B:Battle Royale
Battle Royale games are the current trend in video games and Gamers Concealed Punching Circles (GCPC) is the most popular game of them all. The game takes place in an area that, for the sake of simplicity, can be thought of as a two-dimensional plane. Movement and positioning are a substantial part of the gameplay, but getting to a desired location can be dangerous. You are confident in your ability to handle the other players, however, while you are walking to your destination, there are two hazards posed by the game itself:
- The game zone is bounded by a blue circle. Outside of this circle, there is a deadly force field that would instantly take you out of the game.
- Inside the game zone, there is a red circle where you are exposed to artillery strikes. This circle is also too risky to enter.
You want to move from one spot on the map to another, but the direct path to your destination is blocked by the red circle, so you need to find a way around it. Can you find the shortest path that avoids all hazards by never leaving the blue or entering the red circle? Touching the boundaries of the circles is fine, as long as you do not cross them.
Input Format
The input consists of:
- one line with two integers xc, yc specifying your current location;
- one line with two integers xd, yd specifying your destination;
- one line with three integers xb,yb,rb specifying the center and radius of the blue circle;
- one line with three integers xr , yr, rr specifying the center and radius of the red circle.
All coordinates have an absolute value of at most 1000, and 1≤rb,rr≤1000. The red circle is strictly inside the blue circle. Your current location and destination are strictly inside the blue circle and strictly outside of the red circle, and the direct path between them is blocked by the red circle.
Output Format
Output the length of the shortest path that does not leave the blue or enter the red circle. The output must be accurate up to a relative or absolute error (whichever is lower) of 10−7.
本题答案不唯一,符合要求的答案均正确
样例输入
0 0 10 0 0 0 1000 5 0 2
样例输出
10.8112187742
求两点与圆的切线,加一段弧长,如图
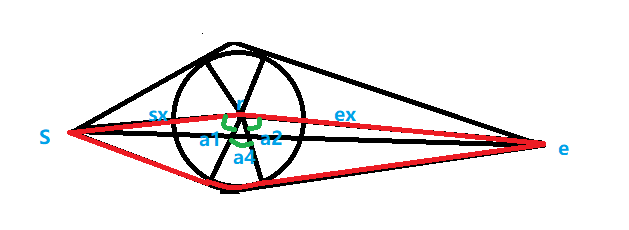
#include <cstring> #include <cstdio> #include <vector> #include <queue> #include <stack> #include <cstdlib> #include <iomanip> #include <cmath> #include <cassert> #include <ctime> #include <map> #include <set> using namespace std; #pragma comment(linker, "/stck:1024000000,1024000000") #pragma GCC diagnostic error "-std=c++11" #define lowbit(x) (x&(-x)) #define max(x,y) (x>=y?x:y) #define min(x,y) (x<=y?x:y) #define MAX 100000000000000000 #define MOD 1000000007 #define pi acos(-1.0) #define ei exp(1) #define PI 3.1415926535897932384626433832 #define ios() ios::sync_with_stdio(true) #define INF 0x3f3f3f3f #define mem(a) (memset(a,0,sizeof(a))) typedef long long ll; int main() { double sa,sb,ea,eb,ba,bb,ra,rb,br,rr; scanf("%lf%lf%lf%lf%lf%lf%lf%lf%lf%lf",&sa,&sb,&ea,&eb,&ba,&bb,&br,&ra,&rb,&rr); double sx=sqrt((sa-ra)*(sa-ra)+(sb-rb)*(sb-rb)); double ex=sqrt((ea-ra)*(ea-ra)+(eb-rb)*(eb-rb)); double se=sqrt((ea-sa)*(ea-sa)+(eb-sb)*(eb-sb)); double x1=sqrt((sa-ra)*(sa-ra)+(sb-rb)*(sb-rb)-rr*rr); double x2=sqrt((ea-ra)*(ea-ra)+(eb-rb)*(eb-rb)-rr*rr); double a1=acos(rr/sx); double a2=acos(rr/ex); double a3=acos((sx*sx+ex*ex-se*se)/(2*sx*ex)); double a4=a3-a2-a1; double x3=a4*rr; printf("%.10lf",x1+x2+x3); return 0; }
C. Coolest Ski Route
John loves winter. Every skiing season he goes heli-skiing with his friends. To do so, they rent a helicopter that flies them directly to any mountain in the Alps. From there they follow the picturesque slopes through the untouched snow.
Of course they want to ski on only the best snow, in the best weather they can get. For this they use a combined condition measure and for any given day, they rate all the available slopes.
Can you help them find the most awesome route?
Input Format
The input consists of:
- one line with two integers n (2≤n≤1000) and m (1≤m≤5000),where nnn is the number of (1-indexed) connecting points between slopes and mmm is the number of slopes.
- m lines, each with three integers s,t,c (1≤s,t≤n,1≤c≤100) representing a slopefrom point s to point t with condition measure c.
