本文主要演示C程序调用Lua脚本的基本实现方法,并解决实践过程中遇到的问题。
特别说明,由于lua-5.1.x版本与后续版本的接口函数的差异较大,为保证本文提供小程序可直接在你本地调试通过,请安装5.2以及后续版本的Lua开源软件。
系统环境和Lua版本要求请参考(内含Lua开源软件下载和安装方法):
http://blog.csdn.net/hanlizhong85/article/details/74058235
1、C程序调用Lua脚本
1.1、C程序实现(add.c):
#include <stdio.h>
#include "lua.h"
#include "lualib.h"
#include "lauxlib.h"
/*the lua interpreter*/
lua_State *L = NULL;
int lua_add(int x, int y)
{
int sum;
/*the function name in lua */
lua_getglobal(L, "add");
/*the first & second argument*/
lua_pushnumber(L, x);
lua_pushnumber(L, y);
/*call the function with 2 arguments, return 1 result.*/
lua_call(L, 2, 1); //调用Lua中的add函数
//lua_pcall(L, 2, 1, 0); //可替换上面的lua_call语句
/*get the result.*/
sum = (int)lua_tonumber(L, -1);
/*cleanup the return*/
lua_pop(L, 1);
return sum;
}
int main(int argc, char *argv[])
{
int sum;
//L = lua_open(); //lua_open是5.0时代的产物,5.1是luaL_newstate的宏,5.2里面已经没有了
L = luaL_newstate(); //no lua_open() after 5.2
/*load Lua base libraries*/
luaL_openlibs(L);
/*load the script*/
luaL_dofile(L, "add.lua");
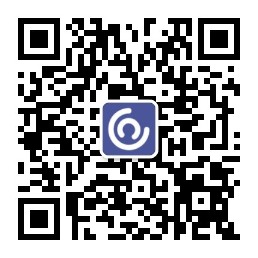
/*call the add function*/
sum = lua_add(2, 20);
/*print the result*/
printf("The sum is %u\n",sum);
/*cleanup Lua*/
lua_close(L);
return 0;
}
1.2、Lua脚本实现(add.lua):
--add two numbers
function add(x, y)
print("calc: "..x.."+"..y.."=")
return x + y
end
1.3、把C文件编译成可执行程序:
[root@HLZ c_lua]# gcc -o add add.c -I /usr/local/include/ -llua -ldl -lm
[root@HLZ c_lua]# ls
add add.c add.lua readme.txt
其中,gcc编译选项-I /usr/local/include用于指定add.c中所使用lua接口函数的声明头文件的位置;链接选项-llua -ldl –lm 表示可执行程序的生成依赖lua,dl和math库。
1.4、执行C程序:
[root@HLZ c_lua]# ./add
calc: 2.0+20.0=
The sum is 22
[root@HLZ c_lua]#
2、常见错误及解决方法:
2.1、C程序add.c的main函数中,Lua脚本上下文 lua_State *L 通过lua_open而非luaL_newstate获取。
若L通过lua_open获取,则链接时会报符号未定义错误:
[root@HLZ c_lua]# gcc -o add add.c -I /usr/local/include/ -llua -ldl -lm
add.c: In function ‘main’:
add.c:34: warning: assignment makes pointer from integer without a cast
/tmp/ccP8ULEE.o: In function `main':
add.c:(.text+0xc7): undefined reference to `lua_open'
collect2: ld returned 1 exit status
上述报错与Lua的版本有关,因为lua_open是5.0版本时代的产物,5.1已经只是luaL_newstate函数的宏定义,5.2版本里面已经没有了,即lua_open只在5.1之前的版本中存在,5.2版本之后务必使用luaL_newstate。