1、数据格式化
步骤:
①创建bean并且用@Repository修饰bean类
②在bean中对需要格式化的参数使用@NumberFormat或@DateTimeFormat修饰
③编写对应的dao
④编写handler
⑤在spring配置文件中配置mvc:annotation-driven标签
注意:handler中接收form表单的方法的参数不用@RequestParam修饰
2、数据校验
步骤:
①加入jar,其中classmate和jboss-logging和validation-api是必须要加的,不然会报一下NoClassDefFoundError的错
classmate-1.3.4.jar
hibernate-validator-5.4.2.Final.jar
hibernate-validator-annotation-processor-5.4.2.Final.jar
hibernate-validator-cdi-5.4.2.Final.jar
jboss-logging-3.3.2.Final.jar
validation-api-2.0.1.Final.jar
②在spring配置文件中配置mvc:annotation-driven标签
③创建bean并且用@Repository修饰bean类
④编写对应的dao
⑤编写handler,并且在处理请求的方法的参数前面使用@Valid修饰
⑥在bean中对需要验证的参数使用验证标签修饰,如:@Email/@NotEmpty
注意:如果加入jar之后,类报错The type javax.validation.Payload cannot be resolved,则需要添加validation-api-x.x.x.Final.jar
3、错误提示
步骤:
①完成数据校验的代码
②在需要显示错误提示的form里面配置modelAttribute
③在需要显示错误提示的form里面添加form:errors标签(path参数值如果是”*”,则代表显示所有错误信息,值是参数名,则只显示对应参数名的错误信息)
4、错误信息国际化
步骤:
①创建i18n文件
②在springmvc配置文件中配置国际化资源
注意:
在国际化资源文件中@NumberFormat和@DateTimeFormat修饰的参数需要使用:typeMismatch.bean类名(首字母小写).参数名, 如:typeMismatch.info.infoDate=xxx
在国际化资源文件中数据校验标签修饰的参数需要使用:标签名.bean类名(首字母小写).参数名, 如:NotEmpty.info.notEmptyData=xxx
5、返回json
步骤:
①添加jar包
jackson-annotations-2.9.6.jar
jackson-core-2.9.6.jar
jackson-databind-2.9.6.jar
②在handler里面写处理方法,并且返回数据(集合类型的数据)
③页面加载jquery接收数据
注意:
①返回的结果会直接输出到页面上,所以可以直接用来作为返回json数据的接口
②handler中被调用的方法需要用@ResponseBody修饰
6、web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<display-name>SpringMVC_9_Data</display-name>
<servlet>
<servlet-name>springDispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springDispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<filter>
<filter-name>HiddenHttpMethodFilter</filter-name>
<filter-class>org.springframework.web.filter.HiddenHttpMethodFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>HiddenHttpMethodFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
7、springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd">
<!-- 配置自动扫描包 -->
<context:component-scan base-package="com.test.springmvc"></context:component-scan>
<!-- 配置视图解析器,把handler返回值解析为实际视图 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/views/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<mvc:annotation-driven></mvc:annotation-driven>
<bean id="messageSource" class="org.springframework.context.support.ResourceBundleMessageSource">
<property name="basename" value="i18n"></property>
</bean>
</beans>
8、Info.java
package com.test.springmvc.bean;
import java.util.Date;
import org.hibernate.validator.constraints.Email;
import org.hibernate.validator.constraints.NotEmpty;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.format.annotation.NumberFormat;
import org.springframework.stereotype.Repository;
@Repository
public class Info {
@NumberFormat(pattern="#.#")
double infoNumber;
@DateTimeFormat(pattern="yyyy-MM-dd")
Date infoDate;
@NotEmpty
String notEmptyData;
@Email
String email;
public Info(){}
public Info(double infoNumber, Date infoDate, String notEmptyData, String email) {
super();
this.infoNumber = infoNumber;
this.infoDate = infoDate;
this.notEmptyData = notEmptyData;
this.email = email;
}
public double getInfoNumber() {
return infoNumber;
