一个处理网络请求的开源项目,是安卓端最火热的轻量级框架,由移动支付Square公司贡献(该公司还贡献了Picasso)
用于替代HttpUrlConnection和Apache HttpClient(android API23 6.0里已移除HttpClient,现在已经打不出来)。
尽管Google在大部分安卓版本中推荐使用HttpURLConnection,但是这个类相比HttpClient实在是太难用,太弱爆了。
OkHttp是一个相对成熟的解决方案,据说Android4.4的源码中可以看到HttpURLConnection已经替换成OkHttp实现了。
允许连接到同一个主机地址的所有请求,提高请求效率
共享Socket,减少对服务器的请求次数
通过连接池,减少了请求延迟
缓存响应数据来减少重复的网络请求
减少了对数据流量的消耗
自动处理GZip压缩
二.OkHttp实现Get请求
1.引入包
compile 'com.squareup.okhttp3:okhttp:3.3.0'
compile 'com.squareup.okio:okio:1.5.0'
compile 'com.google.code.gson:gson:2.6.2'
2.权限
<uses-permission android:name="android.permission.INTERNET"/>
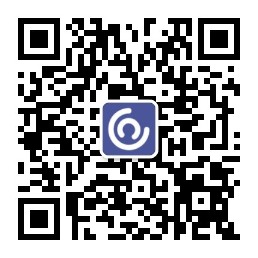
Call是一个请求任务,enqueue是执行
CallBack是请求回调。
请求成功后数据封装在response的body里面。注意:
这些事在子线程里面。如果想更新Ui,还是需要在子线程里面。
最简单的使用:
三. OkHttp实现Post请求
关键代码:
四. OkHttp中同步和异步方法区别
五. OkHttp的拦截器和日志处理
拦截器是一种强大的机制,可以监视、重写和重试调用.下面是一个简单例子,拦截发出的请求和传入的响应的日志.
http://blog.csdn.net/hyb1234hi/article/details/77444099
六. OkHttp的实现图片上传
实际代码展示:
------------------MainActivity-------------------
package com.gjl.day08_ok;
import android.content.Context;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import java.util.List;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private String key = "2f41498b35e69877fc56dc96776e5d1f";
private String url = "http://v.juhe.cn/toutiao/index?type=top&key="+key;
private Button get;
private ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initViews();
}
private void initViews() {
get = findViewById(R.id.get);
get.setOnClickListener(this);
listView = findViewById(R.id.listview);
}
@Override
public void onClick(View v) {
//创建OKHttpClient对象
OKHttpUitls okHttpUitls = new OKHttpUitls();
okHttpUitls.get(url);
okHttpUitls.setOnOKHttpGetListener(new OKHttpUitls.OKHttpGetListener() {
@Override
public void error(String error) {
Toast.makeText(MainActivity.this,error,Toast.LENGTH_SHORT).show();
}
@Override
public void success(String json) {
Toast.makeText(MainActivity.this,json,Toast.LENGTH_SHORT).show();
}
});
}
//adapter
class MyAdapter extends BaseAdapter{
private final Context context;
private final List<NewsBean.ResultBean.DataBean> list;
public MyAdapter(Context context, List<NewsBean.ResultBean.DataBean> list){
this.context = context;
this.list = list;
}
@Override
public int getCount() {
return list.size();
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
TextView textView = new TextView(context);
textView.setText(list.get(position).getTitle());
return textView;
}
}
}
----------------OKHttpUitls---------------------
package com.gjl.day08_ok;
import android.os.Handler;
import android.os.Message;
import android.util.Log;
import java.io.IOException;
import java.util.logging.LogRecord;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
/**
* OKHttp网络请求工具类
* get
* post
* 上传图片
*/
public class OKHttpUitls {
private OKHttpGetListener onOKHttpGetListener;
private MyHandler myHandler = new MyHandler();
private static final String TAG = "OKHttpUitls";
// /get
public void get(String url){
OkHttpClient client = new OkHttpClient();
//创建请求对象
Request request = new Request.Builder().url(url).build();
//创建Call请求队列
//请求都是放到一个队列里面的
Call call = client.newCall(request);
Log.d(TAG, "get() returned: " + call+"------------");
//开始请求
call.enqueue(new Callback() {
// 失败,成功的方法都是在子线程里面,不能直接更新UI
@Override
public void onFailure(Call call, IOException e) {
Message message = myHandler.obtainMessage();
message.obj = "请求失败";
message.what = 0;
myHandler.sendMessage(message);
}
@Override
public void onResponse(Call call, Response response) throws IOException {
Message message = myHandler.obtainMessage();
String json = response.body().string();
message.obj = json;
message.what = 1;
myHandler.sendMessage(message);
}
});
}
//使用接口回到,将数据返回
public interface OKHttpGetListener{
void error(String error);
void success(String json);
}
//给外部调用的方法
public void setOnOKHttpGetListener(OKHttpGetListener onOKHttpGetListener){
this.onOKHttpGetListener = onOKHttpGetListener;
}
//使用Handler,将数据在主线程返回
class MyHandler extends Handler {
@Override
public void handleMessage(Message msg) {
int w = msg.what;
Log.d(TAG, "handleMessage() returned: " +msg );
if (w ==0){
String error = (String) msg.obj;
onOKHttpGetListener.error(error);
}
if (w==1){
String json = (String) msg.obj;
onOKHttpGetListener.success(json);
}
}
}
}
---------------NewsBean------------------
package com.gjl.day08_ok;
import java.util.List;
/**
* Created by Administrator on 2018/1/3 0003.
