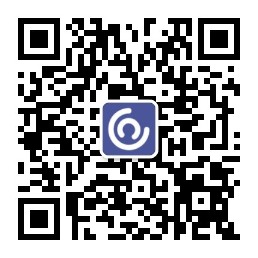
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
# Data for plotting
t = np.arange(0.0, 2.0, 0.01)
s = 1 + np.sin(2 * np.pi * t)
# Note that using plt.subplots below is equivalent to using
# fig = plt.figure() and then ax = fig.add_subplot(111)
fig, ax = plt.subplots()
ax.plot(t, s)
ax.set(xlabel='time (s)', ylabel='voltage (mV)',
title='About as simple as it gets, folks')
ax.grid()
fig.savefig("test.png")
plt.show()
Multiple subplots¶
Simple demo with multiple subplots.

import numpy as np
import matplotlib.pyplot as plt
x1 = np.linspace(0.0, 5.0)
x2 = np.linspace(0.0, 2.0)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
y2 = np.cos(2 * np.pi * x2)
plt.subplot(2, 1, 1)
plt.plot(x1, y1, 'o-')
plt.title('A tale of 2 subplots')
plt.ylabel('Damped oscillation')
plt.subplot(2, 1, 2)
plt.plot(x2, y2, '.-')
plt.xlabel('time (s)')
plt.ylabel('Undamped')
plt.show()
Image Demo¶
Many ways to plot images in Matplotlib.
The most common way to plot images in Matplotlib is with imshow. The following examples demonstrate much of the functionality of imshow and the many images you can create.
First we’ll generate a simple bivariate normal distribution.
delta = 0.025
x = y = np.arange(-3.0, 3.0, delta)
X, Y = np.meshgrid(x, y)
Z1 = np.exp(-X**2 - Y**2)
Z2 = np.exp(-(X - 1)**2 - (Y - 1)**2)
Z = (Z1 - Z2) * 2
im = plt.imshow(Z, interpolation='bilinear', cmap=cm.RdYlGn,
origin='lower', extent=[-3, 3, -3, 3],
vmax=abs(Z).max(), vmin=-abs(Z).max())
plt.show()

It is also possible to show images of pictures.
# A sample image
with cbook.get_sample_data('ada.png') as image_file:
image = plt.imread(image_file)
fig, ax = plt.subplots()
ax.imshow(image)
ax.axis('off') # clear x- and y-axes
# And another image
w, h = 512, 512
with cbook.get_sample_data('ct.raw.gz', asfileobj=True) as datafile:
s = datafile.read()
A = np.fromstring(s, np.uint16).astype(float).reshape((w, h))
A /= A.max()
fig, ax = plt.subplots()
extent = (0, 25, 0, 25)
im = ax.imshow(A, cmap=plt.cm.hot, origin='upper', extent=extent)
markers = [(15.9, 14.5), (16.8, 15)]
x, y = zip(*markers)
ax.plot(x, y, 'o')
ax.set_title('CT density')
plt.show()
Interpolating images¶
It is also possible to interpolate images before displaying them. Be careful, as this may manipulate the way your data looks, but it can be helpful for achieving the look you want. Below we’ll display the same (small) array, interpolated with three different interpolation methods.
The center of the pixel at A[i,j] is plotted at i+0.5, i+0.5. If you are using interpolation=’nearest’, the region bounded by (i,j) and (i+1,j+1) will have the same color. If you are using interpolation, the pixel center will have the same color as it does with nearest, but other pixels will be interpolated between the neighboring pixels.
Earlier versions of matplotlib (<0.63) tried to hide the edge effects from you by setting the view limits so that they would not be visible. A recent bugfix in antigrain, and a new implementation in the matplotlib._image module which takes advantage of this fix, no longer makes this necessary. To prevent edge effects, when doing interpolation, the matplotlib._image module now pads the input array with identical pixels around the edge. e.g., if you have a 5x5 array with colors a-y as below:
a b c d e
f g h i j
k l m n o
p q r s t
u v w x y
the _image module creates the padded array,:
a a b c d e e
a a b c d e e
f f g h i j j
k k l m n o o
p p q r s t t
o u v w x y y
o u v w x y y
does the interpolation/resizing, and then extracts the central region. This allows you to plot the full range of your array w/o edge effects, and for example to layer multiple images of different sizes over one another with different interpolation methods - see examples/layer_images.py. It also implies a performance hit, as this new temporary, padded array must be created. Sophisticated interpolation also implies a performance hit, so if you need maximal performance or have very large images, interpolation=’nearest’ is suggested.
A = np.random.rand(5, 5)
fig, axs = plt.subplots(1, 3, figsize=(10, 3))
for ax, interp in zip(axs, ['nearest', 'bilinear', 'bicubic']):
ax.imshow(A, interpolation=interp)
ax.set_title(interp.capitalize())
ax.grid(True)
plt.show()

