数据结构与算法 线性表
一、简述
记-线性表。线性表是具有相同特性的数据元素的一个有限序列。
线性表的顺序存储结构--顺序表--把线性表中的所有元素按照其逻辑顺序依次存储到计算机中指定存储位置开始的一块连续的存储空间。
线性表的链式存储结构--链表--可以实现存储空间动态管理的链式存储方式。
顺序存储特点:内存上是一片连续的空间,通常分配时就指定大小。通常有一个索引指示这片内存空间的使用情况。(比如一个数组,在配上一个int类型的变量i,i用来指示当前存储到那个位置了,也就是说i指示线性表的实际长度)。
链式存储特点:存储空间可伸缩,内存上可以是分散的,各个节点保存有下一个节点的地址信息,通过地址信息将所有的节点串联起来。
二、顺序表
顺序表例子。输入正数添加到顺序表中,输入负数删除对应节点,输入0清空顺序。比如输入11,将11添加到顺序表中,输入-11,将11删除。
#include <stdio.h>
#include <stdlib.h>
#define SIZE 5
typedef struct sequence_list
{
int data[SIZE];
int size;
}Seq;
Seq* init_seq()
{
Seq* sp;
sp = (Seq*)malloc(sizeof(struct sequence_list));
if(sp!=NULL)
sp->size = -1;
return sp;
}
int is_full(Seq* sp)
{
return (sp->size == SIZE-1);
}
int add_data(Seq* sp,int data)
{
if(is_full(sp))
return -1;
sp->data[++sp->size] = data;
return 0;
}
void display_seq(Seq* sp)
{
int i;
for(i=0;i<=sp->size;++i)
{
printf("data[%d]=%d \n",i,sp->data[i]);
}
}
void remove_data(Seq* sp,int data)
{
if(sp==NULL)
{
printf("Seq is empty!\n");
return;
}
int i;
for(i=0;i<=sp->size;++i)
{
if(sp->data[i]==data)
{
int j;
for(j=i+1;j<=sp->size;++j)
{
sp->data[i]=sp->data[j];
}
sp->size--;
}
}
return;
}
void clear_seq(Seq* sp)
{
free(sp);
sp=NULL;
return ;
}
int main(void)
{
int data;
Seq* sp = init_seq();
if(sp == NULL)
{
printf("malloc failed! \n");
return -1;
}
while(1)
{
scanf("%d",&data);
if(data>0)
{
add_data(sp,data);
display_seq(sp);
}
else
{
if(data<0)
{
remove_data(sp,-data);
display_seq(sp);
}
else
{
clear_seq(sp);
break;
}
}
}
return 0;
}
运行效果截图:
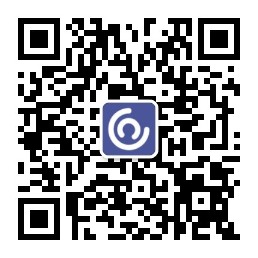
三、线性表
单向链表、单向循环链表、双向链表、双向循环链表。(例子中链表头结点均不存放数据,增加节点方式为头插法)
3.1单向链表
例子:输入正数添加到链表中,输入负数删除对应节点,输入0清空链表。比如输入11,将11添加到链表(头插法),输入-11,将11删除。
#include <stdio.h>
#include <stdlib.h>
typedef struct list_node
{
int data;
struct list_node* next;
}Node;
Node* init_list()
{
Node* head = (Node*)malloc(sizeof(Node));
if(head!=NULL)
{
head->next = NULL;
}
return head;
}
Node* create_node(int data)
{
Node* new_node = (Node*)malloc(sizeof(Node));
if(new_node!=NULL)
{
new_node->next = NULL;
new_node->data = data;
}
return new_node;
}
void add_node2list(Node* head,Node* new_node)
{
if(head==NULL)
{
return;
}
new_node->next = head->next;
head->next = new_node;
}
void display_list(Node* head)
{
if(head==NULL)
{
return;
}
Node* p = head;
while(p=p->next)
{
printf("data===%d \n",p->data);
}
return;
}
void remove_node4list(Node* head,int data)
{
if(head==NULL || head->next == NULL)
{
printf("list is empty!\n");
return;
}
Node* np1 = head,*np2 = head;
while(np2=np2->next)
{
if(np2->data==data)
{
np1->next = np2->next;
free(np2);
break;
}
np1 = np2;
}
return;
}
void free_list(Node* head)
{
if(head==NULL || head->next == NULL)
{
return;
}
Node* np = head;
while(np = head->next)
{
head->next = np->next;
printf("free=%d\n",np->data);
free(np);
}
return;
}
int main(int argc,char* argv[])
{
int data;
Node* head = init_list();
if(head==NULL)
{
printf("init failed!\n");
return -1;
}
while(1)
{
scanf("%d",&data);
if(data>0)
{
Node* new_node = create_node(data);
if(new_node==NULL)
{
printf("create node failed!\n");
continue;
}
add_node2list(head,new_node);
display_list(head);
}
else
{
if(data==0)
{
free_list(head);
break;
}
else
{
remove_node4list(head,-data);
display_list(head);
}
}
}
free(head);
return 0;
}
运行效果截图:
3.2单向循环链表
例子:
#include <stdio.h>
#include <stdlib.h>
typedef struct list_node
{
int data;
struct list_node* next;
}Node;
Node* init_list()
{
Node* head = (Node*)malloc(sizeof(Node));
if(head!=NULL)
{
head->next = head;
}
return head;
}
Node* create_node(int data)
{
