1. 前言
ContentProvider为存储和获取数据提供统一的接口,可以在不同的应用程序之间共享数据。
2. 读取通讯录
常通过ContentProvider来读取通讯录的信息。
Cursor cursor = getContentResolver().query(ContactsContract.Contacts.CONTENT_URI,
null, null, null, null);
while (cursor.moveToNext()) {
int id = cursor.getInt(cursor.getColumnIndex(
ContactsContract.Contacts._ID));
String name = cursor.getString(cursor.getColumnIndex(
ContactsContract.Contacts.DISPLAY_NAME));
}
cursor.close();
需要在AndroidManifest.xml中添加权限。
<uses-permission android:name="android.permission.READ_CONTACTS" />
2. 自定义ContentProvider
自定义ContentProvider利用数据库提供了数据,并对数据进行查询和修改操作。
用户数据库
利用SQLiteOpenHelper创建,数据库包含ID、姓名、地址和年龄。
public class PeopleSQLiteOpenHelper extends SQLiteOpenHelper {
public final static String LOGTAG = "PeopleSQLiteOpenHelper";
public final static String DB_NAME = "people.db";
public final static String TABLE_NAME = "people";
public final static int VERSION = 1;
public final static String COL_ID = "_id";
public final static String COL_NAME = "name";
public final static String COL_ADDR = "addr";
public final static String COL_AGE = "age";
public final static String TABLE_CREATE =
"create table if not exists " + TABLE_NAME + "("
+ COL_ID + " integer primary key autoincrement not null,"
+ COL_NAME + " text not null, "
+ COL_ADDR + " text not null, "
+ COL_AGE + " integer "
+ ")";
public PeopleSQLiteOpenHelper(Context context) {
super(context, DB_NAME, null, VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(TABLE_CREATE);
}
}
自定义ContentProvider
在自定义ContentProvider之前,为了安全需要添加自定义权限。
<!-- 定义读取权限 -->
<permission android:name="com.blog.demo.READ_PEOPLE" />
<!-- 定义修改权限 -->
<permission android:name="com.blog.demo.WRITE_PEOPLE" />
同时也要添加ContentProvider声明,指定读取和写入权限。
<!-- authorities是标识,访问时需要 -->
<!-- 设置export为true,对其他apk开放 -->
<provider
android:authorities="com.blog.demo.people"
android:name=".component.cont.PeopleContentProvider"
android:readPermission="com.blog.demo.READ_PEOPLE"
android:writePermission="com.blog.demo.WRITE_PEOPLE" />
自定义PeopleContentProvider继承ContentProvider,实现了查询、插入、删除和修改四类操作。
定义 people 和 people/# 两种不同uri请求,并利用UriMatcher来解析。people 代表所有用户,people/# 代表某个用户。
public class PeopleContentProvider extends ContentProvider {
private static final String AUTHORITY = "com.blog.demo.people";
private static final String TABLE_NAME = PeopleSQLiteOpenHelper.TABLE_NAME;
private static final int PEOPLE = 1;
private static final int PEOPLE_ID = 2;
private static final UriMatcher uriMatcher;
static {
uriMatcher = new UriMatcher(UriMatcher.NO_MATCH);
uriMatcher.addURI(AUTHORITY, "people", PEOPLE);
uriMatcher.addURI(AUTHORITY, "people/#", PEOPLE_ID);
}
private PeopleSQLiteOpenHelper helper;
@Nullable
@Override
public String getType(@NonNull Uri uri) {
switch(uriMatcher.match(uri)) {
case PEOPLE: // 集合类型 vnd.android.cursor.dir
return "vnd.android.cursor.dir/com.blog.demo.people";
case PEOPLE_ID: // 非集合类型 vnd.android.cursor.item
return "vnd.android.cursor.item/com.blog.demo.people";
default:
throw new IllegalArgumentException("Unsupported URI: " + uri);
}
}
@Override
public boolean onCreate() {
helper = new PeopleSQLiteOpenHelper(getContext());
return true;
}
@Nullable
@Override
public Cursor query(@NonNull Uri uri, @Nullable String[] projection, @Nullable String selection,
@Nullable String[] selectionArgs, @Nullable String sortOrder) {
if (uriMatcher.match(uri) == PEOPLE) {
// 查询所有people数据
return helper.getReadableDatabase().query(TABLE_NAME, projection,
selection, selectionArgs, null, null, sortOrder);
} else if (uriMatcher.match(uri) == PEOPLE_ID) {
// 查询所有指定id的people数据
String id = uri.getPathSegments().get(1);
return helper.getReadableDatabase().query(TABLE_NAME, projection,
PeopleSQLiteOpenHelper.COL_ID +"=?", new String[]{id},
null, null, sortOrder);
}
throw new IllegalArgumentException("Unsupported URI: " + uri);
}
@Nullable
@Override
public Uri insert(@NonNull Uri uri, @Nullable ContentValues values) {
if (uriMatcher.match(uri) == PEOPLE) {
