实际开发中
一般数据库系统自带serial字段或者通过创建sequence实现自增字段
一般数据库系统自带serial字段或者通过创建sequence实现自增字段
•优势
•数据库自带,使用简单
•ID从小到大产生,便于识别
•占用空间小,4byte
•不足
•每个table都需要独立的sequence
•无法支持分布式部署
•修改维护较麻烦
UUID/GUID
在高并发分布式系统中,twitter在把存储系统从MySQL迁移到Cassandra的过程中由于Cassandra没有顺序ID生成机制,于是自己开发了一套全局唯一ID生成服务:Snowflake。
1 41位的时间序列(精确到毫秒,41位的长度可以使用69年)
2 10位的机器标识(10位的长度最多支持部署1024个节点)
3 12位的计数顺序号(12位的计数顺序号支持每个节点每毫秒产生4096个ID序号) 最高位是符号位,始终为0。
优点:高性能,低延迟;独立的应用;按时间有序。 缺点:需要独立的开发和部署。
原理如下:
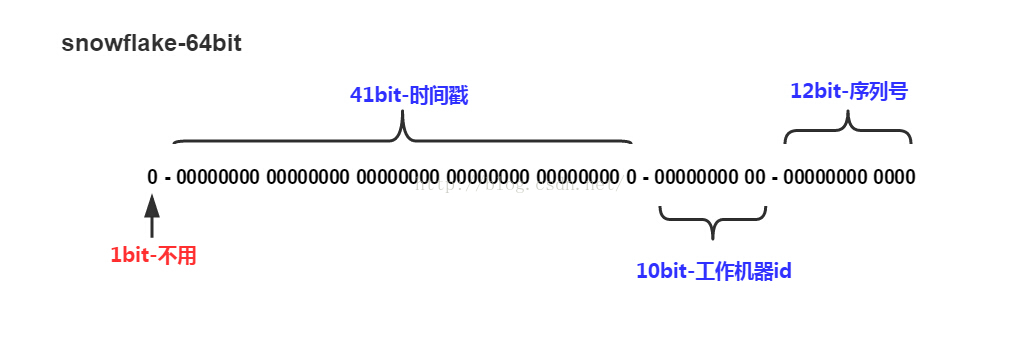
代码如下:
UUID/GUID
•大部分数据库系统都支持uuid
•优势
•可以实现跨表,跨库,甚至跨服务器的唯一标识
•多数据库之间数据汇总简单方便
•可以多服务器,分布式部署
•可以独立于数据库单独产生
•能够实现多种复制方案
•不足
•占用空间大,16byte
•产生的ID,可读性差,无法排序
在高并发分布式系统中,twitter在把存储系统从MySQL迁移到Cassandra的过程中由于Cassandra没有顺序ID生成机制,于是自己开发了一套全局唯一ID生成服务:Snowflake。
1 41位的时间序列(精确到毫秒,41位的长度可以使用69年)
2 10位的机器标识(10位的长度最多支持部署1024个节点)
3 12位的计数顺序号(12位的计数顺序号支持每个节点每毫秒产生4096个ID序号) 最高位是符号位,始终为0。
优点:高性能,低延迟;独立的应用;按时间有序。 缺点:需要独立的开发和部署。
原理如下:
代码如下:
public class IdWorker { private final long workerId; // 当前时间 private final static long twepoch = 1288834974657L; private long sequence = 0L; // 机器标识位数 private final static long workerIdBits = 4L; // 机器id最大值 public final static long maxWorkerId = -1L ^ -1L << workerIdBits; // 序列号位数 private final static long sequenceBits = 10L; // 机器id左移10位 private final static long workerIdShift = sequenceBits; // 时间毫秒左移14位 private final static long timestampLeftShift = sequenceBits + workerIdBits; public final static long sequenceMask = -1L ^ -1L << sequenceBits; private long lastTimestamp = -1L; public IdWorker(final long workerId) { super(); if (workerId > this.maxWorkerId || workerId < 0) { throw new IllegalArgumentException(String.format( "worker Id can't be greater than %d or less than 0", this.maxWorkerId)); } this.workerId = workerId; } public synchronized long nextId() { long timestamp = this.timeGen(); if (this.lastTimestamp == timestamp) { this.sequence = (this.sequence + 1) & this.sequenceMask; if (this.sequence == 0) { System.out.println("###########" + sequenceMask); timestamp = this.tilNextMillis(this.lastTimestamp); } } else { this.sequence = 0; } // 当前时间小于上次时间(异常) if (timestamp < this.lastTimestamp) { try { throw new Exception( String.format( "Clock moved backwards. Refusing to generate id for %d milliseconds", this.lastTimestamp - timestamp)); } catch (Exception e) { e.printStackTrace(); } } this.lastTimestamp = timestamp; // 或运算 long nextId = ((timestamp - twepoch << timestampLeftShift)) | (this.workerId << this.workerIdShift) | (this.sequence); System.out.println("timestamp:" + timestamp + ",timestampLeftShift:" + timestampLeftShift + ",nextId:" + nextId + ",workerId:" + workerId + ",sequence:" + sequence); return nextId; } private long tilNextMillis(final long lastTimestamp) { long timestamp = this.timeGen(); while (timestamp <= lastTimestamp) { timestamp = this.timeGen(); } return timestamp; } private long timeGen() { return System.currentTimeMillis(); } public static void main(String[] args) { IdWorker worker2 = new IdWorker(2); System.out.println(worker2.nextId()); } }