一、 JavaScript的组成
ECMAScript:JavaScript的语法标准
DOM:文档对象模型
BOM:浏览器对象模型
二、 BOM
全称 Browser Object Model 浏览器对象模型
window对象是JS中的顶级对象
所有定义在全局作用域中的变量、函数都会变成window对象的属性和方法
window对象下的属性和方法调用的时候可以省略window
三、window的方法
1. window.open(url,target,param)
url要打开的地址
target新窗口的位置
_blank _self _parent(父框架)
param心窗口的一些设置
2. window。close()关闭窗口
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>window-load</title>
<!--js-->
<script>
//window方法anload,在页面加载完成之后自动执行
window.onload=function(){
var show=document.getElementById("showDiv");
alert(show);
show.innerHTML="<h1>js动态写入</h1>";
}
</script>
</head>
<body>
<div id="showDiv"></div>
</body>
</html>
四、 js获取html标签对象的方式
1. document.getElementById 通过id获取
2. document.getElementsByTagName 通过标签名获取,返回值是数组
如var div = document.get…Name(“div”);
var div1 =div[0];//获取数组中的第一个
3. documen.getElementsByClassName
通过class的值来获取,为标签添加class,
根据class的取值获取标签,返回值是数组
/*鼠标事件,获取鼠标的位置*/
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style>
#main{
width:500px;
height:500px;
background-color: yellow;
}
</style>
</head>
<body>
<h2 id="show_index">000:000</h2>
<div id="main">
<img width:"100px" height="100px" src="img/2.jpg" id="beauty" />
</div>
<script>
var main = document.getElementById("main");
var showIndex=document.getElementById("show_index");
var girl=document.getElementById("beauty");
//绑定鼠标事件
// main.onmousedown
// main.onmouseup
//匿名函数属于另一个区域,event是发生在当前DOM中的事件,需要通过形参传入匿名函数使用
main.onmousemove=function(event){
var x=event.clientX;
var y=event.clientY;
showIndex.innerHTML=x+":"+y;
main.style.position="absolute";
girl.style.position="absolute";
girl.style.left=(x-90)+"px";
girl.style.top=(y-110)+"px";
girl.style.width=(x-100)+100+"px";
girl.style.height=(y-100)+100+"px";
}
</script>
</body>
</html>
五、 动态灵活的onclick设置方式
xx.onclick = function(){
//点击事件的代码
}
六、 定时器
1. 循环执行(间隔多长时间反复执行)
setInterval(code,interval)
参数1:执行的代码,可以使function函数
参数2:间隔的时间,毫秒为单位
2. 取消循环执行定时器
clearInterval(timerId);
/*定时器反复*/
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body background="img/ice.png" >
<script>
setInterval(recy,2000);
function recy() {
alert("小样的,反不死你!");
} </script>
</body>
</html>
七、编辑器
1. sublineText
2. atom(功能最强大的文档编辑器,html,css,js,markdown)
3. 文档编辑语言:markdown
八、 location
window.location
相当于浏览器的地址栏
通俗:在js代码中想要获取访问网址的主机,端口号,协议,搜索字段等,通过location获取
用户统计分析(产品数据统计)
九、navigator
window.navigatory
获取客户端的信息,如浏览器的型号,操作系统型号
十、 历史记录history
forward,back前进,返回等
十一、DOM文档对象模型
document
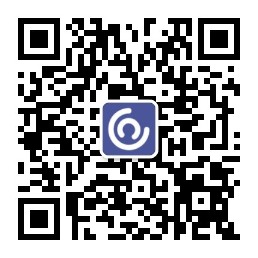
十二、DOM可以做什么
找对象(元素)
设置元素的属性,设置元素的样式
img.src a.href属性
xx.style.position样式
动态创建的删除元素
事件一触发响应
事件源(时间的触发者)
事件名称
事件响应程序
练习:
1.点击图片,更换背景图片。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style>
.box {
height: 180px;
padding-top: 30px;
text-align: center;
}
body {
background: url(img/10.jpg) no-repeat;
}
</style>
</head>
<body>
<div class="box">
<img src="img/10.jpg" width="200px"/>
<img src="img/20.jpg"width="200px" />
<img src="img/30.jpg" width="200px"/>
<img src="img/40.jpg" width="200px"/>
<img src="img/50.jpg" width="200px"/>
</div>
<script>
var box=document.getElementsByTagName("div")[0];
var body=document.body;
var Arr=box.children;
for(var i=0;i<Arr.length;i++)
{
Arr[i].index=i;
Arr[i].onclick=function(){
body.style.backgroundImage = "url("+this.src+")";
}
}
</script>
</body>
</html>
2.隔行变色的表格(鼠标放上去行会变色)
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
* {
padding: 0;
margin: 0;
text-align: center;
}
.wrap {
width: 500px;
margin: 100px auto 0;
}
table {
border-collapse: collapse;
border-spacing: 0;
border: 1px solid #c0c0c0;
width: 500px;
}
th,
td {
border: 1px solid #d0d0d0;
color: #404060;
padding: 10px;
}
th {
background-color: #09c;
font: bold 16px "微软雅黑";
color: #fff;
}
td {
font: 14px "微软雅黑";
}
tbody tr {
background-color: #f0f0f0;
cursor: pointer;
}
.current {
background-color: red!important;
}
</style>
</head>
<body>
<div class="wrap">
<table>
<thead>
<tr>
<th>序号</th>
<th>姓名</th>
<th>课程</th>
<th>成绩</th>
</tr>
</thead>
<tbody id="target">
<tr>
<td>
1
</td>
<td>吕不韦</td>
<td>语文</td>
<td>100</td>
</tr>
<tr>
<td>
2
</td>
<td>吕布</td>
<td>日语</td>
<td>100</td>
</tr>
<tr>
<td>
3
</td>
<td>吕蒙</td>
<td>营销学</td>
<td>100</td>
</tr>
<tr>
<td>
4
</td>
<td>吕尚</td>
<td>数学</td>
<td>100</td>
</tr>
<tr>
<td>
5
</td>
<td>吕雉</td>
<td>英语</td>
<td>100</td>
</tr>
<tr>
<td>
6
</td>
<td>吕超</td>
<td>体育</td>
<td>100</td>
</tr>
</tbody>
</table>
</div>
<script>
//需求:让tr各行变色,鼠标放入tr中,高亮显示。
//1.隔行变色。
var tbody = document.getElementById("target");
var trArr = tbody.children;
//循环判断并各行赋值属性(背景色)
for(var i=0;i<trArr.length;i++){
if(i%2!==0){
trArr[i].style.backgroundColor = "#a3a3a3";
}else{
trArr[i].style.backgroundColor = "#ccc";
}
//鼠标进入高亮显示
//难点:鼠标移开的时候要回复原始颜色。
//计数器(进入tr之后,立刻记录颜色,然后移开的时候使用记录好的颜色)
var color = "";
trArr[i].onmouseover = function () {
//赋值颜色之前,先记录颜色
color = this.style.backgroundColor;
this.style.backgroundColor = "#fff";
}
trArr[i].onmouseout = function () {
this.style.backgroundColor = color;
}
}
</script>
</body>
</html>