*/
public class NewsBean {
/**
* reason : 成功的返回
* result : {"stat":"1","data":[{"uniquekey":"3fc894d4c40e3a39f00d655f5078cf81","title":"奈及利亚男子性侵一只母羊,被村民游街驱逐","date":"2018-01-03 09:15","category":"头条","author_name":"默默看国际","url":"http://mini.eastday.com/mobile/180103091508509.html","thumbnail_pic_s":"http://08.imgmini.eastday.com/mobile/20180103/20180103_33a1e18622da28e4cdf071d6af4ed259_mwpm_03200403.jpg","thumbnail_pic_s02":"http://08.imgmini.eastday.com/mobile/20180103/20180103_9b7a2d414c1fc977a54c1442aedbf116_mwpm_03200403.jpg","thumbnail_pic_s03":"http://08.imgmini.eastday.com/mobile/20180103/20180103_ac6d8dcf05fcc0567bb9e5e96ca6daad_mwpm_03200403.jpg"},{"uniquekey":"7cefdd3a97ed4a6ed41e6c25007a8b48","title":"陕西省副省长冯新柱涉嫌严重违纪接受组织审查","date":"2018-01-03 09:07","category":"头条","author_name":"央视网","url":"http://mini.eastday.com/mobile/180103090703672.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103090703_774e7232fe549c170bbebee072a67a34_1_mwpm_03200403.jpg"},{"uniquekey":"8865142aae93d6a378e041006ee7d1b6","title":"秘鲁一巴士坠入悬崖 已造成至少36人死亡","date":"2018-01-03 09:06","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103090633430.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103090633_058503bd2eeb3b0020d2620791813134_1_mwpm_03200403.jpg"},{"uniquekey":"a28479ee0257cd9e0de010c27857cede","title":"整理遗物发现48年前存折,银行说必须本人来,儿子:人死了咋来","date":"2018-01-03 08:51","category":"头条","author_name":"文化拾忆","url":"http://mini.eastday.com/mobile/180103085151854.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103_d3cabadf11f857e114484f361beb6922_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103_92b8904659fa7a0d942ea6fcc8888560_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://02.imgmini.eastday.com/mobile/20180103/20180103_22983eb112aff42b9184526a66515455_cover_mwpm_03200403.jpg"},{"uniquekey":"0d983e5573e2ffb302482d8c0b05547b","title":"一周三次俯卧撑,教你如何练成斯巴达三百勇士般的胸肌","date":"2018-01-03 08:49","category":"头条","author_name":"我为肌肉狂","url":"http://mini.eastday.com/mobile/180103084932535.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103_34a612db510942a220e7c59cbf9ca53a_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103_7f887cd00ff680d88d1557be18f29b54_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103_36ee82ba7248076ba88cc7925c39dcf8_cover_mwpm_03200403.jpg"},{"uniquekey":"cbc61984a4ee4a400746dc1e49ad5235","title":"特朗普新年推特怼完巴基斯坦接着怼巴勒斯坦:不和谈不给钱!","date":"2018-01-03 08:45","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103084558064.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103084558_84f331ab05efca3261958ba7162c57d1_1_mwpm_03200403.jpg"},{"uniquekey":"efa93b77f7578a5bc41eaba6d8f5f8f9","title":"日福岛县浪江町核事故后时隔7年首次举办新年初航仪式","date":"2018-01-03 08:45","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103084557543.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_3_mwpm_03200403.jpg"},{"uniquekey":"084ad1bf9ccb327633c7939124e4076c","title":"兰海高速遵贵扩容项目全线通车 贵阳至遵义节省45分钟","date":"2018-01-03 08:45","category":"头条","author_name":"人民网","url":"http://mini.eastday.com/mobile/180103084525121.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_65bbf5ca393a14a44f7cd1def6f9f9df_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_215233361bda4db4560cfaaddbc451df_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_8ebe220c5e6d733ec7f56168b8c6b4c9_5_mwpm_03200403.jpg"},{"uniquekey":"0429bdce26f4d16ac500209e968b6831","title":"航空报告显示:2017年为民用航空史上最安全的一年","date":"2018-01-03 08:35","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103083541973.html","thumbnail_pic_s":"http://09.imgmini.eastday.com/mobile/20180103/20180103083541_6bc8a95117524a186802dd58316e246b_1_mwpm_03200403.jpg"},{"uniquekey":"6459f8d5a13ee65c85bffb0d2494582b","title":"恭喜这三生肖!2018年不仅赚大钱,还能遇桃花,人生尽如人意","date":"2018-01-03 08:33","category":"头条","author_name":"小易日记","url":"http://mini.eastday.com/mobile/180103083356823.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103_ae86224131d9664f6859f77a40030d94_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103_667f0d85c68013d04fb36640685b4377_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103_d0246e9e776888b296af9061d2629c41_cover_mwpm_03200403.jpg"},{"uniquekey":"c9aa7974fe8dbe3176e332e574fc9e1c","title":"中国正在让日本加速掉入海沟!