1.自带的pool
QueuedThreadPool pool = new QueuedThreadPool(); pool.setMinThreads(Integer.parseInt(PropertiesUtil.getValue("api_threadpool_min_size"))); pool.setMaxThreads(Integer.parseInt(PropertiesUtil.getValue("api_threadpool_max_size"))); pool.setMaxIdleTimeMs(200); SelectChannelConnector connection0 = new SelectChannelConnector(); connection0.setPort(Integer.parseInt(PropertiesUtil.getValue("jetty.port"))); connection0.setMaxIdleTime(10000); server.setThreadPool(pool);
小技巧:
这里可以设置只接受的线程数,如果超过,那么等待的线程立刻回复busy
if (pool.getThreads() >= pool.getMaxThreads()) { WritePrint(response, "<?xml version=\"1.0\"?><Request><ErrorCode>2</ErrorCode><ResponseMsg>System is busy</ResponseMsg></Request>"); logger.info("Return response:<?xml version=\"1.0\"?><Request><ErrorCode>2</ErrorCode><ResponseMsg>System is busy</ResponseMsg></Request>"); return; }
public void WritePrint(HttpServletResponse response, String outWriter) { try { PrintWriter out = response.getWriter(); out.println(outWriter); out.flush(); out.close(); } catch (IOException e1) { e1.printStackTrace(); } }
注意:jetty主线程占一条,最后等于max thread的线程会rejected,所以实际执行的线程数是(设置的MAX线程-2)
2.第三方pool
先建立pool类
public class ThreadPool { private static Logger logger = LoggerFactory.getLogger(IrapiServer.class); private ThreadPool() {} private static ThreadPoolExecutor pool; public static void initThreadPool(ThreadPoolExecutor pool){ ThreadPool.pool = pool; } public static ThreadPoolExecutor createThreadPool() { int corePoolsize = Integer.valueOf(PropertiesUtil.getValue("api_threadpool_min_size")); int maximumPoolSize = Integer.valueOf(PropertiesUtil.getValue("api_threadpool_max_size")); BlockingQueue<Runnable> queue = new LinkedBlockingQueue<Runnable>(); logger.info("create thread pool: corePoolsize=" + corePoolsize + ", maximumPoolSize=" + maximumPoolSize); ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor( corePoolsize, maximumPoolSize, 1, TimeUnit.SECONDS, queue, new ThreadPoolExecutor.CallerRunsPolicy()); return threadPoolExecutor; } public static ThreadPoolExecutor getThreadPool(){ return ThreadPool.pool; } }
然后在主方法中调用:
ThreadPool.initThreadPool(ThreadPool.createThreadPool());
扫描二维码关注公众号,回复:
240005 查看本文章
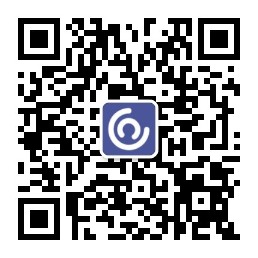
最后,service类要继承线程类,然后执行:
ThreadPool.getThreadPool().execute(irapiServiceHandler);
这样设置等待线程的线程立刻rejected:
if ((ThreadPool.getThreadPool().getTaskCount() - ThreadPool.getThreadPool().getCompletedTaskCount()) >= maximumPoolSize) { WritePrint(response, "<?xml version=\"1.0\"?><Request><ErrorCode>2</ErrorCode><ResponseMsg>System is busy</ResponseMsg></Request>"); logger.info("Return response:<?xml version=\"1.0\"?><Request><ErrorCode>2</ErrorCode><ResponseMsg>System is busy</ResponseMsg></Request>"); return; }