Node* new_node = (Node*)malloc(sizeof(Node));
if(new_node!=NULL)
{
new_node->next = NULL;
new_node->data = data;
}
return new_node;
}
void add_node2list(Node* head,Node* new_node)
{
if(head==NULL)
{
return;
}
new_node->next = head->next;
head->next = new_node;
}
void display_list(Node* head)
{
if(head==NULL)
{
return;
}
Node* p = head;
while((p=p->next) != head)
{
printf("data===%d \n",p->data);
}
return;
}
void remove_node4list(Node* head,int data)
{
if(head==NULL)
{
printf("list is empty!\n");
return;
}
Node* np1 = head,*np2 = head;
while((np2=np2->next) != head)
{
if(np2->data==data)
{
np1->next = np2->next;
free(np2);
break;
}
np1 = np2;
}
return;
}
void free_list(Node* head)
{
if(head==NULL)
{
return;
}
Node* np = head;
while((np = head->next) != head)
{
head->next = np->next;
printf("free=%d\n",np->data);
free(np);
}
return;
}
int main(int argc,char* argv[])
{
int data;
Node* head = init_list();
if(head==NULL)
{
printf("init failed!\n");
return -1;
}
while(1)
{
scanf("%d",&data);
if(data>0)
{
Node* new_node = create_node(data);
if(new_node==NULL)
{
printf("create node failed!\n");
continue;
}
add_node2list(head,new_node);
display_list(head);
}
else
{
if(data==0)
{
free_list(head);
break;
}
else
{
remove_node4list(head,-data);
display_list(head);
}
}
}
free(head);
return 0;
}
运行效果截图
3.3双向链表
测试代码
#include <stdio.h>
#include <stdlib.h>
typedef struct s_list
{
int data;
struct s_list* next;
struct s_list* prev;
}Node;
Node* init_list()
{
Node* head = (Node*)malloc(sizeof(struct s_list));
if(head==NULL)
{
return NULL;
}
head->next = NULL;
head->prev = NULL;
return head;
}
Node* create_node(int data)
{
Node* new_node = (Node*)malloc(sizeof(struct s_list));
if(new_node==NULL)
{
return NULL;
}
new_node->data = data;
new_node->next = NULL;
new_node->prev = NULL;
return new_node;
}
void add_node(Node* head,Node* new_node)
{
if(head==NULL)
{
return;
}
new_node->next = head->next;
if(head->next != NULL)
{
head->next->prev = new_node;
}
new_node->prev = head;
head->next = new_node;
}
void display_list(Node* head)
{
Node* np = head;
while((np=np->next)!= NULL)
{
printf("data==%d\n",np->data);
}
}
void free_list(Node* head)
{
Node* np = head;
while((np=np->next) != NULL)
{
head->next = np->next;
printf("free==%d \n",np->data);
free(np);
}
head->next = NULL;
}
void remove_node(Node* head,int data)
{
Node* np = head;
while((np=np->next)!= NULL)
{
if(np->data==data)
{
np->prev->next = np->next;
if(np->next != NULL)
{
np->next->prev = np->prev;
}
free(np);
break;
}
}
}
int main(int argc,char* argv[])
{
int data;
Node* head = init_list();
while(1)
{
scanf("%d",&data);
if(data>0)
{
Node* new_node = create_node(data);
add_node(head,new_node);
display_list(head);
}
else
{
if(data==0)
{
free_list(head);
break;
}
else
{
remove_node(head,-data);
display_list(head);
}
}
while(getchar()!='\n');
}
free(head);
return 0;
}
运行结果
3.4双向循环链表
测试代码
#include <stdio.h>
#include <stdlib.h>
typedef struct s_list
{
int data;
struct s_list* next;
struct s_list* prev;
}Node;
Node* init_list()
{
Node* head = (Node*)malloc(sizeof(struct s_list));
if(head==NULL)
{
return NULL;
}
head->next = head;
head->prev = head;
return head;
}
Node* create_node(int data)
{
Node* new_node = (Node*)malloc(sizeof(struct s_list));
if(new_node==NULL)
{
return NULL;
}
new_node->data = data;
new_node->next = NULL;
new_node->prev = NULL;
return new_node;
}
void add_node(Node* head,Node* new_node)
{
if(head==NULL)
{
return;
}
new_node->next = head->next;
head->next->prev = new_node;
new_node->prev = head;
head->next = new_node;
return;