日本:中国不负责任!网友:我有证","date":"2018-01-03 08:29","category":"头条","author_name":"大宝视频","url":"http://mini.eastday.com/mobile/180103082958505.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180102_c338547e86199124d6fc961773f64d55_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180102_e2495f0704a2acd8e199191e5507fcf9_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180102_2c14f907eb5a764e5ea15ee94c798e7a_cover_mwpm_03200403.jpg"},{"uniquekey":"4527e5e5dbafe5b54edb94d16592f4de","title":"2018最火元旦档 喜剧电影成观众首选","date":"2018-01-03 08:29","category":"头条","author_name":"新华网","url":"http://mini.eastday.com/mobile/180103082956984.html","thumbnail_pic_s":"http://07.imgmini.eastday.com/mobile/20180103/20180103082956_f103605021ea0485397916f45cfc1d86_1_mwpm_03200403.jpg"},{"uniquekey":"8d37cc835cc7157f1b934004912efb32","title":"摄影师拍肯尼亚小狮子暖心画面 勾肩搭背鼓励同伴","date":"2018-01-03 08:28","category":"头条","author_name":"浙江在线","url":"http://mini.eastday.com/mobile/180103082815594.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_257b59127000455bf11fe724309108dc_3_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_d090693a98ea1b9d62cf26f23460638d_1_mwpm_03200403.jpg","thumbnail_pic_s03":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_86a7a88a9a580d05bec35c86d75d0fb5_2_mwpm_03200403.jpg"},{"uniquekey":"35aadb690c8f8291485c6c42891c55e7","title":"美大使为伊朗示威者叫好 还称会寻求联合国介入","date":"2018-01-03 08:25","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103082524087.html","thumbnail_pic_s":"http://00.imgmini.eastday.com/mobile/20180103/20180103082524_0443b6977578832d44a115cbce968551_1_mwpm_03200403.jpg"},{"uniquekey":"31f3301c3c10a00d4a06ac3a8f992116","title":"男子土里挖到锈迹斑斑的铁盒,撬开看到里面这幕,倒吸一口凉气","date":"2018-01-03 08:22","category":"头条","author_name":"经常阅读","url":"http://mini.eastday.com/mobile/180103082237294.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103_2c1ca8f568b7e6c5d33ab3de76f5521d_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103_5bab3bf04ebaf515bc0f0064b4105cc0_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103_bed7e528b99c3403c5133c82951e998e_cover_mwpm_03200403.jpg"},{"uniquekey":"53b0907301fa7eb38217f24c947dff34","title":"国际热点前瞻丨中国在全球治理领域影响将进一步凸显","date":"2018-01-03 08:09","category":"头条","author_name":"中国军网","url":"http://mini.eastday.com/mobile/180103080916054.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103080916_d41d8cd98f00b204e9800998ecf8427e_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103080916_d41d8cd98f00b204e9800998ecf8427e_1_mwpm_03200403.jpg"},{"uniquekey":"0c64b1b216beccbab9fe5dd852ed4c41","title":"莫迪真急眼了!印度下血本也要干这件事,中印边境再次亮红灯","date":"2018-01-03 08:01","category":"头条","author_name":"阿尔法军事","url":"http://mini.eastday.com/mobile/180103080116509.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103_93fe9a76d3f058729573c9de1d2ba404_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://05.imgmini.eastday.com/mobile/20180103/20180103_d5cbab9bd3067a185adf59dbea3dc05a_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://05.imgmini.eastday.com/mobile/20180103/20180103_0e5533c90e1096aae68e23b2d2c7ba4f_cover_mwpm_03200403.jpg"},{"uniquekey":"973b14d859125c050edcc4843ec8bff5","title":"驻扎中国唯一一支外国军队,只有中国敢\u201c立规矩\u201d!","date":"2018-01-03 07:48","category":"头条","author_name":"军迷亮点","url":"http://mini.eastday.com/mobile/180103074849592.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103_937a51a621e15b8e654ac024d4e9a532_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103_0b19cd313379ad222aa7d4b615fa3af7_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103_5378b437dd84aef0824685c55f34d958_cover_mwpm_03200403.jpg"},{"uniquekey":"a905bd9600fae3c402b4ab5d796216dc","title":"组图:开年辣!陈志朋穿透视蕾丝装豪放露大腿","date":"2018-01-03 07:47","category":"头条","author_name":"视觉中国","url":"http://mini.eastday.com/mobile/180103074721371.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_2_mwpm_03200403.jpg"},{"uniquekey":"e2e2d0bcefb26eefe44b91e2b7eeec97","title":"2018年!