You can specify whether images should be plotted with the array origin x[0,0] in the upper left or lower right by using the origin parameter. You can also control the default setting image.origin in your matplotlibrc file
x = np.arange(120).reshape((10, 12))
interp = 'bilinear'
fig, axs = plt.subplots(nrows=2, sharex=True, figsize=(3, 5))
axs[0].set_title('blue should be up')
axs[0].imshow(x, origin='upper', interpolation=interp)
axs[1].set_title('blue should be down')
axs[1].imshow(x, origin='lower', interpolation=interp)
plt.show()

Finally, we’ll show an image using a clip path.
delta = 0.025
x = y = np.arange(-3.0, 3.0, delta)
X, Y = np.meshgrid(x, y)
Z1 = np.exp(-X**2 - Y**2)
Z2 = np.exp(-(X - 1)**2 - (Y - 1)**2)
Z = (Z1 - Z2) * 2
path = Path([[0, 1], [1, 0], [0, -1], [-1, 0], [0, 1]])
patch = PathPatch(path, facecolor='none')
fig, ax = plt.subplots()
ax.add_patch(patch)
im = ax.imshow(Z, interpolation='bilinear', cmap=cm.gray,
origin='lower', extent=[-3, 3, -3, 3],
clip_path=patch, clip_on=True)
im.set_clip_path(patch)
plt.show()

Demo of the histogram (hist) function with a few features¶
In addition to the basic histogram, this demo shows a few optionalfeatures:
- Setting the number of data bins
- The
normed
flag, which normalizes bin heights so that theintegral of the histogram is 1. The resulting histogram is anapproximation of the probability density function.- Setting the face color of the bars
- Setting the opacity (alpha value).
Selecting different bin counts and sizes can significantly affect theshape of a histogram. The Astropy docs have a great section on how toselect these parameters:http://docs.astropy.org/en/stable/visualization/histogram.html
import matplotlib
import numpy as np
import matplotlib.pyplot as plt
np.random.seed(19680801)
# example data
mu = 100 # mean of distribution
sigma = 15 # standard deviation of distribution
x = mu + sigma * np.random.randn(437)
num_bins = 50
fig, ax = plt.subplots()
# the histogram of the data
n, bins, patches = ax.hist(x, num_bins, density=1)
# add a 'best fit' line
y = ((1 / (np.sqrt(2 * np.pi) * sigma)) *
np.exp(-0.5 * (1 / sigma * (bins - mu))**2))
ax.plot(bins, y, '--')
ax.set_xlabel('Smarts')
ax.set_ylabel('Probability density')
ax.set_title(r'Histogram of IQ: $\mu=100$, $\sigma=15$')
# Tweak spacing to prevent clipping of ylabel
fig.tight_layout()
plt.show()

3D surface (color map)¶
Demonstrates plotting a 3D surface colored with the coolwarm color map.The surface is made opaque by using antialiased=False.
Also demonstrates using the LinearLocator and custom formatting for thez axis tick labels.

from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from matplotlib import cm
from matplotlib.ticker import LinearLocator, FormatStrFormatter
import numpy as np
fig = plt.figure()
ax = fig.gca(projection='3d')
# Make data.
X = np.arange(-5, 5, 0.25)
Y = np.arange(-5, 5, 0.25)
X, Y = np.meshgrid(X, Y)
R = np.sqrt(X**2 + Y**2)
Z = np.sin(R)
# Plot the surface.
surf = ax.plot_surface(X, Y, Z, cmap=cm.coolwarm,
linewidth=0, antialiased=False)
# Customize the z axis.
ax.set_zlim(-1.01, 1.01)
ax.zaxis.set_major_locator(LinearLocator(10))
ax.zaxis.set_major_formatter(FormatStrFormatter('%.02f'))
# Add a color bar which maps values to colors.
fig.colorbar(surf, shrink=0.5, aspect=5)
plt.show()
Streamplot¶
A stream plot, or streamline plot, is used to display 2D vector fields. This example shows a few features of the streamplot function:
- Varying the color along a streamline.
- Varying the density of streamlines.
- Varying the line width along a streamline.
- Controlling the starting points of streamlines.
- Streamlines skipping masked regions and NaN values.

