题目大意[1]:给定一个字符串列表,每个字符串都由26个小写英文字母组成,每个字串长度都在[1,12],列表长度不超过100。26个小写字母从a到z分别映射到
[".-","-...","-.-.","-..",".","..-.","--.","....","..",".---","-.-",".-..","--","-.","---",".--.","--.-",".-.","...","-","..-","...-",".--","-..-","-.--","--.."]
求映射之后得到的字符串列表去重后的长度。
for example:给定一个字符串列表,如["gin", "zen", "gig", "msg"]按上述变换成为
"gin" -> "--...-."
"zen" -> "--...-."
"gig" -> "--...--."
"msg" -> "--...--."
去重后为"--...-." and "--...--.",即长度为2。
很简单,直接上代码:
class Solution:
def uniqueMorseRepresentations(self, words):
"""
:type words: List[str]
:rtype: int
"""
dt = {'a':".-","b":"-...","c":"-.-.","d":"-..","e":".","f":"..-.","g":"--.","h":"....","i":"..","j":".---","k":"-.-","l":".-..","m":"--","n":"-.","o":"---","p":".--.","q":"--.-","r":".-.","s":"...","t":"-","u":"..-","v":"...-","w":".--","x":"-..-","y":"-.--","z":"--.."}
if len(words)>180 or len(words)<=0:
return 0
else:
value = ""
length =[]
for i in range(0,len(words)):
for j in range(0,len(words[i])):
v = dt.get(words[i][j])
value += v
length.append(value)
value = ""
return len(set(length))
然而,发现了一个更pythonic的写法[2]:
def uniqueMorseRepresentations(self, words):
d = [".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..", "--",
"-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--.."]
return len({''.join(d[ord(i) - ord('a')] for i in w) for w in words})
有人会问,ord()是啥,别急,我们瞅瞅看。看python文档里说[3]:
ord
(c)
Given a string of length one, return an integer representing the Unicode code point of the character when the argument is a unicode object, or the value of the byte when the argument is an 8-bit string. For example, ord('a')
returns the integer 97
, ord(u'\u2020')
returns 8224
. This is the inverse of chr()
for 8-bit strings and of unichr()
for unicode objects. If a unicode argument is given and Python was built with UCS2 Unicode, then the character’s code point must be in the range [0..65535] inclusive; otherwise the string length is two, and a TypeError
will be raised.
翻译成人话就是把字母转化成对应ASCII码。
Ref:
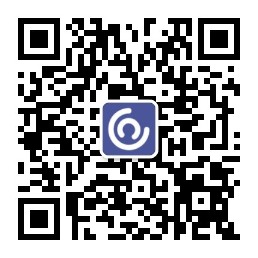
[1] https://leetcode.com/problems/unique-morse-code-words/description/