[1,1,2,3,5,8,13,21......] 第n项的值:
public static int f(int n) throws Exception{
if(n==1 || n ==2){
return 1;
}else{
return f(n-1)+f(n-2);
}
}
2.求水仙花
public class ShuiXianHuaShu {
public static void main(String[] args) {
int x = 0; //定义水仙花数的个数
for(int i=100;i<=999;i++){
int b = i/100; //取得百位数
int s = (i-100*b)/10; //取得十位数
int g = (i-s*10-b*100); //取得个位数
if(i==g*g*g+s*s*s+b*b*b){
x++; //每次符合水仙花数条件,则x+1;
System.out.print(i+" "); //输出符合条件的数
}
}System.out.println(); //换行
System.out.println("水仙花数总共有"+x+"个"); //输出水仙花数的总数
}
}
linux 查看应用日志的集中常用命令:
tail -100f test.log 实时监控100行日志
head -n 10 test.log 查询日志文件中的头10行日志;
cat -n test.log |grep "debug" 查询关键字的日志
根据下表获得总分数大于150 的学生名单:
select st.stuName,sc.stuCode,sum(sc.score) as totalScore from t_student as st left join t_score as sc
on st.stuCode = sc.stuCode
group by sc.stuCode
having sum(sc.score) > 150
利用字符重复出现的次数,编写一个方法,实现基本的字符串压缩功能。比如,字符串“aabcccccaaa”经压缩会变成“a2b1c5a3”。若压缩后的字符串没有变短,则返回原先的字符串。
public class Zipper {
public String zipString(String iniString) {
String str=iniString;
if(str==null||str.isEmpty()){
return null;
}
StringBuffer mystr=new StringBuffer();
char last=str.charAt(0);
int count=1;
for(int i=1;i<str.length();i++){
if(str.charAt(i)==last){
count++;
}
else{
mystr.append(last);
mystr.append(count);
last=str.charAt(i);
count=1;
}
}
mystr.append(last);
mystr.append(count);
if(mystr.length() >= str.length()) {
return str;
}
else{
return mystr.toString();
}
}
}
public static void main(String[] args) {
Zipper z = new Zipper();
String aa = "aaacccd";
System.out.println("得到的数据是:"+z.zipString(aa));
}
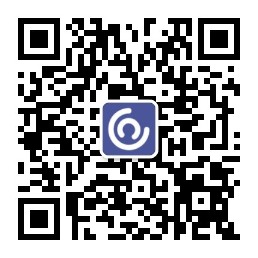
若MxN矩阵中某个元素为0, 则将其所在的行与列清零
public class setZeros {
public void setZero(int[][] matrix)
{
boolean[] row = new boolean[matrix.length];
boolean[] column = new boolean[matrix[0].length];
for (int i = 0; i < matrix.length; i++)
{
for (int j = 0; j < matrix[0].length; j++)
{
if (matrix[i][j] == 0)
{
row[i] = true;
column[j] = true;
}
}
}
for (int i = 0; i < matrix.length; i++)
{
for (int j = 0; j < matrix[0].length; j++)
{
if(row[i] || column[j])
{
matrix[i][j] = 0;
}
}
}
}
}
String name = map.get("name") != null ? map.get("name").toString() : "";
/**
* 判断传入的字符串是否是数字,这个很常用的用了能减少很多恶意的bug @Title: isNumber @param @param
* value @param @return 设定文件 @return boolean 返回类型 @author gdl @date
*/
public static boolean isNumber(String value) {
try {
Integer.parseInt(value);
return true;
} catch (NumberFormatException e) {
return false;
}
}
/**
* StringUtils工具类方法
* 获取一定长度的随机字符串,范围0-9,a-z
* @param length:指定字符串长度
* @return 一定长度的随机字符串
*/
public static String getRandomStringByLength(int length) {
String base = "abcdefghijklmnopqrstuvwxyz0123456789";
Random random = new Random();
StringBuffer sb = new StringBuffer();
for (int i = 0; i < length; i++) {
int number = random.nextInt(base.length());
sb.append(base.charAt(number));
}
return sb.toString();
}
求1+2+3+...+n的和,不能使用乘除、for、while、if、else、switch、case等关键字以及条件判断的语句:
public class Solution {
//方案一:
public int Sum_Solution(int n) {
return (int)(Math.pow(n, 2)+n)>>1;
}
//方案二:
public int Sum_Solution2(int n) {
int sum = n;
boolean ans = (n>0)&&((sum+=Sum_Solution(n-1))>0);
if(ans){
return sum;
}else{
return 0;
}
}
//方案三
public int Sum_Solution3(int n){
int sum = 0;
for (int i = 0; i <= 100; i++) {
sum +=i;
}
return sum;
}
}
@Test
public void te(){
Solution solution = new Solution();
System.out.println(solution.Sum_Solution(100));
System.out.println(solution.Sum_Solution2(100));
System.out.println(solution.Sum_Solution3(100));
}
在一个长度为n的数组里的所有数字都在0到n-1的范围内。 数组中某些数字是重复的,但不知道有几个数字是重复的。也不知道每个数字重复几次。
请找出数组中任意一个重复的数字。 例如,如果输入长度为7的数组{2,3,1,0,2,5,3},那么对应的输出是第一个重复的数字2。
public boolean Sum_Solution4(int numbers[], int length, int[] duplication) {
if (numbers == null || length <= 0) {
return false;
}
for (int i = 0; i < length; i++) {
if (numbers[i] < 0 || numbers[i] >= length)
return false;
}
for (int j = 0; j < length; j++) {
while (numbers[j] != j) {
if (numbers[j] == numbers[numbers[j]]) {//true说明有重复
duplication[0] = numbers[j];
return true;
}
int temp = numbers[j];
numbers[j] = numbers[temp];
numbers[temp] = temp;
}
}
return false;
}
Solution solution = new Solution();
int[] numbers={2,3,1,0,2,5,3};
int length=7;
int[] duplication=new int[1];
boolean bool = solution.Sum_Solution4(numbers, length, duplication);
System.out.println(bool + " "+ duplication[0]);
统计字符,空格,数字,其他字符的个数:
@Test
public void countLength(){
String aa = "aZ09 &";
int sLength = 0;
int NullLength = 0;
int numLength = 0;
int otherLength = 0;
for (int i = 0; i < aa.length() ; i++) {
char tem = aa.charAt(i);
if ((tem >='a' && tem <= 'z') || (tem >='A' && tem <='Z')){
sLength ++;
}else if(tem ==' '){
NullLength++;
}else if((tem >='0' && tem <= '9')){
numLength ++;
}else{
otherLength ++;
}
}
System.out.println("String:"+sLength+"\nNullLength:"+NullLength+"\nnumLength:"+numLength+"\notherLength:"+otherLength);
}
获取不带后缀的名字/获取后缀名
public static String nameWithoutSuffix(String name) { if (StringUtils.isEmpty(name)) { return null; } int index = name.lastIndexOf("."); if (index > 0) { return name.substring(0, index); } return null; } public static String suffix(String name) { if (StringUtils.isEmpty(name)) { return null; } int index = name.lastIndexOf("."); if (index > 0) { return name.substring(index, name.length()); } return null; }