国家终于说清楚,P2P理财的收益为什么比银行高!","date":"2018-01-03 07:47","category":"头条","author_name":"富贵树微金融mp","url":"http://mini.eastday.com/mobile/180103074706123.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_8_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_7_mwpm_03200403.jpg"},{"uniquekey":"8f668d35d454ca26eaad400956ffc44b","title":"超实用!2018年春运火车票今发售 购买全攻略","date":"2018-01-03 07:46","category":"头条","author_name":"人民日报","url":"http://mini.eastday.com/mobile/180103074624429.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_5_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_9_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_3_mwpm_03200403.jpg"},{"uniquekey":"e9aff679eb60f5b65802f0d154b92915","title":"特朗普炮轰完\"巴铁\"又威胁巴勒斯坦:小心断你援助","date":"2018-01-03 07:44","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103074425303.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103074425_29c2af26f0b40755c952991176e747e1_1_mwpm_03200403.jpg"},{"uniquekey":"cbc80337db202a8f7f8c908e24a2c808","title":"近日召开的中央农村工作会议提出必须重塑城乡关系:城乡发展怎么\u201c融\u201d","date":"2018-01-03 07:41","category":"头条","author_name":"中国经济网","url":"http://mini.eastday.com/mobile/180103074109935.html","thumbnail_pic_s":"http://09.imgmini.eastday.com/mobile/20180103/20180103074109_93242998b9ae6cae3fed0af10a587984_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://09.imgmini.eastday.com/mobile/20180103/20180103074109_93242998b9ae6cae3fed0af10a587984_1_mwpm_03200403.jpg"},{"uniquekey":"aaa6ec757315e9631b745a0a454099a0","title":"入冬后首个暴雪警报来了 江苏交警发布安全出行提示","date":"2018-01-03 07:40","category":"头条","author_name":"扬子晚报网","url":"http://mini.eastday.com/mobile/180103074017351.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103074017_b5d107f89565fb417b4593f70701dba9_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://04.imgmini.eastday.com/mobile/20180103/20180103074017_b5d107f89565fb417b4593f70701dba9_2_mwpm_03200403.jpg"},{"uniquekey":"44efe30915df48375cbe2cbabb677559","title":"昨夜雪已到河南 预计黄河以南地区今夜大雪纷飞局部大暴雪","date":"2018-01-03 07:40","category":"头条","author_name":"映象网","url":"http://mini.eastday.com/mobile/180103074005990.html","thumbnail_pic_s":"http://08.imgmini.eastday.com/mobile/20180103/20180103074005_c29bfebe1e90e140f8412d1cb5dd463b_1_mwpm_03200403.jpg"},{"uniquekey":"ccec67cc14897ffdb952379df9944fd0","title":"上海吴淞口凌晨发生沉船事故 10名船员下落不明","date":"2018-01-03 07:37","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103073759599.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103073759_5c86c160492ff65b36ffe086ec1ceea2_1_mwpm_03200403.jpg"},{"uniquekey":"020549445a5b2ea94ffc1289aa77d24b","title":"世界上最早的彩色照片,百年前的少女居然这么美!","date":"2018-01-03 07:36","category":"头条","author_name":"嗅怪猎奇","url":"http://mini.eastday.com/mobile/180103073606032.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103_8215e2023e55bc83026804746b587eaa_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103_6028c4dc05696335127b931b5528028d_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103_83405e85722df5ca0bb63f6debb86533_cover_mwpm_03200403.jpg"},{"uniquekey":"d63630cfe99c9799a85797959d92f382","title":"纽约布朗克斯区大楼再次发生大火 至少23伤","date":"2018-01-03 07:34","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103073415931.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103073415_d609197dba9fcb819afc93aee6785134_1_mwpm_03200403.jpg"},{"uniquekey":"3d2a052a787fbeb51a9adfc6b4017cf6","title":"英国女生节,美女穿着暴露豪放酗酒,完全就成了个疯狂之国","date":"2018-01-03 07:33","category":"头条","author_name":"嗅怪猎奇","url":"http://mini.eastday.com/mobile/180103073353239.html","thumbnail_pic_s":"http://00.imgmini.eastday.com/mobile/20180103/20180103_8d4c7643f41488505190fac6924fc1f6_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://00.imgmini.eastday.com/mobile/20180103/20180103_349aa78b07db8ea5818c8b942c2739af_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://00.imgmini.eastday.com/mobile/20180103/20180103_5d6abe868b72cf413414a9be024bdaea_cover_mwpm_03200403.jpg"},{"uniquekey":"5fec95e0596709fa92f47188cbf1e389","title":"港媒:为中国潜艇\u201c保驾护航\u201d 这个高科技可谓无价之宝\u2014\u2014","date":"2018-01-03 07:26","category":"头条","author_name":"参考消息网","url":"http://mini.eastday.com/mobile/180103072622163.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_4_mwpm_03200403.jpg","thumbnail_pic_s02":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_3_mwpm_03200403.jpg","thumbnail_pic_s03":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_2_mwpm_03200403.jpg"}]}
