索引库中
* @return
* @throws Exception
*/
@Override
public TaotaoResult importAllItems() throws Exception {
//1.注入mapper,发布服务
//2.调用mapper,查询所有商品数据
List<SearchItem> searchItemList = SearchItemMapper.getSearchItemList();
//3.注入solrServer对象
//4.为每个商品创建一个solrInputDocument
for (SearchItem searchItem : searchItemList) {
SolrInputDocument document = new SolrInputDocument();
document.addField("id", searchItem.getId());
document.addField("item_title", searchItem.getTitle());
document.addField("item_sell_point", searchItem.getSell_point());
document.addField("item_price", searchItem.getPrice());
document.addField("item_image", searchItem.getImage());
document.addField("item_category_name", searchItem.getCategory_name());
document.addField("item_desc", searchItem.getItem_desc());
//5.向索引库添加文档
solrServer.add(document);
}
//6.日记修改
solrServer.commit();
//7.返回结果
return TaotaoResult.ok();
}
}
3.4在taotao-search-service发布服务
要注意duubo暴露的端口不能与之前服务端口冲突
<dubbo:service interface="com.taotao.search.service.SearchService" ref="searchServiceImpl" />
4.表现层
要注意我们在管理后台导入的索引,所以在taotao-manager-web编写controller与导入页面
4.1引入服务
在taotao-manager-web引入taotao-search-service暴露的服务接口
<dubbo:reference interface="com.taotao.search.service.SearchService" id="searchService" timeout="300000" />
4.2添加taotao-search-interface的依赖
在taotao-manager-web工程中的pom.xml中添加如下:
<dependency>
<groupId>com.taotao</groupId>
<artifactId>taotao-search-interface</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
4.3编写controller
创建IndexManagerController,索引管理,注入service实现类,拦截的url可以自定义
package com.taotao.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.taotao.common.pojo.TaotaoResult;
import com.taotao.search.service.SearchService;
@Controller
public class IndexManagerController {
@Autowired
private SearchService searchService;
@RequestMapping("/index/importAll")
@ResponseBody
public TaotaoResult importAllSearchItem() {
try {
TaotaoResult result = searchService.importAllItems();
return result;
} catch (Exception e) {
e.printStackTrace();
return TaotaoResult.build(500, "导入数据失败");
}
}
}
4.4创建jsp
在taotao-manager-web中创建一个SearchIndexManager.jsp,并编写导入索引按钮。url要对应searchController拦截的url
如下图:
中间做了一点效果,用户点击时按钮不可点击,导入索引库成功后还原按钮状态
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<div>
<a href="javascript:void(0)" class="easyui-linkbutton" onclick="importAll()">一键导入商品数据到索引库</a>
</div>
<script type="text/javascript">
function importAll() {
//移除原有按钮
$('.l-btn-text').remove("span");
//追加不可点击按钮
$('.l-btn-left').after('<input type="button" disabled="true" id="disabledButton" value="正在导入商品搜索索引库" >');
$.ajax({
url:"/index/importAll",
type: "GET",
dataType: "json",
success:function(data){
//移除不可点击按钮
$('#disabledButton').remove("input");
//追加原有按钮
$('.l-btn-left').after('<span class="l-btn-text">一键导入商品数据到索引库</span>');
if (data.status==200) {
$.messager.alert('提示','商品数据导入成功!');
} else {
$.messager.alert('提示','商品数据导入失败!');
}
}
});
}
</script>
4.5修改index.jsp
url要对应
<li>
<span>网站索引管理</span>
<ul>
<li data-options="attributes:{'url':'SearchIndexManager'}">导入索引库</li>
</ul>
</li>
然后安装修改的工程、启动服务层与表现层即可。