本篇博客主要编写了链表的相关程序,主要内容如下:
一.相关叙述
1.将数据节点用Node来表示,Node类中包含数据部分data,指针部分*Next(用于指向下一个节点)。
2.整个链表的头节点*plist用于方便插入和链表的描述,不用于存储数据和链表的输出,整个链表从头结点的下一个节点开始,表示的位置为0。
3.在链表的相关操作中,例如:增加元素,遍历等,均是从头结点往下(头节点元素插入时,从头结点下一个元素往上操作)进行节点遍历以找到相应的节点位置,从而进行相关操作。
4.ps:可将Node节点的data部分扩充为相应的类,将符号“<<”,"=="等重载,
二.相关代码
2.1头结点的定义Node.h以及Node.cpp:
#ifndef Node_H_
#define Node_H_
class Node
{
public:
Node();
~Node();
int data;
Node *next;
void printNode();
};
#endif
#include"Node.h"
#include<iostream>
using namespace std;
void Node:: printNode()
{
cout << data << endl;
}
Node::Node()
{
data = 0;
next = NULL;
}
Node::~Node()
{}
2.2Mylist.h及list.cpp:
#ifndef LIST_H_
#define LIST_H_
#include"Node.h"
class List
{
public:
List();
~List();
void ClearList();
bool ListEmpty();
int ListLength();
bool GetElem(int i,Node *pNode);
int LocateElem(Node *pNode);
bool PriorElem(Node *CurrentNode,Node *pPreNode);
bool NextElem(Node *CurrentNode, Node *pNextNode);
void ListTraverse();
bool ListInsert(int i,Node *pNode);
bool ListDelete(int i,Node*pNode);
bool ListInsertHead(Node *pNode);
bool ListInsertTail(Node *pNode);
private:
Node *m_plist;
int m_isize;
int m_ilength;
};
#endif
#include"List.h"
#include<iostream>
using namespace std;
List::List()
{
m_plist = new Node;
m_plist->next = NULL;
m_plist->data = 0;
m_ilength = 0;
}
List::~List()//删除所有节点
{
ClearList();
delete m_plist;
m_plist = NULL;
}
void List::ClearList()//除第一个外的节点清空
{
Node *CurrentNode = m_plist->next;
while (CurrentNode != NULL)
{
Node *temp = CurrentNode->next;
delete CurrentNode;
CurrentNode=temp;
}
m_plist->next = NULL;
}
bool List::ListEmpty()
{
if (m_ilength == 0)
{
return true;
}
else
{
return false;
}
}
int List::ListLength()
{
return m_ilength;
}
bool List::GetElem(int i, Node *pNode)
{
Node *CurrentNode = m_plist;
for (int k = 0; k <= i; k++)
{
CurrentNode = CurrentNode->next;
}
pNode->data = CurrentNode->data;
return true;
}
int List::LocateElem(Node *pNode)
{
Node *CurrentNode = m_plist->next;// start form the node head's next one
int count = 0;
while (CurrentNode->next != NULL)
{
if (CurrentNode->data == pNode->data)
{
return count;
}
count++;
CurrentNode = CurrentNode->next;
}
return -1;//return -1 if nothing is found
}
bool List::PriorElem(Node *pCurrentNode, Node *pPreNode)
{
Node *newNode = m_plist;
Node *currentBefore = new Node;
while (newNode->next != NULL)
{
currentBefore = newNode;
newNode = newNode->next;
if (pCurrentNode->data == newNode->data)
{
if (newNode == m_plist)
{
return false;
}
pPreNode->data=currentBefore->data;
return true;
}
}
return false;
}
bool List::NextElem(Node *CurrentNode, Node *pNextNode)
{
Node *newNode = m_plist;
Node *currenttemp = new Node;
while (newNode->next != NULL)
{
currenttemp=newNode;
newNode = newNode->next;
if (CurrentNode->data == currenttemp->data)
{
if (currenttemp->next == NULL)
{
return false;
}
pNextNode->data = newNode->data;
return true;
}
//pNextNode->data = newNode->data;
//return true;
}
return false;
}
void List::ListTraverse()
{
Node*CurrentNode = m_plist;
while (CurrentNode->next != NULL)
{
CurrentNode=CurrentNode->next;
CurrentNode->printNode();
}
}
bool List::ListInsert(int i, Node *pNode)
{
Node *CurrentNode = m_plist;
for (int k = 0; k < i; k++)
{
CurrentNode = CurrentNode->next;
}
Node *NewNode = new Node;
NewNode->data = pNode->data;
NewNode->next = CurrentNode->next;
CurrentNode->next = NewNode;
m_ilength++;
return true;
}
bool List::ListDelete(int i, Node*pNode)
{
Node *CurrentNode = m_plist;
Node *CurrentBeforeNode = NULL;
for (int k = 0; k <=i; k++)
{
CurrentBeforeNode=CurrentNode;
CurrentNode = CurrentNode->next;
}
CurrentBeforeNode->next = CurrentNode->next;
pNode->data = CurrentNode->data;
delete CurrentNode;
CurrentNode = NULL;
m_ilength--;
return true;
}
bool List::ListInsertHead(Node *pNode)//从头结点之后开始插入
{
Node *newNode = new Node;