* error_code : 0
*/
private String reason;
private ResultBean result;
private int error_code;
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public ResultBean getResult() {
return result;
}
public void setResult(ResultBean result) {
this.result = result;
}
public int getError_code() {
return error_code;
}
public void setError_code(int error_code) {
this.error_code = error_code;
}
public static class ResultBean {
/**
* stat : 1
* data : [{"uniquekey":"3fc894d4c40e3a39f00d655f5078cf81","title":"奈及利亚男子性侵一只母羊,被村民游街驱逐","date":"2018-01-03 09:15","category":"头条","author_name":"默默看国际","url":"http://mini.eastday.com/mobile/180103091508509.html","thumbnail_pic_s":"http://08.imgmini.eastday.com/mobile/20180103/20180103_33a1e18622da28e4cdf071d6af4ed259_mwpm_03200403.jpg","thumbnail_pic_s02":"http://08.imgmini.eastday.com/mobile/20180103/20180103_9b7a2d414c1fc977a54c1442aedbf116_mwpm_03200403.jpg","thumbnail_pic_s03":"http://08.imgmini.eastday.com/mobile/20180103/20180103_ac6d8dcf05fcc0567bb9e5e96ca6daad_mwpm_03200403.jpg"},{"uniquekey":"7cefdd3a97ed4a6ed41e6c25007a8b48","title":"陕西省副省长冯新柱涉嫌严重违纪接受组织审查","date":"2018-01-03 09:07","category":"头条","author_name":"央视网","url":"http://mini.eastday.com/mobile/180103090703672.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103090703_774e7232fe549c170bbebee072a67a34_1_mwpm_03200403.jpg"},{"uniquekey":"8865142aae93d6a378e041006ee7d1b6","title":"秘鲁一巴士坠入悬崖 已造成至少36人死亡","date":"2018-01-03 09:06","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103090633430.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103090633_058503bd2eeb3b0020d2620791813134_1_mwpm_03200403.jpg"},{"uniquekey":"a28479ee0257cd9e0de010c27857cede","title":"整理遗物发现48年前存折,银行说必须本人来,儿子:人死了咋来","date":"2018-01-03 08:51","category":"头条","author_name":"文化拾忆","url":"http://mini.eastday.com/mobile/180103085151854.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103_d3cabadf11f857e114484f361beb6922_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103_92b8904659fa7a0d942ea6fcc8888560_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://02.imgmini.eastday.com/mobile/20180103/20180103_22983eb112aff42b9184526a66515455_cover_mwpm_03200403.jpg"},{"uniquekey":"0d983e5573e2ffb302482d8c0b05547b","title":"一周三次俯卧撑,教你如何练成斯巴达三百勇士般的胸肌","date":"2018-01-03 08:49","category":"头条","author_name":"我为肌肉狂","url":"http://mini.eastday.com/mobile/180103084932535.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103_34a612db510942a220e7c59cbf9ca53a_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103_7f887cd00ff680d88d1557be18f29b54_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103_36ee82ba7248076ba88cc7925c39dcf8_cover_mwpm_03200403.jpg"},{"uniquekey":"cbc61984a4ee4a400746dc1e49ad5235","title":"特朗普新年推特怼完巴基斯坦接着怼巴勒斯坦:不和谈不给钱!","date":"2018-01-03 08:45","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103084558064.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103084558_84f331ab05efca3261958ba7162c57d1_1_mwpm_03200403.jpg"},{"uniquekey":"efa93b77f7578a5bc41eaba6d8f5f8f9","title":"日福岛县浪江町核事故后时隔7年首次举办新年初航仪式","date":"2018-01-03 08:45","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103084557543.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103084557_345a835484ac3149fb76474cb2d4a48b_3_mwpm_03200403.jpg"},{"uniquekey":"084ad1bf9ccb327633c7939124e4076c","title":"兰海高速遵贵扩容项目全线通车 贵阳至遵义节省45分钟","date":"2018-01-03 08:45","category":"头条","author_name":"人民网","url":"http://mini.eastday.com/mobile/180103084525121.