管道基本概念:
管道是Unix中最古老的进程间通信形式
我们把从一个进程连接到另一个进程的一个数据流称为一个“管道”
管道的本质:固定大小的内核缓冲区
管道限制:
管道是半双工的,数据只能向一个方向流动;需要双方通信时,需要建立起两个管道
只能用于具有共同祖先的进程(具有亲缘关系的进程)之间进行通信,通常,一个管道由一个进程创建,然后该进程调用fork,此后,父子进程之间就可以应用该管道
匿名管道pipe:
原型:int pipe(int fd[2])
功能:创建一个无名管道
参数:
扫描二维码关注公众号,回复:
2565708 查看本文章
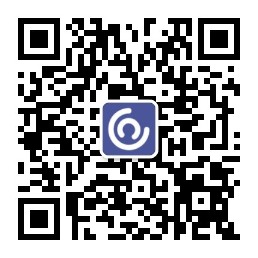
fd:文件描述符数组,其中,fd[0]表示读端,fd[1]表示写端
返回值:成功返回0,失败返回错误码
管道创建后示意图:
管道的读写规则如下:
管道读写示例:
1 #include <unistd.h> 2 #include <sys/stat.h> 3 #include <sys/wait.h> 4 #include <sys/types.h> 5 #include <fcntl.h> 6 7 #include <stdlib.h> 8 #include <stdio.h> 9 #include <errno.h> 10 #include <string.h> 11 #include <signal.h> 12 13 #define ERR_EXIT(m) \ 14 do \ 15 { \ 16 perror(m); \ 17 exit(EXIT_FAILURE); \ 18 } while(0) 19 20 21 //测试 默认情况下,管道的读写 22 int main41(void ) 23 { 24 int pipefd[2]; 25 pid_t pid; 26 if (pipe(pipefd) == -1 ) 27 { 28 printf("pipe() err..\n"); 29 return -1; 30 } 31 pid = fork(); 32 if (pid == -1) 33 { 34 printf("fork err..\n"); 35 return -1; 36 } 37 38 if (pid == 0) 39 { 40 printf("子进程延迟3秒后,再写。。\n"); 41 sleep(3); 42 close(pipefd[0]); 43 write(pipefd[1], "hello hello....", 6); 44 close(pipefd[1]); 45 printf("child .....quit\n"); 46 exit(0); 47 } 48 else if (pid > 0 ) 49 { 50 int len = 0; 51 char buf[100] = {0}; 52 close(pipefd[1]); 53 54 55 printf("测试非阻塞情况下,begin read ...\n"); 56 len = -1; 57 while (len < 0) 58 { 59 len = read(pipefd[0], buf, 100); 60 if (len == -1) 61 { 62 sleep(1); 63 //close(pipefd[0]); 64 perror("\nread err:"); 65 //exit(0); 66 } 67 } 68 69 printf("len:%d, buf:%s \n", len , buf); 70 close(pipefd[0]); 71 } 72 73 wait(NULL); 74 printf("parent ..quit\n"); 75 return 0; 76 77 } 78 79 80 //文件状态设置成阻塞非阻塞 81 int main(void ) 82 { 83 int pipefd[2]; 84 pid_t pid; 85 if (pipe(pipefd) == -1 ) 86 { 87 printf("pipe() err..\n"); 88 return -1; 89 } 90 pid = fork(); 91 if (pid == -1) 92 { 93 printf("fork err..\n"); 94 return -1; 95 } 96 97 if (pid == 0) 98 { 99 sleep(3); 100 close(pipefd[0]); 101 write(pipefd[1], "hello hello....", 6); 102 close(pipefd[1]); 103 printf("child .....quit\n"); 104 } 105 else if (pid > 0 ) 106 { 107 int len = 0; 108 char buf[100] = {0}; 109 close(pipefd[1]); 110 111 /* 112 int fcntl(int fd, int cmd); 113 int fcntl(int fd, int cmd, long arg); 114 int fcntl(int fd, int cmd, struct flock *lock); 115 F_GETFL F_SETFL O_NONBLOCK 116 //注意不要设置错误成F_GETFD F_SETFD 117 118 119 */ 120 int flags = fcntl(pipefd[0], F_GETFL); 121 flags = flags | O_NONBLOCK; 122 int ret = fcntl(pipefd[0], F_SETFL, flags); 123 if (ret == -1) 124 { 125 printf("fcntl err.\n"); 126 exit(0); 127 } 128 129 //把pipefd[0]文件描述符修改成非阻塞 man fcntl 130 printf("begin read ...\n"); 131 len = -1; 132 while (len < 0) 133 { 134 len = read(pipefd[0], buf, 100); 135 if (len == -1) 136 { 137 sleep(1); 138 //close(pipefd[0]); 139 perror("read err.\n"); 140 //exit(0); 141 } 142 } 143 144 printf("len:%d, buf:%s \n", len , buf); 145 close(pipefd[0]); 146 } 147 148 wait(NULL); 149 printf("parent ..quit\n"); 150 return 0; 151 152 }