1.环境准备
参考我的上两篇博客
(2)SpringBoot入门,整合Mybatis并使用Mybatis-Generator自动生成所需代码
2.在pom文件中引入Pagehelper分页插件
<!-- 分页插件 -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.5</version>
</dependency>
3.配置分页插件
打开application.properties,添加如下几行配置信息
#分页插件
pagehelper.helper-dialect=mysql
pagehelper.params=count=countSql
pagehelper.reasonable=true
pagehelper.support-methods-arguments=true
至此,pagehelper分页插件整合完毕,下面开始测试
4.测试
测试之前,我们先给上次创建的Person表增加几条数据
然后修改PersonController的代码
@Controller
public class PersonController {
@Autowired
private PersonService personService;
@GetMapping("/getAllPerson")
public String getAllPerson(Model model,@RequestParam(defaultValue = "1",value = "pageNum") Integer pageNum){
PageHelper.startPage(pageNum,5);
List<Person> list = personService.getAllPerson();
PageInfo<Person> pageInfo = new PageInfo<Person>(list);
model.addAttribute("pageInfo",pageInfo);
return "list";
}
}
其中:PageHelper.startPage(int PageNum,int PageSize):用来设置页面的位置和展示的数据条目数,我们设置每页展示5条数据。PageInfo用来封装页面信息,返回给前台界面。PageInfo中的一些我们需要用到的参数如下表:
PageInfo.list | 结果集 |
PageInfo.pageNum | 当前页码 |
PageInfo.pageSize | 当前页面显示的数据条目 |
PageInfo.pages | 总页数 |
PageInfo.total | 数据的总条目数 |
PageInfo.prePage | 上一页 |
PageInfo.nextPage | 下一页 |
PageInfo.isFirstPage | 是否为第一页 |
PageInfo.isLastPage | 是否为最后一页 |
PageInfo.hasPreviousPage | 是否有上一页 |
PageHelper.hasNextPage | 是否有下一页 |
下面处理返回到list界面的数据信息,依然使用thymeleaf模版,代码如下
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div align="center">
<table border="1">
<tr>
<th>id</th>
<th>name</th>
<th>sex</th>
<th>age</th>
</tr>
<tr th:each="person:${pageInfo.list}">
<td th:text="${person.id}"></td>
<td th:text="${person.name}"></td>
<td th:text="${person.sex}"></td>
<td th:text="${person.age}"></td>
</tr>
</table>
<p>当前 <span th:text="${pageInfo.pageNum}"></span> 页,总 <span th:text="${pageInfo.pages}"></span> 页,共 <span th:text="${pageInfo.total}"></span> 条记录</p>
<a th:href="@{/getAllPerson}">首页</a>
<a th:href="@{/getAllPerson(pageNum=${pageInfo.hasPreviousPage}?${pageInfo.prePage}:1)}">上一页</a>
<a th:href="@{/getAllPerson(pageNum=${pageInfo.hasNextPage}?${pageInfo.nextPage}:${pageInfo.pages})}">下一页</a>
<a th:href="@{/getAllPerson(pageNum=${pageInfo.pages})}">尾页</a>
</div>
</body>
</html>
打开浏览器查看效果
扫描二维码关注公众号,回复:
2327610 查看本文章
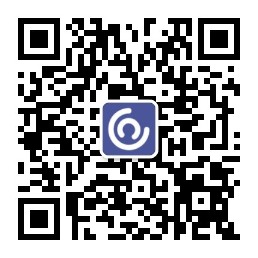
怎么样,是不是很简单。
5.扩展
在springboot的项目中,如果我们修改了前台界面,会发现刷新界面后没有效果,因此需要重启项目,这样很麻烦,简单两步教你搞定它:
(1)在配置文件中给thymeleaf添加如下配置:
spring.thymeleaf.cache=false
(2)在Intellij Idea按Ctrl+Shift+F9
之后在浏览器中刷新界面,即可显示出对页面的更改信息。
欢迎大家评论和转发,如有错误请提出,我会及时改正。