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_65bbf5ca393a14a44f7cd1def6f9f9df_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_215233361bda4db4560cfaaddbc451df_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103084525_8ebe220c5e6d733ec7f56168b8c6b4c9_5_mwpm_03200403.jpg"},{"uniquekey":"0429bdce26f4d16ac500209e968b6831","title":"航空报告显示:2017年为民用航空史上最安全的一年","date":"2018-01-03 08:35","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103083541973.html","thumbnail_pic_s":"http://09.imgmini.eastday.com/mobile/20180103/20180103083541_6bc8a95117524a186802dd58316e246b_1_mwpm_03200403.jpg"},{"uniquekey":"6459f8d5a13ee65c85bffb0d2494582b","title":"恭喜这三生肖!2018年不仅赚大钱,还能遇桃花,人生尽如人意","date":"2018-01-03 08:33","category":"头条","author_name":"小易日记","url":"http://mini.eastday.com/mobile/180103083356823.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103_ae86224131d9664f6859f77a40030d94_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103_667f0d85c68013d04fb36640685b4377_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103_d0246e9e776888b296af9061d2629c41_cover_mwpm_03200403.jpg"},{"uniquekey":"c9aa7974fe8dbe3176e332e574fc9e1c","title":"中国正在让日本加速掉入海沟!日本:中国不负责任!网友:我有证","date":"2018-01-03 08:29","category":"头条","author_name":"大宝视频","url":"http://mini.eastday.com/mobile/180103082958505.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180102_c338547e86199124d6fc961773f64d55_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180102_e2495f0704a2acd8e199191e5507fcf9_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180102_2c14f907eb5a764e5ea15ee94c798e7a_cover_mwpm_03200403.jpg"},{"uniquekey":"4527e5e5dbafe5b54edb94d16592f4de","title":"2018最火元旦档 喜剧电影成观众首选","date":"2018-01-03 08:29","category":"头条","author_name":"新华网","url":"http://mini.eastday.com/mobile/180103082956984.html","thumbnail_pic_s":"http://07.imgmini.eastday.com/mobile/20180103/20180103082956_f103605021ea0485397916f45cfc1d86_1_mwpm_03200403.jpg"},{"uniquekey":"8d37cc835cc7157f1b934004912efb32","title":"摄影师拍肯尼亚小狮子暖心画面 勾肩搭背鼓励同伴","date":"2018-01-03 08:28","category":"头条","author_name":"浙江在线","url":"http://mini.eastday.com/mobile/180103082815594.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_257b59127000455bf11fe724309108dc_3_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_d090693a98ea1b9d62cf26f23460638d_1_mwpm_03200403.jpg","thumbnail_pic_s03":"http://02.imgmini.eastday.com/mobile/20180103/20180103082815_86a7a88a9a580d05bec35c86d75d0fb5_2_mwpm_03200403.jpg"},{"uniquekey":"35aadb690c8f8291485c6c42891c55e7","title":"美大使为伊朗示威者叫好 还称会寻求联合国介入","date":"2018-01-03 08:25","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103082524087.html","thumbnail_pic_s":"http://00.imgmini.eastday.com/mobile/20180103/20180103082524_0443b6977578832d44a115cbce968551_1_mwpm_03200403.jpg"},{"uniquekey":"31f3301c3c10a00d4a06ac3a8f992116","title":"男子土里挖到锈迹斑斑的铁盒,撬开看到里面这幕,倒吸一口凉气","date":"2018-01-03 08:22","category":"头条","author_name":"经常阅读","url":"http://mini.eastday.com/mobile/180103082237294.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103_2c1ca8f568b7e6c5d33ab3de76f5521d_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103_5bab3bf04ebaf515bc0f0064b4105cc0_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103_bed7e528b99c3403c5133c82951e998e_cover_mwpm_03200403.jpg"},{"uniquekey":"53b0907301fa7eb38217f24c947dff34","title":"国际热点前瞻丨中国在全球治理领域影响将进一步凸显","date":"2018-01-03 08:09","category":"头条","author_name":"中国军网","url":"http://mini.eastday.com/mobile/180103080916054.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103080916_d41d8cd98f00b204e9800998ecf8427e_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://02.imgmini.eastday.com/mobile/20180103/20180103080916_d41d8cd98f00b204e9800998ecf8427e_1_mwpm_03200403.jpg"},{"uniquekey":"0c64b1b216beccbab9fe5dd852ed4c41","title":"莫迪真急眼了!印度下血本也要干这件事,中印边境再次亮红灯","date":"2018-01-03 08:01","category":"头条","author_name":"阿尔法军事","url":"http://mini.eastday.com/mobile/180103080116509.