一、JPA
1. 概念:JPA顾名思义就是Java Persistence API的意思,是JDK 5.0注解或XML描述对象-关系表的映射关系,并将运行期的实体对象持久化到数据库中。
2.为什么 使用JPA:
JPA 是 JCP 组织发布的 Java EE 标准之一,因此任何声称符合 JPA 标准的框架都遵循同样的架构,提供相同的访问API,这保证了基于JPA开发的企业应用能够经过少量的修改就能够在不同的JPA框架下运行。
a.容器级特性的支持
JPA框架中支持大数据集、事务、并发等容器级事务,这使得 JPA 超越了简单持久化框架的局限,在企业应用发挥更大的作用。
b.简单方便
JPA的主要目标之一就是提供更加简单的编程模型:在JPA框架下创建实体和创建Java 类一样简单,没有任何的约束和限制,只需要使用 javax.persistence.Entity进行注释,JPA的框架和接口也都非常简单,没有太多特别的规则和设计模式的要求,开发者可以很容易的掌握。JPA基于非侵入式原则设计,因此可以很容易的和其它框架或者容器集成。
c.查询能力
JPA的查询语言是面向对象而非面向数据库的,它以面向对象的自然语法构造查询语句,可以看成是Hibernate HQL的等价物。JPA定义了独特的JPQL(Java Persistence Query Language),JPQL是EJB QL的一种扩展,它是针对实体的一种查询语言,操作对象是实体,而不是关系数据库的表,而且能够支持批量更新和修改、JOIN、GROUP BY、HAVING 等通常只有 SQL 才能够提供的高级查询特性,甚至还能够支持子查询。
d.高级特性
JPA 中能够支持面向对象的高级特性,如类之间的继承、多态和类之间的复杂关系,这样的支持能够让开发者最大限度的使用面向对象的模型设计企业应用,而不需要自行处理这些特性在关系数据库的持久化。
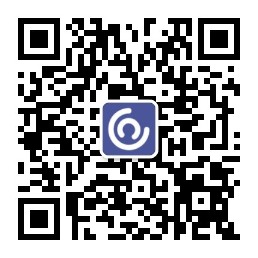
二、SpringBoot整合JPA
1.工程目录
这里有个小坑,之前搞了半天发现自动生成不了实体类对应的表后来发现是包名的原因,这里要注意SpringbootJpaDemoApplication所在的包与entity所在包之间的关系,SpringbootJpaDemoApplication在com.example.demo中那么我们的实体类User就得在com.example.demo.Entity下,这样才能成功对应生成实体类的表
2.配置pom:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>springboot-jpa-demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>springboot-jpa-demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.4.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- jpa依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- mysql依赖 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- 热部署 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<!-- optional=true, 依赖不会传递, 该项目依赖devtools; 之后依赖boot项目的项目如果想要使用devtools, 需要重新引入 -->
<optional>true</optional>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3.配置application.yml及application-test.yml
application.yml:
spring:
profiles:
active: test
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=true&serverTimezone=UTC
username: root
password: 123
jpa:
database: MYSQL
hibernate:
ddl-auto: update
show-sql: true
jackson:
date-format: yyyy-MM-dd HH:mm:ss
time-zone: UTC
application-test.yml:
spring:
devtools:
restart:
enabled: true
additional-paths: src/main/java
我们的实体User类 :
package com.example.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="t_user")
public class User {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)//自增
private Integer id;
private String name;
private String passWord;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassWord() {
return passWord;
}
public void setPassWord(String passWord) {
this.passWord = passWord;
}
}
到这里,我们启动项目,然后就会自动在数据库中生成对应的t_user表
4.创建controller,service,dto做一些增删改查的操作
a.controller:
package com.example.demo.controller;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.example.demo.entity.User;
import com.example.demo.service.IUserService;
@RestController
public class UserController {
@Autowired
IUserService userService;
@GetMapping(value="/getUserById")
public Optional<User> findUserById(@RequestParam("Id") Integer id){
return userService.findUserById(id);
}
@GetMapping("/all")
public List<User> findAll(){
return userService.findAll();
}
@PostMapping("/update")
public User updateUser(@RequestParam("Id") Integer id,@RequestParam("name") String name,@RequestParam("passWord") String passWord) {
return userService.updateUserById(id, name, passWord);
}
@PutMapping("/insert")
public User insertUser(@RequestParam("name") String name,@RequestParam("passWord") String passWord) {
return userService.insertUser(name, passWord);
}
@DeleteMapping("/delete")
public void deleteUser(@RequestParam("Id") Integer id) {
userService.deleteUserById(id);
}
}
b.service:
package com.example.demo.service;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.demo.dto.IUserDto;
import com.example.demo.entity.User;
@Service
public class UserServiceImpl implements IUserService{
@Autowired
IUserDto iUserDto;
@Override
public Optional<User> findUserById(Integer id) {
return iUserDto.findById(id);
}
@Override
public User updateUserById(Integer id,String name, String passWord) {
User user = new User();
user.setId(id);
user.setName(name);
user.setPassWord(passWord);
return iUserDto.save(user);
}
@Override
public User insertUser(String name, String passWord) {
User user = new User();
user.setName(name);
user.setPassWord(passWord);
return iUserDto.save(user);
}
@Override
public void deleteUserById(Integer id) {
iUserDto.deleteById(id);
}
@Override
public List<User> findAll() {
return iUserDto.findAll();
}
}
c.dto:
package com.example.demo.dto;
import java.util.List;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.demo.entity.User;
public interface IUserDto extends JpaRepository<User,Integer>{//默认传的是ID为条件,如果需要其他条件来进行CRDU操作可通过增加方法来实现
public List<User> findByName(String name);//方法名不能乱写,要按你需要的条件来写 findBy你的字段名
}
5.测试:
这里采用火狐的RESTClient
a.put用法
b.delete用法
c.post用法
d.Get用法