SQLiteDatabase db = helper.getWritableDatabase();
try {
db.beginTransaction();
long id = db.insert(TABLE_NAME, null, values);
if (id > 0) {
db.setTransactionSuccessful();
return ContentUris.withAppendedId(uri, id);
}
} finally {
db.endTransaction();
}
}
throw new IllegalArgumentException("Unsupported URI: " + uri);
}
@Override
public int delete(@NonNull Uri uri, @Nullable String selection, @Nullable String[] selectionArgs) {
if (uriMatcher.match(uri) == PEOPLE_ID) {
String id = uri.getPathSegments().get(1);
SQLiteDatabase db = helper.getWritableDatabase();
try {
db.beginTransaction();
int result = db.delete(TABLE_NAME,
PeopleSQLiteOpenHelper.COL_ID +"=?", new String[]{id});
db.setTransactionSuccessful();
return result;
} finally {
db.endTransaction();
}
}
throw new IllegalArgumentException("Unsupported URI: " + uri);
}
@Override
public int update(@NonNull Uri uri, @Nullable ContentValues values, @Nullable String selection,
@Nullable String[] selectionArgs) {
if (uriMatcher.match(uri) == PEOPLE_ID) {
String id = uri.getPathSegments().get(1);
SQLiteDatabase db = helper.getWritableDatabase();
try {
db.beginTransaction();
int result = db.update(TABLE_NAME, values,
PeopleSQLiteOpenHelper.COL_ID +"=?", new String[]{id});
db.setTransactionSuccessful();
return result;
} finally {
db.endTransaction();
}
}
throw new IllegalArgumentException("Unsupported URI: " + uri);
}
}
3. 读取用户数据
需要添加读取权限。
<uses-permission android:name="com.blog.demo.READ_PEOPLE"/>
<uses-permission android:name="com.blog.demo.WRITE_PEOPLE"/>
查询操作,查询所有用户信息和根据ID查询某个用户信息。
private void query() {
List<People> list = new ArrayList<>();
Cursor cursor = getContentResolver().query(PeopleConstant.CONTENT_URI,
null, null, null, null);
if (cursor != null) {
while (cursor.moveToNext()) {
int id = cursor.getInt(cursor.getColumnIndex(PeopleColumns.ID));
String name = cursor.getString(cursor.getColumnIndex(PeopleColumns.NAME));
String addr = cursor.getString(cursor.getColumnIndex(PeopleColumns.ADDR));
int age = cursor.getInt(cursor.getColumnIndex(PeopleColumns.AGE));
list.add(new People(id, name, addr, age));
}
cursor.close();
}
}
private void query(int id) {
Cursor cursor = getContentResolver().query(
ContentUris.withAppendedId(PeopleConstant.CONTENT_URI, id),
null, null, null, null);
if (cursor != null) {
People people = null;
if (cursor.moveToNext()) {
int rid = cursor.getInt(cursor.getColumnIndex(PeopleColumns.ID));
String name = cursor.getString(cursor.getColumnIndex(PeopleColumns.NAME));
String addr = cursor.getString(cursor.getColumnIndex(PeopleColumns.ADDR));
int age = cursor.getInt(cursor.getColumnIndex(PeopleColumns.AGE));
people = new People(rid, name, addr, age);
}
cursor.close();
}
}
添加、修改和删除操作。
扫描二维码关注公众号,回复:
2815418 查看本文章
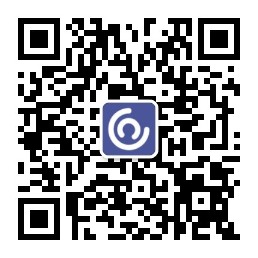
private void insert(String name, String addr, int age) {
ContentValues insertValues = new ContentValues();
insertValues.put(PeopleColumns.NAME, name);
insertValues.put(PeopleColumns.ADDR, addr);
insertValues.put(PeopleColumns.AGE, age);
getContentResolver().insert(PeopleConstant.CONTENT_URI, insertValues);
}
private void update(int id, String name, String addr, int age) {
ContentValues updateValues = new ContentValues();
updateValues.put(PeopleColumns.NAME, name);
updateValues.put(PeopleColumns.ADDR, addr);
updateValues.put(PeopleColumns.AGE, age);
getContentResolver().update(ContentUris.withAppendedId(
PeopleConstant.CONTENT_URI, id),
updateValues, null, null);
}
private void delete(int id) {
getContentResolver().delete(ContentUris.withAppendedId(
PeopleConstant.CONTENT_URI, id), null, null);
}
辅助类PeopleConstant。
public class PeopleConstant {
public final static Uri CONTENT_URI =
Uri.parse("content://com.blog.demo.people/people");
public final static class PeopleColumns {
public final static String ID = "_id";
public final static String NAME = "name";
public final static String ADDR = "addr";
public final static String AGE = "age";
}
}