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103_93fe9a76d3f058729573c9de1d2ba404_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://05.imgmini.eastday.com/mobile/20180103/20180103_d5cbab9bd3067a185adf59dbea3dc05a_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://05.imgmini.eastday.com/mobile/20180103/20180103_0e5533c90e1096aae68e23b2d2c7ba4f_cover_mwpm_03200403.jpg"},{"uniquekey":"973b14d859125c050edcc4843ec8bff5","title":"驻扎中国唯一一支外国军队,只有中国敢\u201c立规矩\u201d!","date":"2018-01-03 07:48","category":"头条","author_name":"军迷亮点","url":"http://mini.eastday.com/mobile/180103074849592.html","thumbnail_pic_s":"http://06.imgmini.eastday.com/mobile/20180103/20180103_937a51a621e15b8e654ac024d4e9a532_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://06.imgmini.eastday.com/mobile/20180103/20180103_0b19cd313379ad222aa7d4b615fa3af7_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://06.imgmini.eastday.com/mobile/20180103/20180103_5378b437dd84aef0824685c55f34d958_cover_mwpm_03200403.jpg"},{"uniquekey":"a905bd9600fae3c402b4ab5d796216dc","title":"组图:开年辣!陈志朋穿透视蕾丝装豪放露大腿","date":"2018-01-03 07:47","category":"头条","author_name":"视觉中国","url":"http://mini.eastday.com/mobile/180103074721371.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_4_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103074721_c0659ea763834321f618b2b472685f35_2_mwpm_03200403.jpg"},{"uniquekey":"e2e2d0bcefb26eefe44b91e2b7eeec97","title":"2018年!国家终于说清楚,P2P理财的收益为什么比银行高!","date":"2018-01-03 07:47","category":"头条","author_name":"富贵树微金融mp","url":"http://mini.eastday.com/mobile/180103074706123.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_8_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103074706_37832c54bc7e4aa77ba76733ec5f744c_7_mwpm_03200403.jpg"},{"uniquekey":"8f668d35d454ca26eaad400956ffc44b","title":"超实用!2018年春运火车票今发售 购买全攻略","date":"2018-01-03 07:46","category":"头条","author_name":"人民日报","url":"http://mini.eastday.com/mobile/180103074624429.html","thumbnail_pic_s":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_5_mwpm_03200403.jpg","thumbnail_pic_s02":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_9_mwpm_03200403.jpg","thumbnail_pic_s03":"http://01.imgmini.eastday.com/mobile/20180103/20180103074624_b676a975bc2ac85139fefa68780ccf55_3_mwpm_03200403.jpg"},{"uniquekey":"e9aff679eb60f5b65802f0d154b92915","title":"特朗普炮轰完\"巴铁\"又威胁巴勒斯坦:小心断你援助","date":"2018-01-03 07:44","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103074425303.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103074425_29c2af26f0b40755c952991176e747e1_1_mwpm_03200403.jpg"},{"uniquekey":"cbc80337db202a8f7f8c908e24a2c808","title":"近日召开的中央农村工作会议提出必须重塑城乡关系:城乡发展怎么\u201c融\u201d","date":"2018-01-03 07:41","category":"头条","author_name":"中国经济网","url":"http://mini.eastday.com/mobile/180103074109935.html","thumbnail_pic_s":"http://09.imgmini.eastday.com/mobile/20180103/20180103074109_93242998b9ae6cae3fed0af10a587984_2_mwpm_03200403.jpg","thumbnail_pic_s02":"http://09.imgmini.eastday.com/mobile/20180103/20180103074109_93242998b9ae6cae3fed0af10a587984_1_mwpm_03200403.jpg"},{"uniquekey":"aaa6ec757315e9631b745a0a454099a0","title":"入冬后首个暴雪警报来了 江苏交警发布安全出行提示","date":"2018-01-03 07:40","category":"头条","author_name":"扬子晚报网","url":"http://mini.eastday.com/mobile/180103074017351.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103074017_b5d107f89565fb417b4593f70701dba9_1_mwpm_03200403.jpg","thumbnail_pic_s02":"http://04.imgmini.eastday.com/mobile/20180103/20180103074017_b5d107f89565fb417b4593f70701dba9_2_mwpm_03200403.jpg"},{"uniquekey":"44efe30915df48375cbe2cbabb677559","title":"昨夜雪已到河南 预计黄河以南地区今夜大雪纷飞局部大暴雪","date":"2018-01-03 07:40","category":"头条","author_name":"映象网","url":"http://mini.eastday.com/mobile/180103074005990.html","thumbnail_pic_s":"http://08.imgmini.eastday.com/mobile/20180103/20180103074005_c29bfebe1e90e140f8412d1cb5dd463b_1_mwpm_03200403.jpg"},{"uniquekey":"ccec67cc14897ffdb952379df9944fd0","title":"上海吴淞口凌晨发生沉船事故 10名船员下落不明","date":"2018-01-03 07:37","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103073759599.html","thumbnail_pic_s":"http://04.imgmini.eastday.com/mobile/20180103/20180103073759_5c86c160492ff65b36ffe086ec1ceea2_1_mwpm_03200403.jpg"},{"uniquekey":"020549445a5b2ea94ffc1289aa77d24b","title":"世界上最早的彩色照片,百年前的少女居然这么美!","date":"2018-01-03 