命令行解析类CommandLineParser
该类的作用主要用于命令行的解析,也就是分解命令行的作用。以前版本没这个类时,如果要运行带参数的.exe,必须在命令行中输入文件路径以及各种参数,并且输入的参数格式要与代码中的if语句判断内容格式一样,很不方便。另外如果想要更改输入格式的话在主函数文件中要相应更改很多地方。现在有了这个类,只需要改keys里面的内容就可以了,并且运行时可以直接在vs下用F5,不需要cmd命令行带参运行。
在OpenCV源码中,其声明位于头文件 utility中。因此在使用前,一般需要进行头文件包含。
#include "utility.hpp"
类成员
public:
CommandLineParser (int argc, const char *const argv[], const String &keys);//构造函数
CommandLineParser (const CommandLineParser &parser);//拷贝构造函数
~CommandLineParser ();//析构函数
void about (const String &message);
bool check () const;
template<typename T >
T get (const String &name, bool space_delete=true) const;
template<typename T >
T get (int index, bool space_delete=true) const;
String getPathToApplication () const;
bool has (const String &name) const;
CommandLineParser & operator= (const CommandLineParser &parser);//赋值运算符重载
void printErrors () const;
void printMessage () const;
protected:
void getByIndex (int index, bool space_delete, int type, void *dst) const;
void getByName (const String &name, bool space_delete, int type, void *dst) const;
Impl * impl;
成员介绍
构造函数
CommandLineParser (int argc, const char *const argv[], const String &keys);//构造函数
argc : 命令行参数个数 (from main())
argv : 命令行参数数组 (from main())
keys : 一个描述了可以识别的命令行参数 (见keys语法)
Keys 语法
keys参数是一个包含多个块的字符串,每个部分对应的一个参数描述都由花括号括起来。参数描述分为三个部分,由 ” | “分隔开来:
- 1、参数名字,可以使用多个名称来表示同一个参数,但之间需要添加空格(参数名前可以使用前缀@标准时positional)
- 2、默认值,如果参数没有被提供则使用默认值
- 3、帮助信息
const String keys =
"{help h usage ? | | print this message }"
"{@image1 | | image1 for compare }"
"{@image2 |<none>| image2 for compare }"
"{@repeat |1 | number }"
"{path |. | path to file }"
"{fps | -1.0 | fps for output video }"
"{N count |100 | count of objects }"
"{ts timestamp | | use time stamp }"
;
源码
CommandLineParser::CommandLineParser(int argc, const char* const argv[], const String& keys)
{
impl = new Impl;
impl->refcount = 1;
// 应用路径
size_t pos_s = String(argv[0]).find_last_of("/\\");
if (pos_s == String::npos)
{
impl->path_to_app = "";
impl->app_name = String(argv[0]);
}
else
{
impl->path_to_app = String(argv[0]).substr(0, pos_s);
impl->app_name = String(argv[0]).substr(pos_s + 1, String(argv[0]).length() - pos_s);
}
impl->error = false;
impl->error_message = "";
// 解析参数key
std::vector<String> k = impl->split_range_string(keys, '{', '}');
int jj = 0;
for (size_t i = 0; i < k.size(); i++)
{
std::vector<String> l = impl->split_string(k[i], '|', true);
CommandLineParserParams p;
p.keys = impl->split_string(l[0]);
p.def_value = l[1];
p.help_message = cat_string(l[2]);
p.number = -1;
if (p.keys.size() <= 0)
{
impl->error = true;
impl->error_message = "Field KEYS could not be empty\n";
}
else
{
if (p.keys[0][0] == '@')
{
p.number = jj;
jj++;
}
impl->data.push_back(p);
}
}
// 解析参数agrv
jj = 0;
for (int i = 1; i < argc; i++)
{
String s(argv[i]);
bool hasSingleDash = s.length() > 1 && s[0] == '-';
if (hasSingleDash)
{
bool hasDoubleDash = s.length() > 2 && s[1] == '-';
String key = s.substr(hasDoubleDash ? 2 : 1);
String value = "true";
size_t equalsPos = key.find('=');
if(equalsPos != String::npos) {
value = key.substr(equalsPos + 1);
key = key.substr(0, equalsPos);
}
impl->apply_params(key, value);
}
else
{
impl->apply_params(jj, s);
jj++;
}
}
impl->sort_params();
}
成员函数
void about(const String & message);
设置about信息。当调用printMessage时会显示参数信息(参数列表右侧部分)
bool check();
当访问参数时发生错误(转换错误、参数缺失等)后会返回false;并调用printErrors打印错误列表
template<typename T > T get (const String &name, bool space_delete=true) const;
template<typename T > T get (int index, bool space_delete=true) const;
用于获取positional参数,并将返回值转换为选定的类型。
name: 参数名字
index: 参数位置索引(从0开始)
space_delete: 从左至右移除字符串中的空格
bool has(const String &name) const
检测某个域是否存在
name: 被检测的参数名称
扫描二维码关注公众号,回复:
2853550 查看本文章
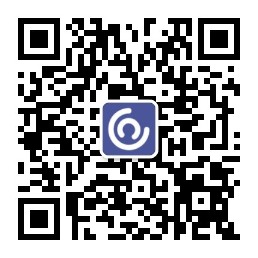
样例
CommandLineParser parser(argc, argv, keys);
parser.about("Application name v1.0.0");
if (parser.has("help"))
{
parser.printMessage();
return 0;
}
int N = parser.get<int>("N");
double fps = parser.get<double>("fps");
String path = parser.get<String>("path");
use_time_stamp = parser.has("timestamp");
String img1 = parser.get<String>(0);
String img2 = parser.get<String>(1);
int repeat = parser.get<int>(2);
if (!parser.check())
{
parser.printErrors();
return 0;
}
Reference
[1] OpenCV官网
[2] OpenCV中CommandLineParser类