SpringBoot----读取配置文件中的属性
1、继续使用上一篇文章的项目springboot4,修改配置文件application.properties文件如下:
###############################定义属性###############################
com.etc.name=zoey
com.etc.title=测试属性
com.etc.desc=${com.etc.name}在${com.etc.title}
#随机字符串
com.etc.test=${random.value}
#随机int
com.etc.number=${random.int}
#10以内的随机整数
com.etc.test1=${random.int(10)}
2、在com.etc.service包下新建TestProperties.java类如下:
package com.etc.service;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
/**
* @Description:属性实体类:获取配置文件中属性
* @author zoey
* @date:2018年3月13日
*/
@Component//加了这个属性,才能够扫描到这个类,否则无法注入到其他类中去
public class TestProperties {
//通过@Value("${属性名}")注解来加载对应的配置属性
@Value("${com.etc.name}")
private String name;
@Value("${com.etc.title}")
private String title;
@Value("${com.etc.desc}")
private String desc;
@Value("${com.etc.test}")
private String test;
@Value("${com.etc.number}")
private int number;
@Value("${com.etc.test1}")
private int test1;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public String getTest() {
return test;
}
public void setTest(String test) {
this.test = test;
}
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
public int getTest1() {
return test1;
}
public void setTest1(int test1) {
this.test1 = test1;
}
}
3、在src/main/test文件夹下的com.etc.test包下的ApplicationTest.java类中进行测试如下:
package com.etc.springboot4;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import com.etc.domain.User;
import com.etc.domain.UserRepository;
import com.etc.service.TestProperties;
//要作为SpringBoot的测试类,必须要添加这两个注解
@RunWith(SpringRunner.class)//指定使用的单元测试执行类,SpringRunner是spring-test提供的测试执行单元类
@SpringBootTest //类似springboot程序的测试引导入口
public class ApplicationTest {
@Autowired
private TestProperties testProperties;
@Test
public void testProperties() {
System.out.println("测试属性");
String name = testProperties.getName();
System.out.println("name:"+name);
String desc = testProperties.getDesc();
System.out.println("desc:"+desc);
int test1 = testProperties.getTest1();
System.out.println("test1:"+test1);
}
}
4、在com.etc.controller包下的UserController.java类中编写代码如下:
@RequestMapping("/users")
@RestController
@Transactional
public class UserController {
@Autowired
private TestProperties testProperties;
@Value("${com.etc.test1}")
private int test1 ;
/**
* @Description:读取配置文件中的属性
* 访问地址:http://localhost:8080/users/test
* @return
* @author:zoey
* @date:2018年3月13日
*/
@RequestMapping("/test")
public String testProperties() {
System.out.println("测试属性");
String name = testProperties.getName();
int number = testProperties.getNumber();
System.out.println("name:"+name+"number:"+number);
System.out.println("======================");
System.out.println("test1:"+test1);
return "test";
}
}
5、运行测试类:Run As-->JUnit Test
运行结果如下:
有一个问题:中文乱码(读取配置文件中属性,中文乱码),如何解决??
6、运行启动类:Run As--> Java Application
访问地址:http://localhost:8080/users/test
扫描二维码关注公众号,回复:
2408330 查看本文章
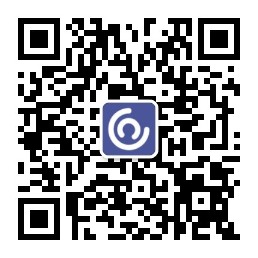
运行结果如下:
测试属性
name:zoeynumber:1086588778
======================
test1:3
总结:
想要读取配置文件中的属性,需要进行如下操作:
- 在配置文件中定义属性
- 使用@Value(${xxx})注解获取属性的值(也可以专门定义一个类,获取配置文件中的属性,到时候在别的类中想要读取配置文件中的属性时,只需要注入这个类便可以)
- 读取配置文件的中文值时,会乱码,该如何解决??