07:36","category":"头条","author_name":"嗅怪猎奇","url":"http://mini.eastday.com/mobile/180103073606032.html","thumbnail_pic_s":"http://03.imgmini.eastday.com/mobile/20180103/20180103_8215e2023e55bc83026804746b587eaa_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://03.imgmini.eastday.com/mobile/20180103/20180103_6028c4dc05696335127b931b5528028d_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://03.imgmini.eastday.com/mobile/20180103/20180103_83405e85722df5ca0bb63f6debb86533_cover_mwpm_03200403.jpg"},{"uniquekey":"d63630cfe99c9799a85797959d92f382","title":"纽约布朗克斯区大楼再次发生大火 至少23伤","date":"2018-01-03 07:34","category":"头条","author_name":"环球网","url":"http://mini.eastday.com/mobile/180103073415931.html","thumbnail_pic_s":"http://02.imgmini.eastday.com/mobile/20180103/20180103073415_d609197dba9fcb819afc93aee6785134_1_mwpm_03200403.jpg"},{"uniquekey":"3d2a052a787fbeb51a9adfc6b4017cf6","title":"英国女生节,美女穿着暴露豪放酗酒,完全就成了个疯狂之国","date":"2018-01-03 07:33","category":"头条","author_name":"嗅怪猎奇","url":"http://mini.eastday.com/mobile/180103073353239.html","thumbnail_pic_s":"http://00.imgmini.eastday.com/mobile/20180103/20180103_8d4c7643f41488505190fac6924fc1f6_cover_mwpm_03200403.jpg","thumbnail_pic_s02":"http://00.imgmini.eastday.com/mobile/20180103/20180103_349aa78b07db8ea5818c8b942c2739af_cover_mwpm_03200403.jpg","thumbnail_pic_s03":"http://00.imgmini.eastday.com/mobile/20180103/20180103_5d6abe868b72cf413414a9be024bdaea_cover_mwpm_03200403.jpg"},{"uniquekey":"5fec95e0596709fa92f47188cbf1e389","title":"港媒:为中国潜艇\u201c保驾护航\u201d 这个高科技可谓无价之宝\u2014\u2014","date":"2018-01-03 07:26","category":"头条","author_name":"参考消息网","url":"http://mini.eastday.com/mobile/180103072622163.html","thumbnail_pic_s":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_4_mwpm_03200403.jpg","thumbnail_pic_s02":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_3_mwpm_03200403.jpg","thumbnail_pic_s03":"http://05.imgmini.eastday.com/mobile/20180103/20180103072622_d41d8cd98f00b204e9800998ecf8427e_2_mwpm_03200403.jpg"}]
*/
private String stat;
private List<DataBean> data;
public String getStat() {
return stat;
}
public void setStat(String stat) {
this.stat = stat;
}
public List<DataBean> getData() {
return data;
}
public void setData(List<DataBean> data) {
this.data = data;
}
public static class DataBean {
/**
* uniquekey : 3fc894d4c40e3a39f00d655f5078cf81
* title : 奈及利亚男子性侵一只母羊,被村民游街驱逐
* date : 2018-01-03 09:15
* category : 头条
* author_name : 默默看国际
* url : http://mini.eastday.com/mobile/180103091508509.html
* thumbnail_pic_s : http://08.imgmini.eastday.com/mobile/20180103/20180103_33a1e18622da28e4cdf071d6af4ed259_mwpm_03200403.jpg
* thumbnail_pic_s02 : http://08.imgmini.eastday.com/mobile/20180103/20180103_9b7a2d414c1fc977a54c1442aedbf116_mwpm_03200403.jpg
* thumbnail_pic_s03 : http://08.imgmini.eastday.com/mobile/20180103/20180103_ac6d8dcf05fcc0567bb9e5e96ca6daad_mwpm_03200403.jpg
*/
private String uniquekey;
private String title;
private String date;
private String category;
private String author_name;
private String url;
private String thumbnail_pic_s;
private String thumbnail_pic_s02;
private String thumbnail_pic_s03;
public String getUniquekey() {
return uniquekey;
}
public void setUniquekey(String uniquekey) {
this.uniquekey = uniquekey;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public String getAuthor_name() {
return author_name;
}
public void setAuthor_name(String author_name) {
this.author_name = author_name;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getThumbnail_pic_s() {
return thumbnail_pic_s;
}
public void setThumbnail_pic_s(String thumbnail_pic_s) {
this.thumbnail_pic_s = thumbnail_pic_s;
}
public String getThumbnail_pic_s02() {
return thumbnail_pic_s02;
}
public void setThumbnail_pic_s02(String thumbnail_pic_s02) {
this.thumbnail_pic_s02 = thumbnail_pic_s02;
}
public String getThumbnail_pic_s03() {
return thumbnail_pic_s03;
}
public void setThumbnail_pic_s03(String thumbnail_pic_s03) {
this.thumbnail_pic_s03 = thumbnail_pic_s03;
}
}
}
}
--------------------activity_main.xml------------------
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.gjl.day08_ok.MainActivity"> <Button android:id="@+id/get" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="get请求"/> <ListView android:id="@+id/listview" android:layout_width="match_parent" android:layout_height="wrap_content"> </ListView> </LinearLayout>