1、String:字符串常量是引用类型
StringBuilder:字符串变量(非线程安全的)
StringBuffer:字符串变量(线程安全)
2、String常用的方法及作用
//常用
String s = "hello";
System.out.println("s:"+s);
//方式1
//String(String original):把字符串数据封装成字符串对象
String s = new String("hello");
System.out.println("s:"+s);
String s1 = new String("hello");
String s2 = "hello";
String s3 = "hello";
System.out.println("s1==s2:"+(s1==s2)); //false
System.out.println("s1==s3:"+(s1==s3)); //false
System.out.println("s2==s3:"+(s2==s3)); //true
//方式2
//String(char[] value):把字符数组的数据封装成字符串对象
char[] chs = {'h','e','l','l','o'};
String s = new String(chs);
System.out.println("s:"+s);
//方式3
//String(char[] value, int index, int count):把字符数组中的一部分数据封装成字符串对象
//String s3 = new String(chs,0,chs.length);
char[] chs = {'h','e','l','l','o'};
String s = new String(chs,1,3);
System.out.println("s:"+s);
//创建字符串对象
String s1 = "hello";
String s2 = "hello";
String s3 = "Hello";
//boolean equals(Object obj):比较字符串的内容是否相同
System.out.println(s1.equals(s2));//true
System.out.println(s1.equals(s3));//false
public boolean equalsIgnoreCase(String another) 比较字符串与another是否一样(忽略大小写);
//boolean equalsIgnoreCase(String str):比较字符串的内容是否相同,忽略大小写
System.out.println(sa1.equalsIgnoreCase(sa2));//true
System.out.println(sa1.equalsIgnoreCase(sa3));//true
public boolean endsWith(String suffix) 判断一个字符串是否以suffix字符串结尾;
String s="hello";
//boolean endsWith(String str):判断字符串对象是否以指定的str结尾
System.out.println(s.endsWith("lo"));//true
System.out.println(s.endsWith("ll"));//false
public boolean startsWith(String prefix) 判断字符串是否以prefix字符串开头;
//boolean startsWith(String str):判断字符串对象是否以指定的str开头
System.out.println(sa1.startsWith("he"));//true
System.out.println(sa1.startsWith("ll"));//false
//创建字符串对象
String s = "helloworld";
public int length() 返回字符串的长度;
//int length():获取字符串的长度,其实也就是字符个数
System.out.println(s.length());
public char charAt(int index) 返回字符串中第index个字符;
//char charAt(int index):获取指定索引处的字符
System.out.println(s.charAt(0)); //h
System.out.println(s.charAt(1)); //e
public int indexOf(String str) 返回字符串中第一次出现str的位置;
//int indexOf(String str):获取str在字符串对象中第一次出现的索引
System.out.println(s.indexOf("l"));//2
System.out.println(s.indexOf("owo"));//4
System.out.println(s.indexOf("ak"));//-1
public int indexOf(String str,int fromIndex) 返回字符串从fromIndex开始第一次出现str的位置;
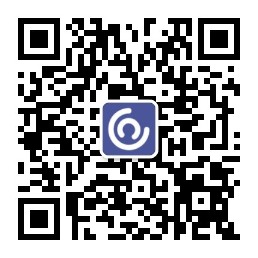
//创建字符串对象
String s = "helloworld";
//截取首字母
System.out.println(s.indexOf("l",4));
public String substring(int beginIndex) 返回该字符串从beginIndex开始到结尾的子字符串;
//String substring(int start):从start开始截取字符串
System.out.println(s.substring(0));//helloworld
System.out.println(s.substring(5));//world
public String substring(int beginIndex,int endIndex) 返回该字符串从beginIndex开始到endsIndex结尾的子字符串
//String substring(int start,int end):从start开始,到end结束截取字符串
System.out.println(s.substring(0, s.length()));//helloworld
System.out.println(s.substring(3,8));//lowor
//创建字符串对象
String s = "helloworld";
//截取首字母
String s1 = s.substring(0, 1);
System.out.println(s1);//h
public String replace(char oldchar,char newChar) 在字符串中用newChar字符替换oldChar字符
//创建字符串对象
String s = "helloworld";
String s1 = s.replace('e', 'a');
System.out.println(s1);//halloworld
public String toUpperCase() 返回一个字符串为该字符串的大写形式;
//String toUpperCase():把字符串转换为大写字符串
System.out.println("HelloWorld".toUpperCase());//HELLOWORLD
public String toLowerCase() 返回一个字符串为该字符串的小写形式
//String toLowerCase():把字符串转换为小写字符串
System.out.println("HelloWorld".toLowerCase());//helloworld
public String trim() 返回该字符串去掉开头和结尾空格后的字符串