}
void display_list(Node* head)
{
Node* np = head;
while((np=np->next)!=head)
{
printf("data==%d\n",np->data);
}
}
void free_list(Node* head)
{
Node* np = head;
while((np=np->next)!=head)
{
head->next = np->next;
printf("free==%d \n",np->data);
free(np);
}
head->next = head;
head->prev = head;
}
void remove_node(Node* head,int data)
{
Node* np = head;
while((np=np->next)!=head)
{
if(np->data==data)
{
np->prev->next = np->next;
np->next->prev = np->prev;
free(np);
break;
}
}
}
int main(int argc,char* argv[])
{
int data;
Node* head = init_list();
while(1)
{
scanf("%d",&data);
if(data>0)
{
Node* new_node = create_node(data);
add_node(head,new_node);
display_list(head);
}
else
{
if(data==0)
{
free_list(head);
break;
}
else
{
remove_node(head,-data);
display_list(head);
}
}
while(getchar()!='\n');
}
free(head);
return 0;
}
运行效果截图
四、补充
Linux内核链表。(双向链表,非原版)
#ifndef __DLIST_H
#define __DLIST_H
/* This file is from Linux Kernel (include/linux/list.h)
* and modified by simply removing hardware prefetching of list items.
* Here by copyright, credits attributed to wherever they belong.
* Kulesh Shanmugasundaram (kulesh [squiggly] isis.poly.edu)
*/
/*
* Simple doubly linked list implementation.
*
* Some of the internal functions (“__xxx”) are useful when
* manipulating whole lists rather than single entries, as
* sometimes we already know the next/prev entries and we can
* generate better code by using them directly rather than
* using the generic single-entry routines.
*/
/**
* container_of - cast a member of a structure out to the containing structure
*
* @ptr: the pointer to the member.
* @type: the type of the container struct this is embedded in.
* @member: the name of the member within the struct.
*
*/
#define offsetof(TYPE, MEMBER) ((size_t) &((TYPE *)0)->MEMBER)
#define container_of(ptr, type, member) ({ \
const typeof( ((type *)0)->member ) *__mptr = (ptr); \
(type *)( (char *)__mptr - offsetof(type,member) );})
/*
* These are non-NULL pointers that will result in page faults
* under normal circumstances, used to verify that nobody uses
* non-initialized list entries.
*/
#define LIST_POISON1 ((void *) 0x00100100)
#define LIST_POISON2 ((void *) 0x00200)
struct list_head {
struct list_head *next, *prev;
};
//初始化
#define LIST_HEAD_INIT(name) { &(name), &(name) }
#define LIST_HEAD(name) \
struct list_head name = LIST_HEAD_INIT(name)
#define INIT_LIST_HEAD(ptr) do { \
(ptr)->next = (ptr); (ptr)->prev = (ptr); \
} while (0)
/*
* Insert a new entry between two known consecutive entries.
*
* This is only for internal list manipulation where we know
* the prev/next entries already!
*/
//插入节点
static inline void __list_add(struct list_head *new,
struct list_head *prev,
struct list_head *next)
{
next->prev = new;
new->next = next;
new->prev = prev;
prev->next = new;
}
/**
* list_add – add a new entry
* @new: new entry to be added
* @head: list head to add it after
*
* Insert a new entry after the specified head.
* This is good for implementing stacks.
*/
//头插
static inline void list_add(struct list_head *new, struct list_head *head)
{
__list_add(new, head, head->next);
}
/**
* list_add_tail – add a new entry
* @new: new entry to be added
* @head: list head to add it before
*
* Insert a new entry before the specified head.
* This is useful for implementing queues.
*/
//尾插
static inline void list_add_tail(struct list_head *new, struct list_head *head)
{
__list_add(new, head->prev, head);
}
/*
* Delete a list entry by making the prev/next entries
* point to each other.