Points without incoming slopes are mountain tops with beautiful scenery, points without outgoing slopes are valleys. The helicopter can land on every connecting point, so the friends can start and end their tour at any point they want. All slopes go downhill, so regardless of where they start, they cannot reach the same point again after taking any of the slopes.
Output Format
Output a single number nnn that is the maximum sum of condition measures along a path that the friends could take
Hint
样例输入1
5 5 1 2 15 2 3 12 1 4 17 4 2 11 5 4 9
样例输出1
40
样例输入2
6 6 1 2 2 4 5 2 2 3 3 1 3 2 5 6 2 1 2 4
样例输出2
7
#include <iostream> #include <algorithm> #include <cstring> #include <cstdio> #include <vector> #include <queue> #include <stack> #include <cstdlib> #include <iomanip> #include <cmath> #include <cassert> #include <ctime> #include <map> #include <set> using namespace std; #pragma comment(linker, "/stck:1024000000,1024000000") #pragma GCC diagnostic error "-std=c++11" #define lowbit(x) (x&(-x)) #define max(x,y) (x>=y?x:y) #define min(x,y) (x<=y?x:y) #define MAX 100000000000000000 #define MOD 1000000007 #define pi acos(-1.0) #define ei exp(1) #define PI 3.1415926535897932384626433832 #define ios() ios::sync_with_stdio(true) #define INF 0x3f3f3f3f #define mem(a) (memset(a,0,sizeof(a))) typedef long long ll; vector<pair<int,int> >v[1006]; typedef pair<int,int> p; int n,m,dis[1006]; void priority_queue_dijkstra() { memset(dis,0,sizeof(dis)); priority_queue<p,vector<p>,less<p> >q; for(int i=1;i<=n;i++) q.push(make_pair(0,i)); while(!q.empty()) { p t=q.top(); q.pop(); if(dis[t.second]!=t.first) continue; for(int i=0;i<v[t.second].size();i++) { if(dis[v[t.second][i].first]<t.first+v[t.second][i].second) { dis[v[t.second][i].first]=t.first+v[t.second][i].second; q.push(make_pair(dis[v[t.second][i].first],v[t.second][i].first)); } } } } int main() { scanf("%d%d",&n,&m); for(int i=0,x,y,z;i<m;i++) { scanf("%d%d%d",&x,&y,&z); v[x].push_back({y,z}); } int inf=-INF; priority_queue_dijkstra(); for(int i=1;i<=n;i++) inf=max(inf,dis[i]); printf("%d\n",inf); return 0; }
D. Down the Pyramid
Do you like number pyramids? Given a number sequence that represents the base, you are usually supposed to build the rest of the "pyramid" bottom-up: For each pair of adjacent numbers, you would compute their sum and write it down above them. For example, given the base sequence [1,2,3], the sequence directly above it would be [3,5], and the top of the pyramid would be [8]:
However, I am not interested in completing the pyramid – instead, I would much rather go underground. Thus, for a sequence of nnn non-negative integers, I will write down a sequence of n+1 non-negative integers below it such that each number in the original sequence is the sum of the two numbers I put below it. However, there may be several possible sequences or perhaps even none at all satisfying this condition. So, could you please tell me how many sequences there are for me to choose from?
Input Format
The input consists of:
- one line with the integer n (1≤n≤106), the length of the base sequence.
- one line with n integers a1,⋯,an (0≤ai≤108), forming the base sequence.
Output Format
Output a single integer, the number of non-negative integer sequences that would have the input sequence as the next level in a number pyramid.
样例输入1
6 12 5 7 7 8 4
样例输出1
2
样例输入2
3 10 1000 100
样例输出2
0
#include <iostream> #include <algorithm> #include <cstring> #include <cstdio> #include <vector> #include <queue> #include <stack> #include <cstdlib> #include <iomanip> #include <cmath> #include <cassert> #include <ctime> #include <map> #include <set> using namespace std; #pragma comment(linker, "/stck:1024000000,1024000000") #pragma GCC diagnostic error "-std=c++11" #define lowbit(x) (x&(-x)) #define max(x,y) (x>=y?x:y) #define min(x,y) (x<=y?x:y) #define MAX 100000000000000000 #define MOD 1000000007 #define pi acos(-1.0) #define ei exp(1) #define PI 3.1415926535897932384626433832 #define ios() ios::sync_with_stdio(true) #define INF 0x3f3f3f3f #define mem(a) (memset(a,0,sizeof(a))) typedef long long ll; int ansl=-INF,ansr=INF; int n; int main() { scanf("%d",&n); int cnt=0; for(int i=1,x;i<=n;i++) { scanf("%d",&x); cnt=cnt*-1+x; if(i&1) ansr=min(ansr,cnt); else ansl=max(ansl,cnt*-1); } if(ansr<ansl) printf("0\n"); else if(ansl<0 && ansr<0) printf("0\n"); else if(ansl<0 && ansr>=0) printf("%d\n",ansr+1); else printf("%d\n",ansr-ansl+1); return 0; }