newNode->data=pNode->data;
newNode->next=m_plist->next;
m_plist->next = newNode;
m_ilength++;
return true;
}
bool List::ListInsertTail(Node *pNode)
{
Node *CurrentNode = m_plist;
while (CurrentNode->next!=NULL)
{
CurrentNode = CurrentNode->next;//找尾为节点,从为节点插入
}
Node *newNode = new Node;
newNode->data = pNode->data;
CurrentNode->next = newNode;
m_ilength++;
return true;
}
2.3测试阶段:
扫描二维码关注公众号,回复:
2492035 查看本文章
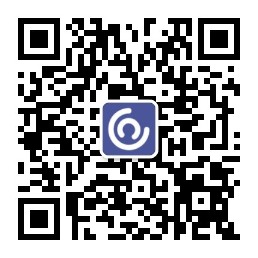
#include<iostream>
#include"List.h"
#include"Node.h"
using namespace std;
int main()
{
Node node1;
node1.data = 1;
Node node2;
node2.data = 2;
Node node3;
node3.data = 3;
Node node4;
node4.data = 4;
Node node5;
node5.data = 5;
Node node6;
Node node;
List *plist = new List();
plist->ListInsertHead(&node1);
plist->ListInsertHead(&node2);
plist->ListInsertHead(&node3);
plist->ListInsertHead(&node4);
plist->ListInsertHead(&node5);
plist->ListTraverse();
plist->PriorElem(&node3,&node);
cout << "node3's prior is:"<<node.data << endl;
plist->NextElem(&node3, &node);
cout << "node3's next is:"<<node.data << endl;
plist->GetElem(4, &node);
cout << "number 4 node is:" << node.data << endl;
int i=plist->LocateElem(&node3);
cout << "the node3 is loacted at:" << i<< endl;
plist->ListDelete(2, &node6);
cout << "the number 2 is delete:" << node6.data << endl;
cout << "the list's length is:" << plist->ListLength() << endl;
plist->ListTraverse();
plist->ClearList();
plist->ListTraverse();
system("pause");
return 0;
}
2.4结果:
三.将类代替原节点中int data数据:
1.首先定义student类:
#ifndef STUDENT_H_
#define STUDENT_H_
#include<string>
#include<iostream>
using namespace std;
class Student
{
friend ostream & operator<<(ostream &os, Student const &student);
public:
Student(string name="fei", double score=12);
~Student();
Student & operator=(Student const &student);
bool operator==(Student &student);
double Score;
string Name;
};
#endif
#include<string>
#include<iostream>
#include"student.h"
using namespace std;
Student::Student(string name, double score) :Name(name), Score(score){}
Student::~Student(){}
ostream & operator<<(ostream &os, Student const &student)
{
os << student.Name <<','<< student.Score;
return os;
}
Student & Student::operator=(Student const &student)
{
this->Name=student.Name;
this->Score = student.Score;
return *this;
}
bool Student::operator==(Student &student)
{
if ((this->Name == student.Name) && (this->Score == student.Score))
{
return true;
}
else
return false;
}
2.然后将list::List()函数中的对应位置改为:
m_plist = new Node;
m_plist->next = NULL;
m_plist->data.Name ="看不见我" ;
m_plist->data.Score = 25;
m_ilength = 0;
3.测试文件:
#include<iostream>
#include"List.h"
#include"Node.h"
#include"student.h"
using namespace std;
int main()
{
Node node1;
node1.data.Name = "fei";
node1.data.Score = 12;
Node node2;
node2.data.Name = "yan";
node2.data.Score = 13;
Node node3;
node3.data.Name = "jia";
node3.data.Score = 14;
Node node4;
node4.data.Name = "good";
node4.data.Score = 15;
Node node5;
node5.data.Name = "boy";
node5.data.Score = 16;
Node node6;
Node node;
List *plist = new List();
plist->ListInsertHead(&node1);
plist->ListInsertHead(&node2);
plist->ListInsertHead(&node3);
plist->ListInsertHead(&node4);
plist->ListInsertHead(&node5);
plist->ListTraverse();
plist->PriorElem(&node3,&node);
cout << "node3's prior is:"<<node.data << endl;
plist->NextElem(&node3, &node);
cout << "node3's next is:"<<node.data << endl;
plist->GetElem(4, &node);
cout << "number 4 node is:" << node.data << endl;
int i=plist->LocateElem(&node3);
cout << "the node3 is loacted at:" << i<< endl;
plist->ListDelete(2, &node6);
cout << "the number 2 is delete:" << node6.data << endl;
cout << "the list's length is:" << plist->ListLength() << endl;
plist->ListTraverse();
plist->ClearList();
plist->ListTraverse();
system("pause");
return 0;
}
4.结果