import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
w = 3
Y, X = np.mgrid[-w:w:100j, -w:w:100j]
U = -1 - X**2 + Y
V = 1 + X - Y**2
speed = np.sqrt(U*U + V*V)
fig = plt.figure(figsize=(7, 9))
gs = gridspec.GridSpec(nrows=3, ncols=2, height_ratios=[1, 1, 2])
# Varying density along a streamline
ax0 = fig.add_subplot(gs[0, 0])
ax0.streamplot(X, Y, U, V, density=[0.5, 1])
ax0.set_title('Varying Density')
# Varying color along a streamline
ax1 = fig.add_subplot(gs[0, 1])
strm = ax1.streamplot(X, Y, U, V, color=U, linewidth=2, cmap='autumn')
fig.colorbar(strm.lines)
ax1.set_title('Varying Color')
# Varying line width along a streamline
ax2 = fig.add_subplot(gs[1, 0])
lw = 5*speed / speed.max()
ax2.streamplot(X, Y, U, V, density=0.6, color='k', linewidth=lw)
ax2.set_title('Varying Line Width')
# Controlling the starting points of the streamlines
seed_points = np.array([[-2, -1, 0, 1, 2, -1], [-2, -1, 0, 1, 2, 2]])
ax3 = fig.add_subplot(gs[1, 1])
strm = ax3.streamplot(X, Y, U, V, color=U, linewidth=2,
cmap='autumn', start_points=seed_points.T)
fig.colorbar(strm.lines)
ax3.set_title('Controlling Starting Points')
# Displaying the starting points with blue symbols.
ax3.plot(seed_points[0], seed_points[1], 'bo')
ax3.axis((-w, w, -w, w))
# Create a mask
mask = np.zeros(U.shape, dtype=bool)
mask[40:60, 40:60] = True
U[:20, :20] = np.nan
U = np.ma.array(U, mask=mask)
ax4 = fig.add_subplot(gs[2:, :])
ax4.streamplot(X, Y, U, V, color='r')
ax4.set_title('Streamplot with Masking')
ax4.imshow(~mask, extent=(-w, w, -w, w), alpha=0.5,
interpolation='nearest', cmap='gray', aspect='auto')
ax4.set_aspect('equal')
plt.tight_layout()
plt.show()
Barchart Demo¶
Bar charts of many shapes and sizes with Matplotlib.
Bar charts are useful for visualizing counts, or summary statistics with error bars. These examples show a few ways to do this with Matplotlib.
# Credit: Josh Hemann
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.ticker import MaxNLocator
from collections import namedtuple
n_groups = 5
means_men = (20, 35, 30, 35, 27)
std_men = (2, 3, 4, 1, 2)
means_women = (25, 32, 34, 20, 25)
std_women = (3, 5, 2, 3, 3)
fig, ax = plt.subplots()
index = np.arange(n_groups)
bar_width = 0.35
opacity = 0.4
error_config = {'ecolor': '0.3'}
rects1 = ax.bar(index, means_men, bar_width,
alpha=opacity, color='b',
yerr=std_men, error_kw=error_config,
label='Men')
rects2 = ax.bar(index + bar_width, means_women, bar_width,
alpha=opacity, color='r',
yerr=std_women, error_kw=error_config,
label='Women')
ax.set_xlabel('Group')
ax.set_ylabel('Scores')
ax.set_title('Scores by group and gender')
ax.set_xticks(index + bar_width / 2)
ax.set_xticklabels(('A', 'B', 'C', 'D', 'E'))
ax.legend()
fig.tight_layout()
plt.show()

