DZY has a sequence a[1..n]. It is a permutation of integers 1∼n.
Now he wants to perform two types of operations:
0lr: Sort a[l..r] in increasing order.
1lr: Sort a[l..r] in decreasing order.
After doing all the operations, he will tell you a position k, and ask you the value of a[k].
Input
First line contains t, denoting the number of testcases.
t testcases follow. For each testcase:
First line contains n,m. m is the number of operations.
Second line contains n space-separated integers a[1],a[2],⋯,a[n], the initial sequence. We ensure that it is a permutation of 1∼n.
Then m lines follow. In each line there are three integers opt,l,r to indicate an operation.
Last line contains k.
(1≤t≤50,1≤n,m≤100000,1≤k≤n,1≤l≤r≤n,opt∈{0,1}. Sum of n in all testcases does not exceed 150000. Sum of m in all testcases does not exceed 150000)
Output
For each testcase, output one line - the value of a[k] after performing all m operations.
Sample Input
1
6 3
1 6 2 5 3 4
0 1 4
1 3 6
0 2 4
3
Sample Output
5
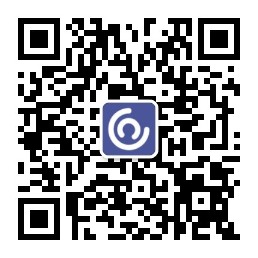
Hint
1 6 2 5 3 4 -> [1 2 5 6] 3 4 -> 1 2 [6 5 4 3] -> 1 [2 5 6] 4 3. At last
.
这次题目之前我们数据结构专题出过,所以比赛时候就直接交了;
考虑朴素算法:
每次对区间进行 sort,假设平均复杂度 NlogN
算上操作次数t. TLE是肯定的;
思路:
考虑用线段树进行维护,我们可以将大于 mid的设为1,小于mid 设为0 ,那么升序的时候将后面>mid设为1;降序则将前面设为1.每次二分答案,如果查询 [k,k]区间==1,说明在左区间;反之在右区间;
#include<bits/stdc++.h>
using namespace std;
const int maxn = 2e5+7;
int lazy[maxn<<2];
int s[maxn<<2];
int a[maxn];
int op[maxn];
int l[maxn];
int r[maxn];
int n,k;
int tot;
void pushDown( int rt , int L , int R ){
if( lazy[rt] != -1 ){
int mid = ( L + R ) >> 1 ;
s[rt<<1] = ( mid - L + 1 ) * lazy[rt] ;
s[rt<<1|1] = ( R - mid ) * lazy[rt];
lazy[rt<<1] = lazy[rt];
lazy[rt<<1|1] = lazy[rt];
lazy[rt] = -1;
}
return ;
}
void pushUp( int rt ){
s[rt] = s[rt<<1] + s[rt<<1|1];
return ;
}
void build( int l , int r , int rt , int v ){
lazy[rt] = -1;
if( l == r ){
s[rt] = ( a[l] > v ) ;
return ;
}
int mid = ( l + r ) >> 1 ;
build( l , mid , rt << 1 , v );
build( mid + 1 , r , rt << 1 | 1 , v);
pushUp(rt);
}
void update( int L , int R , int v , int l , int r , int rt ){
if( L <= l && R >= r ){
s[rt] = v * ( r - l + 1 );
lazy[rt] = v;
return;
}
pushDown(rt,l,r);
int mid = ( l + r ) >> 1;
if( L <= mid ) update( L , R , v , l , mid , rt << 1 );
if( R > mid ) update( L , R , v , mid + 1 , r , rt << 1 | 1);
pushUp(rt);
}
int query( int L , int R , int l , int r , int rt ){
if( L <= l && R >= r ){
return s[rt];
}
pushDown(rt,l,r);
int ans = 0 ;
int mid = ( l + r ) >> 1;
if( L <= mid ) ans += query( L , R , l , mid, rt << 1 );
if( R > mid ) ans += query( L , R , mid + 1, r , rt << 1 | 1 );
return ans ;
}
int main(){
int t;
cin>>t;
while(t--){
memset(s,0,sizeof(s));
memset(lazy,0,sizeof(lazy));
memset(a,0,sizeof(a));
memset(op,0,sizeof(op));
memset(l,0,sizeof(l));
memset(r,0,sizeof(r));
scanf("%d",&n);
int m ;
scanf("%d",&m);
for( int i = 1 ; i <= n ; i++ ){
scanf("%d",&a[i]);
}
for( int i = 0 ; i < m ; i++ ){
scanf("%d%d%d",&op[i],&l[i],&r[i]);
}
cin>>k;
int li = 1 , ri = n ;
while( li < ri ){
int mid = ( li + ri ) >> 1 ;
build( 1 , n , 1 , mid );
for( int i = 0 ; i < m ; i++ ){
int L = l[i];
int R = r[i];
int c = query( L , R , 1 , n , 1 );
update( L , R , 0 , 1 , n , 1 );
if( op[i] ){
if( L <= L + c - 1 ){
update( L , L + c - 1 , 1 , 1, n , 1 );
}
}else{
if( R - c + 1 <= R ){
update( R - c + 1 , R , 1 , 1 , n , 1 );
}
}
}
if( query( k , k , 1 , n , 1 ) ){
li = mid + 1 ;
}else ri = mid ;
}
printf("%d\n",li);
}
return 0 ;
}