1.练习:找到一个大于100k的文件,按照100k为单位,拆分成多个子文件,并且以编号作为文件名结束。
一个222k的txt文件,拆分之后
什么是流(Stream),流就是一系列的数据
InputStream字节输入流
OutputStream字节输出流
用于以字节的形式读取和写入数据import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Arrays;
public class SplitFile {
/**
* 拆分的思路,先把源文件的所有内容读取到内存中,然后从内存中挨个分到子文件里
* @param srcFile 要拆分的源文件
* @param eachSize 按照这个大小,拆分
*/
public static void split(File srcFile,int eachSize){
if (0 == srcFile.length())
throw new RuntimeException("文件长度为0,不可拆分");
//创建一个文件内容数组,大小为原文件大小
byte[] fileContent = new byte[(int)srcFile.length()];
try {
FileInputStream fis = new FileInputStream(srcFile);//创建基于文件的输入流
fis.read(fileContent);//将文件读取到数组中,
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
int fileNumber ;//拆分的份数
if (0 == srcFile.length()%eachSize)
fileNumber =(int)srcFile.length()/eachSize;
else
fileNumber =(int)srcFile.length()/eachSize+1;
for (int i=1;i<=fileNumber;i++){
String eachFileName = srcFile.getName()+"-"+i;
File eachFile = new File(srcFile.getParent(),eachFileName); //getParent()返回此抽象路径名父目录的路径名字符串
byte[] eachContent;
// 从源文件的内容里,复制部分数据到子文件
// 除开最后一个文件,其他文件大小都是100k
// 最后一个文件的大小是剩余的
// copyOfRange(byte[] original, int from, int to)将指定数组的指定范围复制到一个新数组
if (i!=fileNumber){//如果不是最后一个
eachContent = Arrays.copyOfRange(fileContent,eachSize*(i-1),eachSize*i);//from从哪里开始,to到哪里结束
}else {
eachContent = Arrays.copyOfRange(fileContent,eachSize*(i-1),fileContent.length);
}
try {
FileOutputStream fos = new FileOutputStream(eachFile);
fos.write(eachContent);
fos.close();
System.out.println("子文件"+eachFile.getAbsolutePath()+",其大小是"+eachFile.length()+"字节");
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
long eachSize = 100*1024;//需要拆的每一块大小100k
File srcFile = new File("E:/file/LOL.txt");
split(srcFile,(int)eachSize);
}
}
更多学习资源尽在http://how2j.cn?p=42562
扫描二维码关注公众号,回复:
2789726 查看本文章
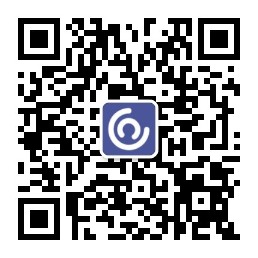
研究了好久文件合并,应该是最笨的方法QAQ,等改进吧,嘻嘻
public void merge(){
try {
File eachFile1 = new File("E:/file/LOL.txt-1");
File eachFile2 = new File("E:/file/LOL.txt-2");
File eachFile3 = new File("E:/file/LOL.txt-2");
File newFile = new File("E:/file/combin.txt");
FileOutputStream fos = new FileOutputStream(newFile);
FileInputStream f1 = new FileInputStream(eachFile1);
FileInputStream f2 = new FileInputStream(eachFile2);
FileInputStream f3 = new FileInputStream(eachFile2);
byte[] eachContent = new byte[(int)(eachFile1.length())];
f1.read(eachContent);
fos.write(eachContent);
f1.close();
f2.read(eachContent);
f2.close();
fos.write(eachContent);
f3.read(eachContent);
f3.close();
fos.write(eachContent);
fos.flush();
System.out.println("写入成功");
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}