链接:https://www.nowcoder.com/acm/contest/141/C
来源:牛客网
Shuffle Cards
时间限制:C/C++ 1秒,其他语言2秒
空间限制:C/C++ 262144K,其他语言524288K
Special Judge, 64bit IO Format: %lld
题目描述
Eddy likes to play cards game since there are always lots of randomness in the game. For most of the cards game, the very first step in the game is shuffling the cards. And, mostly the randomness in the game is from this step. However, Eddy doubts that if the shuffling is not done well, the order of the cards is predictable!
To prove that, Eddy wants to shuffle cards and tries to predict the final order of the cards. Actually, Eddy knows only one way to shuffle cards that is taking some middle consecutive cards and put them on the top of rest. When shuffling cards, Eddy just keeps repeating this procedure. After several rounds, Eddy has lost the track of the order of cards and believes that the assumption he made is wrong. As Eddy’s friend, you are watching him doing such foolish thing and easily memorizes all the moves he done. Now, you are going to tell Eddy the final order of cards as a magic to surprise him.
Eddy has showed you at first that the cards are number from 1 to N from top to bottom.
For example, there are 5 cards and Eddy has done 1 shuffling. He takes out 2-nd card from top to 4-th card from top(indexed from 1) and put them on the top of rest cards. Then, the final order of cards from top will be [2,3,4,1,5].
输入描述:
The first line contains two space-separated integer N, M indicating the number of cards and the number of shuffling Eddy has done.
Each of following M lines contains two space-separated integer pi, si indicating that Eddy takes pi-th card from top to (pi+si-1)-th card from top(indexed from 1) and put them on the top of rest cards.
1 ≤ N, M ≤ 105
1 ≤ pi ≤ N
1 ≤ si ≤ N-pi+1
输出描述:
Output one line contains N space-separated integers indicating the final order of the cards from top to bottom.
示例1
输入
5 1
2 3
输出
2 3 4 1 5
示例2
输入
5 2
2 3
2 3
输出
3 4 1 2 5
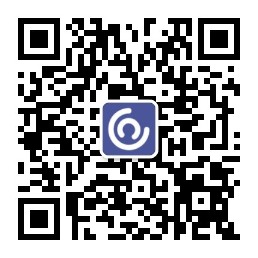
示例3
输入
5 3
2 3
1 4
2 4
输出
3 4 1 5 2
题意: 有一个1-n的序列,然后m次操作,每次将区间
移到序列最前端,最后输出m次操作之后的序列。
rope大法好.
代码
#include <bits/stdc++.h>
using namespace std;
#define fi first
#define se second
#define bug(x) cout<<"@@ "<<x<<"\n"
typedef long long ll;
typedef unsigned long long ull;
typedef pair<int, int> pii;
const int N = (int) 1e5 + 11;
const int M = (int) 1e6 + 11;
const int INF = (int)0x3f3f3f3f;
const int MOD = (int)1e9 + 7;
#include <ext/rope>
using namespace __gnu_cxx;
rope<int> L;
void Output(rope<int> a){
for(int i = 0; i < (int)a.size(); ++i){
printf("%d ", a[i]);
}
puts("");
}
int main(){
#ifndef ONLINE_JUDGE
freopen("in.txt", "r", stdin);
freopen("out.txt", "w", stdout);
#endif
int n, m; scanf("%d%d", &n, &m);
L.push_back(0);
for(int i = 1; i <= n; i++){
L.push_back(i);
}
while(m--){
int a, b; scanf("%d%d", &a, &b);
L = L.substr(0, 1) + L.substr(a, b) + L.substr(1, a - 1) + L.substr(b + a, n - a - b + 1);
}
for(int i = 1; i <= n; i++){
printf("%d ", L[i]);
}
return 0;
}
链接:https://www.nowcoder.com/acm/contest/141/E
来源:牛客网
Sort String
时间限制:C/C++ 1秒,其他语言2秒
空间限制:C/C++ 262144K,其他语言524288K
Special Judge, 64bit IO Format: %lld
题目描述
Eddy likes to play with string which is a sequence of characters. One day, Eddy has played with a string S for a long time and wonders how could make it more enjoyable. Eddy comes up with following procedure:
- For each i in [0,|S|-1], let Si be the substring of S starting from i-th character to the end followed by the substring of first i characters of S. Index of string starts from 0.
- Group up all the Si. Si and Sj will be the same group if and only if Si=Sj.
- For each group, let Lj be the list of index i in non-decreasing order of Si in this group.
- Sort all the Lj by lexicographical order.
Eddy can’t find any efficient way to compute the final result. As one of his best friend, you come to help him compute the answer!
输入描述:
Input contains only one line consisting of a string S.
1≤ |S|≤ 106
S only contains lowercase English letters(i.e. ).
输出描述:
First, output one line containing an integer K indicating the number of lists.
For each following K lines, output each list in lexicographical order.
For each list, output its length followed by the indexes in it separated by a single space.
示例1
输入
abab
输出
2
2 0 2
2 1 3
示例2
输入
deadbeef
输出
8
1 0
1 1
1 2
1 3
1 4
1 5
1 6
1 7
hash
代码
#pragma GCC optimize ("O2")
#include <bits/stdc++.h>
using namespace std;
#define bug(x) cout<<"@@@"<<x<<"@@@\n";
typedef unsigned long long ull;
typedef long long ll;
typedef pair<int, int> pii;
const int N = (int)2000000 + 11;
const int M = (int) 1e6 + 11;
const int MOD = (int) 1e9 + 7;
const int INF = (int) 0x3f3f3f3f;
const int seed = (int) 233;
unordered_map<ull, int> mp ;
unordered_map<ull, int>:: iterator it;
vector<int>ve[N]; int sz = 0;
struct Node{
int a; int id;
Node(){}
Node(int _a, int _id){
a = _a; id = _id;
}
}node[N];
bool cmp(Node a, Node b){
return a.a < b.a;
}
ull H[N * 2], X[N * 2];
void init(string s){
int len = s.size();
H[len]=0;
for(int i=len-1;i>=0;i--){
H[i]=H[i+1]*seed+s[i]-'a';
}
X[0]=1;
for(int i=1;i<=len;i++){
X[i]=X[i-1]*seed;
}
}
inline ull getHash(int a,int mid){
return H[a]-H[a+mid]*X[mid];
}
int main() {
string s; cin>>s; int n = s.size();
s+=s; int m = s.size();
init(s);
mp[getHash(0, n)] = ++sz;
ve[sz].push_back(0);
for(int i = n; i < m - 1; i++){
ull has = getHash(i - n + 1, n);
if(!mp[has]) mp[has] = ++sz;
ve[mp[has]].push_back(i - n + 1);
}
printf("%d\n", mp.size());
int cnt = 0;
for(it = mp.begin(); it != mp.end(); it++)
node[cnt++] = Node(ve[it->second][0], it->second);
sort(node, node + cnt, cmp);
Node z;
for(int i = 0; i < cnt; i++){
z = node[i];
printf("%d ", ve[z.id].size());
int flag = 0; int zzx = ve[z.id].size();
for(int j = 0; j < zzx; j++){
if(flag ++) putchar(' ');
printf("%d", ve[z.id][j]);
}
puts("");
}
return 0;
}