
1 #成员修饰符 修饰符可以规定内部的字段、属性、方法等 是共有的成员,私有的成员 2 class Foo: 3 def __init__(self,name,age): 4 self.name=name 5 self.age=age #可以在外部直接访问 6 7 obj=Foo('Jack',22) 8 print(obj.name) 9 print(obj.age)
1 #成员修饰符 修饰符可以规定内部的字段、属性、方法等 是共有的成员,私有的成员 2 class Foo: 3 def __init__(self,name,age): 4 self.name=name 5 #self.age=age #可以在外部直接访问 6 self.__age=age #添加 __ 两个下划线,就变成私有字段,外部不能访问 7 obj=Foo('Jack',22) 8 print(obj.name) 9 print(obj.age)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py Traceback (most recent call last): Jack File "F:/python从入门到放弃/7.24/面向对象.py", line 16, in <module> print(obj.age) AttributeError: 'Foo' object has no attribute 'age' Process finished with exit code 1
私有字段外部不能直接访问。可以通过内部的方法,间接访问
1 #成员修饰符 修饰符可以规定内部的字段、属性、方法等 是共有的成员,私有的成员 2 class Foo: 3 def __init__(self,name,age): 4 self.name=name 5 #self.age=age #可以在外部直接访问 6 self.__age=age #添加 __ 两个下划线,就变成私有字段,外部不能访问 7 def get_age(self): 8 return self.__age 9 obj=Foo('Jack',22) 10 print(obj.name) 11 print(obj.get_age())
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py Jack 22 Process finished with exit code 0

1 #成员修饰符 修饰符可以规定内部的字段、属性、方法等 是共有的成员,私有的成员 2 class Foo: 3 gender='男' #对于静态字段 4 def __init__(self,name,age): 5 self.name=name 6 #self.age=age #对于普通字段可以在外部直接访问 7 self.__age=age #添加 __ 两个下划线,就变成私有字段,外部不能访问 8 def get_age(self): 9 return self.__age 10 obj=Foo('Jack',22) 11 # print(obj.name) 12 # print(obj.get_age()) 13 print(obj.gender)

1 class Foo: 2 #gender='男' #对于静态字段 3 __gender = '男' 4 def __init__(self,name,age): 5 self.name=name 6 #self.age=age #对于普通字段可以在外部直接访问 7 self.__age=age #添加 __ 两个下划线,就变成私有字段,外部不能访问 8 def get_age(self): 9 return self.__age 10 def get_gender(self): 11 return Foo.__gender 12 obj=Foo('Jack',22) 13 # print(obj.name) 14 # print(obj.get_age()) 15 print(obj.get_gender())

1 class Foo: 2 __gender='男' 3 def __init__(self): 4 pass 5 6 # def get_gender(self): 7 # return Foo.__gender 8 9 @staticmethod 10 def get_gender(): 11 return Foo.__gender 12 # obj=Foo() 13 # print(obj.get_gender()) 14 print(Foo.get_gender())

1 #案例 数据库账户 2 class Database: 3 __root="root" 4 __pwd='abc123' 5 __port='3306' 6 __dbname='ssoa' 7 # def __init__(self,pwd): 8 # pass 9 ###############只读模式######################## 10 def get_root(self): #只能访问,不能修改 可读 11 return self.__root 12 def get_port(self): 13 return self.__port 14 15 ###########读写模式 ################# 16 def get_pwd(self): #获取密码 17 return self.__pwd 18 def set_pwd(self,pwd): #修改密码 19 self.__pwd = pwd 20 21 db= Database() 22 print(db.get_pwd()) #调用密码 23 db.set_pwd('456') #修改密码 24 print(db.get_pwd())#调用密码

