水题使我快乐_(:зゝ∠)_
半个暑假过去了,是时候刷点水题恢复一下了,哈哈哈。
题目地址
总结:1~9,12~13水题;10大数乘法,11希尔排序或归并排序等(除快速排序)
6-1
void PrintN(int N)
{
int x=1;
while(x<=N)
printf("%d\n",x++);
}
6-2
double f( int n, double a[], double x )
{
double ans = 0.0;
int z = 0;
double y = 1.0;
for(;z<=n;z++,y*=x)
ans += a[z]*y;
return ans;
}
6-3
int Sum ( int List[], int N )
{
int x=0;
int sum=0;
while(x<N)
sum+=List[x++];
return sum;
}
6-4
ElementType Average( ElementType S[], int N )
{
ElementType sum = 0;
int x=N;
while(N)
sum+=S[--N];
return sum/x;
}
6-5
ElementType Max( ElementType S[], int N )
{
ElementType M=S[N-1];
#define Max(a,b) (a>b?a:b)
while(N--)
M=Max(S[N],M);
#undef Max(a,b)
return M;
}
6-6
int FactorialSum( List L )
{
int sum=0;
while(L!=NULL)
{
int x,y;
for(x=1,y=1;x<=L->Data;x++)
y*=x;
sum+=y;
L=L->Next;
}
return sum;
}
6-7
int IsTheNumber ( const int N )
{
#include<math.h>
double sq=sqrt(N);
if(sq-(int)sq)
return 0;
else
{
int num=N;
int dig[10]={0};
while(num)
{
int x=num%10;
num/=10;
dig[x]++;
if(dig[x]>1)
return 1;
}
}
return 0;
}
6-8
扫描二维码关注公众号,回复:
2598008 查看本文章
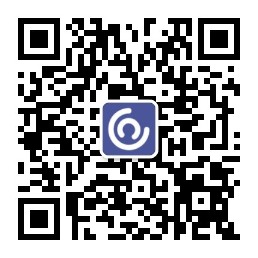
int Factorial( const int N )
{
if(!N)
return 1;
if(N<0)
return 0;
int x=N,y=N;
while(--y)
x*=y;
return x;
}
6-9
int Count_Digit ( const int N, const int D )
{
if(!N&&!D)
return 1;
int num = abs(N),cnt = 0;
while(num)
{
int x = num%10;
num/=10;
if(x==D)
cnt++;
}
return cnt;
}
特殊情况:N和D都是0的时候,输出应为1。
6-10
void Big_multiplication(int* a,int* b,int *result);
void Print_Factorial ( const int N )
{
if(!N||N==1)
{
printf("1\n");
return;
}
if(N<0)
{
printf("Invalid input\n");
return;
}
int num1[10]={1,1};
int num2[3000]={1,2};
int mul[3000];
Big_multiplication(num1,num2,mul);
int i;
for(i=3;i<=N;i++)
{
int x=i,y=1,j;
while(x)
{
num1[y]=x%10;
x/=10;
y++;
}
num1[0]=y-1;
for(j=0;j<=mul[0];j++)
num2[j]=mul[j];
Big_multiplication(num1,num2,mul);
}
for(i=mul[0];i>=1;i--)
printf("%d",mul[i]);
printf("\n");
return;
}
void Big_multiplication(int* a,int* b,int *result)
{
for(int i=0;i<3000;i++)
result[i]=0;
int i=1;
while(i<=b[0])
{
int j=1;
while(j<=a[0])
{
result[i+j-1]+=a[j]*b[i];
j++;
}
i++;
}
i=1;
while(result[i]||i<=b[0]+a[0]-1)
{
result[i+1]+=result[i]/10;
result[i]=result[i]%10;
i++;
}
result[0]=i-1;
}
这是个大数乘法题,1000的阶乘位数达到两千多位,必须用数组存储结果。
这里用的是模拟乘法运算的方法,数组【0】存放此数组数的总位数,先不管是否进位,计算出结果每一位,最后从最低位往高位逐位进位处理。
注意特殊情况:负数时输出Invalid input,0时输出1。
6-11
这道题用冒泡、选择和插入排序都是不行的,最后一个测试点时间超限。于是测试了快速排序、希尔排序和归并排序。
期望很高的快速排序竟然也超时了,然而希尔排序和归并排序都成功通过测试。我想最后一个测试点应该卡的就是快速排序的最坏情况。
ElementType Median( ElementType A[], int N )
{
int i,j,x;
ElementType temp;
for(x=N/2;x>0;x/=2)
{
for(i=x;i<N;i++)
{
temp=A[i];
for(j=i;j>=x;j-=x)
if(temp<A[j-x])
A[j]=A[j-x];
else
break;
A[j]=temp;
}
}
return A[N/2];
}
采用希尔排序,最后一个测试点能通过(36ms左右)。
#include<stdlib.h>
void merge(ElementType A[],ElementType temp[],int lops,int rops,int rightend)
{
int i,leftend,sum,tempos;
leftend=rops-1;
tempos=lops;
sum=rightend-lops+1;
while(lops<=leftend&&rops<=rightend)
if(A[lops]<=A[rops])
temp[tempos++]=A[lops++];
else
temp[tempos++]=A[rops++];
while(lops<=leftend)
temp[tempos++]=A[lops++];
while(rops<=rightend)
temp[tempos++]=A[rops++];
for(i=0;i<sum;i++,rightend--)
A[rightend]=temp[rightend];
}
void mersort(ElementType A[],ElementType temp[],int left,int right)
{
if(left<right)
{
int x=(left+right)/2;
mersort(A,temp,left,x);
mersort(A,temp,x+1,right);
merge(A,temp,left,x+1,right);
}
}
ElementType Median( ElementType A[], int N )
{
ElementType* temp;
temp=(ElementType*)malloc(N*sizeof(ElementType));
mersort(A,temp,0,N-1);
free(temp);
return A[N/2];
}
采用归并排序,最后一个测试点能通过(39ms左右)。
6-12
int even( int n )
{
if(n%2)
return 0;
return 1;
}
6-13
int Search_Bin(SSTable T, KeyType k)
{
int left,right;
left=1,right=T.length;
while(left<right)
{
KeyType x = T.R[(left+right)/2].key;
if(x==k)
return (left+right)/2;
if(x>k)
right=(left+right)/2-1;
else
left=(left+right)/2+1;
}
return 0;
}
简单的二分查找