//创建字符串对象
String s1 = "helloworld";
String s2 = " helloworld ";
String s3 = " hello world ";
System.out.println("---"+s1.trim()+"---");//---helloworld---
System.out.println("---"+s2.trim()+"---");//---helloworld---
System.out.println("---"+s3.trim()+"---");//---hello world---
public String[] split(String regex) 将一个字符串按照指定的分隔符分隔,返回分隔后的字符串数组
//String[] split(String str)
//创建字符串对象
String s = "aa,bb,cc";
String[] strArray = s.split(",");
for(int i=0; i<strArray.length; i++) {
System.out.println(strArray[i]);
}
3、StringBuilder的最常用法
StringBuilder sb = new StringBuilder();
System.out.println("sb:"+sb);
System.out.println("sb.capacity():"+sb.capacity());//16
System.out.println("sb.length():"+sb.length());//0
//创建对象
StringBuilder sb = new StringBuilder();
//public StringBuilder append(任意类型)
StringBuilder sb2 = sb.append("hello");
System.out.println("sb:"+sb);
System.out.println("sb2:"+sb2);
System.out.println(sb == sb2); //true
//链式编程
sb.append("hello").append("world").append(true).append(100); //helloworldtrue100
System.out.println("sb:"+sb);
//public StringBuilder reverse() //值倒序排列
sb.reverse();
System.out.println("sb:"+sb);//001eurtdlrowolleh
4、 StringBuffer的最常用法
StringBuffer sb = new StringBuffer();
System.out.println("sb:"+sb);
System.out.println("sb.capacity():"+sb.capacity());//16
System.out.println("sb.length():"+sb.length());//0
//创建对象
StringBuffer sb = new StringBuffer();
//public StringBuffer append(任意类型)
StringBuffer sb2 = sb.append("hello");
System.out.println("sb:"+sb);
System.out.println("sb2:"+sb2);
System.out.println(sb == sb2); //true
//链式编程
sb.append("hello").append("world").append(true).append(100); //helloworldtrue100
System.out.println("sb:"+sb);
//public StringBuffer reverse() //值倒序排列
sb.reverse();
System.out.println("sb:"+sb);//001eurtdlrowolleh
//public StringBuffer deleteCharAt(int index)删除指定位置的字符,然后将剩余的内容形成新的字符串
StringBuffer sb = new StringBuffer("helloworld");
sb. deleteCharAt(1);
System.out.println(sb);//hlloworld
//public StringBuffer insert(int offset, boolean b)在StringBuffer对象中插入内容,然后形成新的字符串。
StringBuffer sb = new StringBuffer("helloworld");
sb.insert(4,false);
System.out.println(sb);//hellfalseoworld
//public void setCharAt(int index, char ch)修改对象中索引值为index位置的字符为新的字符ch
StringBuffer sb = new StringBuffer("helloworld");
sb.setCharAt(2,'a');;
System.out.println(sb);//healoworld
//public void trimToSize()存储空间缩小到和字符串长度,减少空间浪费
StringBuffer sb = new StringBuffer("helloworld");
sb.trimToSize();System.out.println(sb.capacity());//10
StringBuffer sb1 = new StringBuffer("helloworld");
System.out.println(sb1.capacity());//26
//public void setCharAt(int index, char ch)修改对象中索引值为index位置的字符为新的字符ch
StringBuffer sb = new StringBuffer("helloworld");
sb.setCharAt(2,'a');;
System.out.println(sb);//healoworld
4、String类型和StringBuffer、StringBuilder的区别
String 类型和StringBuffer的主要性能区别:String是不可变的对象, 因此在每次对String 类型进行改变的时候,都会生成一个新的String 对象,然后将指针指向新的 String 对象,所以经常改变内容的字符串最好不要用 String ,因为每次生成对象都会对系统性能产生影响,特别当内存中无引用对象多了以后, JVM 的 GC 就会开始工作,性能就会降低。使用 StringBuffer 类时,每次都会对 StringBuffer 对象本身进行操作,而不是生成新的对象并改变对象引用。所以多数情况下推荐使用StringBuffer ,特别是字符串对象经常改变的情况下。在某些特别情况下, String 对象的字符串拼接其实是被 Java Compiler编译成了 StringBuffer 对象的拼接,所以这些时候 String 对象的速度并不会比 StringBuffer 对象慢.StringBuilder与StringBuffer有公共父类AbstractStringBuilder(抽象类)。StringBuilder、StringBuffer的方法都会调用AbstractStringBuilder中 的公共方法,如super.append(...)。只是StringBuffer会在方法上加synchronized关键字,进行同步。最后,如果程序不是多线程的,那么使用StringBuilder效率高于StringBuffer。