*
* This is only for internal list manipulation where we know
* the prev/next entries already!
*/
static inline void __list_del(struct list_head *prev, struct list_head *next)
{
next->prev = prev;
prev->next = next;
}
/**
* list_del – deletes entry from list.
* @entry: the element to delete from the list.
* Note: list_empty on entry does not return true after this, the entry is in an undefined state.
*/
static inline void list_del(struct list_head *entry)
{
__list_del(entry->prev, entry->next);
entry->next = (void *) 0;
entry->prev = (void *) 0;
}
/**
* list_del_init – deletes entry from list and reinitialize it.
* @entry: the element to delete from the list.
*/
static inline void list_del_init(struct list_head *entry)
{
__list_del(entry->prev, entry->next);
INIT_LIST_HEAD(entry);
}
/**
* list_move – delete from one list and add as another’s head
* @list: the entry to move
* @head: the head that will precede our entry
*/
static inline void list_move(struct list_head *list,
struct list_head *head)
{
__list_del(list->prev, list->next);
list_add(list, head);
}
/**
* list_move_tail – delete from one list and add as another’s tail
* @list: the entry to move
* @head: the head that will follow our entry
*/
static inline void list_move_tail(struct list_head *list,
struct list_head *head)
{
__list_del(list->prev, list->next);
list_add_tail(list, head);
}
/**
* list_empty – tests whether a list is empty
* @head: the list to test.
*/
static inline int list_empty(struct list_head *head)
{
return head->next == head;
}
static inline void __list_splice(struct list_head *list,
struct list_head *head)
{
struct list_head *first = list->next;
struct list_head *last = list->prev;
struct list_head *at = head->next;
first->prev = head;
head->next = first;
last->next = at;
at->prev = last;
}
/**
* list_splice – join two lists
* @list: the new list to add.
* @head: the place to add it in the first list.
*/
static inline void list_splice(struct list_head *list, struct list_head *head)
{
if (!list_empty(list))
__list_splice(list, head);
}
/**
* list_splice_init – join two lists and reinitialise the emptied list.
* @list: the new list to add.
* @head: the place to add it in the first list.
*
* The list at @list is reinitialised
*/
static inline void list_splice_init(struct list_head *list,
struct list_head *head)
{
if (!list_empty(list)) {
__list_splice(list, head);
INIT_LIST_HEAD(list);
}
}
/**由结构体成员变量地址获取出结构体的首地址
* list_entry – get the struct for this entry
* @ptr: the &struct list_head pointer.
* @type: the type of the struct this is embedded in.
* @member: the name of the list_struct within the struct.
*/
#define list_entry(ptr, type, member) \
((type *)((char *)(ptr)-(unsigned long)(&((type *)0)->member)))
/**
* list_for_each - iterate over a list
* @pos: the &struct list_head to use as a loop counter.
* @head: the head for your list.
*/
#define list_for_each(pos, head) \
for (pos = (head)->next; pos != (head); \
pos = pos->next)
/**
* list_for_each_prev - iterate over a list backwards
* @pos: the &struct list_head to use as a loop counter.
* @head: the head for your list.
*/
#define list_for_each_prev(pos, head) \
for (pos = (head)->prev; pos != (head); \
pos = pos->prev)
/**
* list_for_each_safe - iterate over a list safe against removal of list entry
* @pos: the &struct list_head to use as a loop counter.
* @n: another &struct list_head to use as temporary storage
* @head: the head for your list.
*/
#define list_for_each_safe(pos, n, head) \
for (pos = (head)->next, n = pos->next; pos != (head); \
pos = n, n = pos->next)
/**
* list_for_each_entry - iterate over list of given type
* @pos: the type * to use as a loop counter.
* @head: the head for your list.
* @member: the name of the list_struct within the struct.
*/
#define list_for_each_entry(pos, head, member) \
for (pos = list_entry((head)->next, typeof(*pos), member); \
&pos->member != (head); \
pos = list_entry(pos->member.next, typeof(*pos), member))
/**
* list_for_each_entry_safe – iterate over list of given type safe against removal of list entry
* @pos: the type * to use as a loop counter.
* @n: another type * to use as temporary storage
* @head: the head for your list.
* @member: the name of the list_struct within the struct.
*/
#define list_for_each_entry_safe(pos, n, head, member) \
for (pos = list_entry((head)->next, typeof(*pos), member), \
n = list_entry(pos->member.next, typeof(*pos), member); \
&pos->member != (head); \
pos = n, n = list_entry(n->member.next, typeof(*n), member))
#endif
六、总结
1、单链表
插入节点
删除节点
2、双链表
插入节点
删除节点