}
public void setInfoNumber(double infoNumber) {
this.infoNumber = infoNumber;
}
public Date getInfoDate() {
return infoDate;
}
public void setInfoDate(Date infoDate) {
this.infoDate = infoDate;
}
public String getNotEmptyData() {
return notEmptyData;
}
public void setNotEmptyData(String notEmptyData) {
this.notEmptyData = notEmptyData;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
9、InfoDao.java
package com.test.springmvc.dao;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import org.springframework.stereotype.Repository;
import com.test.springmvc.bean.Info;
@Repository
public class InfoDao {
private static Map<Integer, Info>map = null;
static{
map=new HashMap<Integer,Info>();
map.put(1, new Info(12345.6,new Date(),"not_empty1","[email protected]"));
map.put(2, new Info(23456.7,new Date(),"not_empty2","[email protected]"));
map.put(3, new Info(34567.8,new Date(),"not_empty3","[email protected]"));
map.put(4, new Info(45678.9,new Date(),"not_empty4","[email protected]"));
}
public Collection<Info> getAll(){
return map.values();
}
public boolean add(Info info){
map.put(map.size()+1, info);
return true;
}
}
10、Handler.java
package com.test.springmvc.handlers;
import java.util.Collection;
import java.util.Map;
import javax.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.validation.Errors;
import org.springframework.validation.FieldError;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.test.springmvc.bean.Info;
import com.test.springmvc.dao.InfoDao;
@Controller
public class Handler {
@Autowired
public InfoDao infoDao;
@RequestMapping("/testJson")
@ResponseBody
public Collection<Info> testJson(){
return infoDao.getAll();
}
@RequestMapping("/list")
public String show(Map<String, Object>map){
map.put("infos", infoDao.getAll());
return "list";
}
@RequestMapping(value="/emp",method=RequestMethod.GET)
public String addPage(Map<String,Object>map){
map.put("info", new Info());
return "edit";
}
//这里错误信息的类型可以是BindingResult或者Errors
//需代码中需要注意的是,@Valid是必须的,只有加上@Valid,才会对传过来的参数 User类进行验证,后面必须紧跟着Errors result, 其中result是用来存放验证错误信息的,它们之间是不能有任务参数的,是成对出现的,有多少个@Valid,就必须有多少个Errors result。
@RequestMapping(value="/emp",method=RequestMethod.POST)
public String save(@Valid Info info, Errors result,Map<String, Object>map){
System.out.println("info:"+info.toString());
if(result.getErrorCount() > 0){
System.out.println("出错了!");
for(FieldError error:result.getFieldErrors()){
System.out.println(error.getField() + ":" + error.getDefaultMessage());
}
//若验证出错, 则转向定制的页面
map.put("info", info);
return "edit";
}else{
System.out.println("插入数据");
System.out.println(info.toString());
infoDao.add(info);
}
return "redirect:/list";
}
}
11、index.jsp
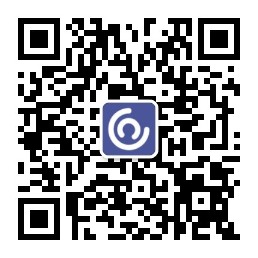
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="js/jquery-1.4.2.min.js"></script>
<script>
$(function(){
$.ajax({
type : 'post',
url : this.href,
async: false,
data:{},
dataType: 'json',
success : function(data) {
for(var i=0;i<data.length;i++){
var id = data[i].id;
var name = data[i].lastName;
}
}
});
})
</script>
</head>
<body>
<a href="list">list data</a>
<br><br>
<a href="testJson" id="testJson">test json</a>
<br><br>