1 class ClassFather: 2 def __init__(self): 3 self.__age=23 4 self.score=90 5 6 class ClassSon(ClassFather): 7 def __init__(self,name): 8 self.name=name 9 self.__gender='男' 10 super().__init__() 11 12 def show(self): 13 print(self.name) 14 print(self.__gender) 15 print(self.score) 16 #print(self.__age)#私有字段只能在类的内部使用,不能被继承 17 18 s=ClassSon('李逵') 19 s.show()
类的特殊成员
__init__/__call__
1 class Foo: 2 def __init__(self): #对象后面加上() 自动执行 init 方法 3 print('init') 4 5 6 def __call__(self, *args, **kwargs): ##对象后面加() 自动执行 call 方法 7 print('call') 8 9 10 obj=Foo() 11 obj()
##相当于 Foo()()
__int__/__str__
1 class Foo: 2 def __init__(self): 3 pass 4 def __int__(self): 5 return 111 6 def __str__(self): 7 return 'string' 8 obj=Foo() 9 10 print(obj,'\n',type(obj)) 11 12 #int ,对象,自动执行对象的__int__ 方法,并将返回值赋值给int对象 13 r=int(obj) 14 print(r) 15 #str ,对象,自动执行对象的__str__ 方法,并将返回值赋值给str对象 16 t=str(obj) 17 print(t)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py string <class '__main__.Foo'> 111 string Process finished with exit code 0
在实际使用中,使用__str__() 方法的频率更大
例如:
扫描二维码关注公众号,回复:
2364277 查看本文章
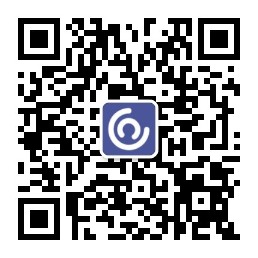
1 class Foo: 2 def __init__(self,name,age): 3 self.name=name 4 self.age=age 5 6 obj=Foo('Jach',12) 7 print(obj)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py <__main__.Foo object at 0x000000000222D1D0> Process finished with exit code 0
1 class Foo: 2 def __init__(self,name,age): 3 self.name=name 4 self.age=age 5 6 def __str__(self): #用于打印 7 return '%s----%s'%(self.name,self.age) 8 9 obj=Foo('Jach',12) 10 #如果直接调用对象打印出来,会默认直接调用 __str__ 方法 11 #内部先把'print(obj)'默认转换成>>>'print(str(obj))' 获取其返回值 12 print(obj)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py Jach----12 Process finished with exit code 0
__add__
1 class Foo: 2 def __init__(self,name,age): 3 self.name=name 4 self.age=age 5 6 def __add__(self, other): 7 #self == obj('亚瑟',12) 8 #other==obj('后羿',23) 9 # return self.age+other.age 10 return Foo(self.name,other.age) 11 12 obj=Foo('亚瑟',12) 13 obj2=Foo('后羿',23) 14 15 r=obj+obj2 16 #两个相加时,会自动执行第一个对象的 __add__ 方法,并且把第二个参数当做参数传递进去 17 print(r,type(r)) 18 print(r.name) 19 print(r.age)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py <__main__.Foo object at 0x00000000024BD4E0> <class '__main__.Foo'> 亚瑟 23 Process finished with exit code 0
构造方法 对象被创造的时候自动触发 __init__
析构方法 对象被销毁的时候自动触发 __del__
1 class Foo: 2 def __init__(self,name,age): 3 self.name=name 4 self.age=age 5 6 def __add__(self, other): 7 #self == obj('亚瑟',12) 8 #other==obj('后羿',23) 9 # return self.age+other.age 10 return Foo(self.name,other.age) 11 def __del__(self): 12 print('析构方法,对象销毁时,自动执行') 13 14 obj=Foo('亚瑟',12) 15 obj2=Foo('后羿',23) 16 17 r=obj+obj2 18 #两个相加时,会自动执行第一个对象的 __add__ 方法,并且把第二个参数当做参数传递进去 19 print(r,type(r)) 20 print(r.name) 21 print(r.age)
运行结果:
"D:\Program Files (x86)\python36\python.exe" F:/python从入门到放弃/7.24/面向对象.py <__main__.Foo object at 0x0000000001EAD470> <class '__main__.Foo'> 亚瑟 23 析构方法,对象销毁时,自动执行 析构方法,对象销毁时,自动执行 析构方法,对象销毁时,自动执行 Process finished with exit code 0