1.HashMap数据结构
首先先看下HashMap的数据结构图
我们都知道数组的储存方式在是连续的,查找速度比较快,但插入和删除数据比较慢
而链表的存储方式是非连续的,所以插入和删除速度较快,但查找速度就比较慢,HashMap在数据结构上两种都采用了。
/**
* The table, initialized on first use, and resized as
* necessary. When allocated, length is always a power of two.
* (We also tolerate length zero in some operations to allow
* bootstrapping mechanics that are currently not needed.)
*/
transient Node<K,V>[] table;
再看下Node中的变量 (Node中含有hash值、key、value以及指向下个节点的next)
final int hash;
final K key;
V value;
Node<K,V> next;
Node(int hash, K key, V value, Node<K,V> next) {
this.hash = hash;
this.key = key;
this.value = value;
this.next = next;
}
通过这个table变量我们就能理解上面那个数据结构图,它不仅使用了数组,而且还采用了链表结构。
2.HashMap变量
首先我们看下HashMap中的变量,在看之前思考几个问题:
1. HashMap数据结构既然使用了数组,那么数组默认内存大小是多少?
2.当已使用内存大小超过总内存的一个百分比后,需要继续增大内存,这个百分比是多少?
3.当链表节点长多超过多少时储存结构变成红黑树?
/**
* The default initial capacity - MUST be a power of two.
*/
static final int DEFAULT_INITIAL_CAPACITY = 1 << 4; // aka 16
由此可以知道数组的默认大小是16
扫描二维码关注公众号,回复:
2756261 查看本文章
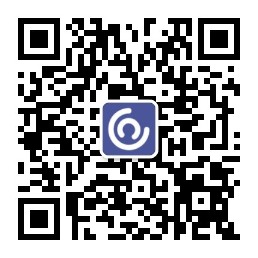
/**
* The maximum capacity, used if a higher value is implicitly specified
* by either of the constructors with arguments.
* MUST be a power of two <= 1<<30.
*/
static final int MAXIMUM_CAPACITY = 1 << 30;
当数组不够大的时候,就会增加内存大小,大小的上限为1<<30,也就是2的30次方
/**
* The load factor used when none specified in constructor.
*/
static final float DEFAULT_LOAD_FACTOR = 0.75f;
这个变量就是问题2的答案,也被称为负载因子,默认为0.75
/**
* The bin count threshold for using a tree rather than list for a
* bin. Bins are converted to trees when adding an element to a
* bin with at least this many nodes. The value must be greater
* than 2 and should be at least 8 to mesh with assumptions in
* tree removal about conversion back to plain bins upon
* shrinkage.
*/
static final int TREEIFY_THRESHOLD = 8;
这个变量就是问题三的答案,当节点数量超过8时,储存结构转换成红黑树
/**
* The bin count threshold for untreeifying a (split) bin during a
* resize operation. Should be less than TREEIFY_THRESHOLD, and at
* most 6 to mesh with shrinkage detection under removal.
*/
static final int UNTREEIFY_THRESHOLD = 6;
当bin的个数小于6时由树形结构调整为列表
/**
* The number of key-value mappings contained in this map.
*/
transient int size;
当前HashMap中储存key-value的大小
/**
* The number of times this HashMap has been structurally modified
* Structural modifications are those that change the number of mappings in
* the HashMap or otherwise modify its internal structure (e.g.,
* rehash). This field is used to make iterators on Collection-views of
* the HashMap fail-fast. (See ConcurrentModificationException).
*/
transient int modCount;
HashMap被修改和删除的次数
3.HashMap的储存过程
HashMap hashMap = new HashMap();
hashMap.put("1","1230");
System.out.print(hashMap.get("1"));
我们从hashMap.put()方法开始
public V put(K key, V value) {
return putVal(hash(key), key, value, false, true);
}
这里详细讲下hash()函数,首先先看源码:
static final int hash(Object key) {
int h;
return (key == null) ? 0 : (h = key.hashCode()) ^ (h >>> 16);
}
如果key的值不为空的话,就把变量h等于key值的hashCode,然后把h的高16位与低16位进行一个异或运算,这样得到的值和数组的默认长度16相对应,方便后续计算该Node存储的数组下标是多少。
未完。。。。待续