1、数据格式化<br>
步骤:<br>
①创建bean并且用@Repository修饰bean类<br>
②在bean中对需要格式化的参数使用@NumberFormat或@DateTimeFormat修饰<br>
③编写对应的dao<br>
④编写handler<br>
⑤在spring配置文件中配置mvc:annotation-driven标签<br>
注意:handler中接收form表单的方法的参数不用@RequestParam修饰<br>
2、数据校验<br>
步骤:<br>
①加入jar,其中classmate和jboss-logging和validation-api是必须要加的,不然会报一下NoClassDefFoundError的错<br>
  classmate-1.3.4.jar<br>
  hibernate-validator-5.4.2.Final.jar<br>
  hibernate-validator-annotation-processor-5.4.2.Final.jar<br>
  hibernate-validator-cdi-5.4.2.Final.jar<br>
  jboss-logging-3.3.2.Final.jar<br>
  validation-api-2.0.1.Final.jar<br>
②在spring配置文件中配置mvc:annotation-driven标签<br>
③创建bean并且用@Repository修饰bean类<br>
④编写对应的dao<br>
⑤编写handler,并且在处理请求的方法的参数前面使用@Valid修饰<br>
⑥在bean中对需要验证的参数使用验证标签修饰,如:@Email/@NotEmpty<br>
注意:如果加入jar之后,类报错The type javax.validation.Payload cannot be resolved,则需要添加validation-api-x.x.x.Final.jar<br>
3、错误提示<br>
步骤:<br>
①完成数据校验的代码<br>
②在需要显示错误提示的form里面配置modelAttribute<br>
③在需要显示错误提示的form里面添加form:errors标签(path参数值如果是"*",则代表显示所有错误信息,值是参数名,则只显示对应参数名的错误信息)<br>
4、错误信息国际化<br>
步骤:<br>
①创建i18n文件<br>
②在springmvc配置文件中配置国际化资源<br>
注意:<br>
在国际化资源文件中@NumberFormat和@DateTimeFormat修饰的参数需要使用:typeMismatch.bean类名(首字母小写).参数名, 如:typeMismatch.info.infoDate=xxx<br>
在国际化资源文件中数据校验标签修饰的参数需要使用:标签名.bean类名(首字母小写).参数名, 如:NotEmpty.info.notEmptyData=xxx<br>
5、返回json<br>
步骤:<br>
①添加jar包<br>
  jackson-annotations-2.9.6.jar<br>
  jackson-core-2.9.6.jar<br>
  jackson-databind-2.9.6.jar<br>
②在handler里面写处理方法,并且返回数据(集合类型的数据)<br>
③页面加载jquery接收数据<br>
注意:<br>
①返回的结果会直接输出到页面上,所以可以直接用来作为返回json数据的接口<br>
②handler中被调用的方法需要用@ResponseBody修饰<br>
</body>
</html>
12、list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Info list</title>
</head>
<body>
<a href="emp">Add info</a>
<br><br>
<c:if test="${empty requestScope.infos}">
no data
</c:if>
<c:if test="${!empty requestScope.infos}">
<table border="1" cellpadding="10" cellspacing="0">
<tr>
<td>infoNumber</td>
<td>infoDate</td>
<td>notEmptyData</td>
<td>email</td>
</tr>
<c:forEach items="${requestScope.infos}" var="infoData">
<tr>
<td>${infoData.infoNumber}</td>
<td>${infoData.infoDate}</td>
<td>${infoData.notEmptyData}</td>
<td>${infoData.email}</td>
</tr>
</c:forEach>
</table>
</c:if>
</body>
</html>
13、edit.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Add Info</title>
</head>
<body>
<!-- 这里的modelAttribute一定要是bean的首字母小写名,不然会导致form:errors读不到字段名而不显示 -->
<form:form method="POST" action="emp" modelAttribute="info">
infoNumber:<form:input path="infoNumber"/><form:errors path="infoNumber"></form:errors><br>
infoDate:<form:input path="infoDate"/><form:errors path="infoDate"></form:errors><br>
notEmptyData:<form:input path="notEmptyData"/><form:errors path="notEmptyData"></form:errors><br>
email:<form:input path="email"/><form:errors path="email"></form:errors><br>
<br>
<input type="submit" value="submit">
</form:form>
</body>
</html>
14、i18n.properties
typeMismatch.info.infoDate=\u65F6\u95F4\u683C\u5F0F\u4E0D\u6B63\u786E
NotEmpty.info.notEmptyData=\u8FD9\u4E2A\u6570\u636E\u4E0D\u80FD\u4E3A\u7A7A
Email.info.email=\u8FD9\u4E2A\u6570\u636E\u9700\u8981\u662F\u90AE\u7BB1\u5730\u5740
15、项目目录
16、demo
https://download.csdn.net/download/qq_22778717/10601152