This example comes from an application in which grade school gym teachers wanted to be able to show parents how their child did across a handful of fitness tests, and importantly, relative to how other children did. To extract the plotting code for demo purposes, we’ll just make up some data for little Johnny Doe…
Student = namedtuple('Student', ['name', 'grade', 'gender'])
Score = namedtuple('Score', ['score', 'percentile'])
# GLOBAL CONSTANTS
testNames = ['Pacer Test', 'Flexed Arm\n Hang', 'Mile Run', 'Agility',
'Push Ups']
testMeta = dict(zip(testNames, ['laps', 'sec', 'min:sec', 'sec', '']))
def attach_ordinal(num):
"""helper function to add ordinal string to integers
1 -> 1st
56 -> 56th
"""
suffixes = dict((str(i), v) for i, v in
enumerate(['th', 'st', 'nd', 'rd', 'th',
'th', 'th', 'th', 'th', 'th']))
v = str(num)
# special case early teens
if v in {'11', '12', '13'}:
return v + 'th'
return v + suffixes[v[-1]]
def format_score(scr, test):
"""
Build up the score labels for the right Y-axis by first
appending a carriage return to each string and then tacking on
the appropriate meta information (i.e., 'laps' vs 'seconds'). We
want the labels centered on the ticks, so if there is no meta
info (like for pushups) then don't add the carriage return to
the string
"""
md = testMeta[test]
if md:
return '{0}\n{1}'.format(scr, md)
else:
return scr
def format_ycursor(y):
y = int(y)
if y < 0 or y >= len(testNames):
return ''
else:
return testNames[y]
def plot_student_results(student, scores, cohort_size):
# create the figure
fig, ax1 = plt.subplots(figsize=(9, 7))
fig.subplots_adjust(left=0.115, right=0.88)
fig.canvas.set_window_title('Eldorado K-8 Fitness Chart')
pos = np.arange(len(testNames))
rects = ax1.barh(pos, [scores[k].percentile for k in testNames],
align='center',
height=0.5, color='m',
tick_label=testNames)
ax1.set_title(student.name)
ax1.set_xlim([0, 100])
ax1.xaxis.set_major_locator(MaxNLocator(11))
ax1.xaxis.grid(True, linestyle='--', which='major',
color='grey', alpha=.25)
# Plot a solid vertical gridline to highlight the median position
ax1.axvline(50, color='grey', alpha=0.25)
# set X-axis tick marks at the deciles
cohort_label = ax1.text(.5, -.07, 'Cohort Size: {0}'.format(cohort_size),
horizontalalignment='center', size='small',
transform=ax1.transAxes)
# Set the right-hand Y-axis ticks and labels
ax2 = ax1.twinx()
scoreLabels = [format_score(scores[k].score, k) for k in testNames]
# set the tick locations
ax2.set_yticks(pos)
# make sure that the limits are set equally on both yaxis so the
# ticks line up
ax2.set_ylim(ax1.get_ylim())
# set the tick labels
ax2.set_yticklabels(scoreLabels)
ax2.set_ylabel('Test Scores')
ax2.set_xlabel(('Percentile Ranking Across '
'{grade} Grade {gender}s').format(
grade=attach_ordinal(student.grade),
gender=student.gender.title()))
rect_labels = []
# Lastly, write in the ranking inside each bar to aid in interpretation
for rect in rects:
# Rectangle widths are already integer-valued but are floating
# type, so it helps to remove the trailing decimal point and 0 by
# converting width to int type
width = int(rect.get_width())
rankStr = attach_ordinal(width)
# The bars aren't wide enough to print the ranking inside
if (width < 5):
# Shift the text to the right side of the right edge
xloc = width + 1
# Black against white background
clr = 'black'
align = 'left'
else:
# Shift the text to the left side of the right edge
xloc = 0.98*width
# White on magenta
clr = 'white'
align = 'right'
# Center the text vertically in the bar
yloc = rect.get_y() + rect.get_height()/2.0
label = ax1.text(xloc, yloc, rankStr, horizontalalignment=align,
verticalalignment='center', color=clr, weight='bold',
clip_on=True)
rect_labels.append(label)
# make the interactive mouse over give the bar title
ax2.fmt_ydata = format_ycursor
# return all of the artists created
return {'fig': fig,
'ax': ax1,
'ax_right': ax2,
'bars': rects,
'perc_labels': rect_labels,
'cohort_label': cohort_label}
student = Student('Johnny Doe', 2, 'boy')
scores = dict(zip(testNames,
(Score(v, p) for v, p in
zip(['7', '48', '12:52', '17', '14'],
np.round(np.random.uniform(0, 1,
len(testNames))*100, 0)))))
cohort_size = 62 # The number of other 2nd grade boys
arts = plot_student_results(student, scores, cohort_size)
plt.show()

Basic pie chart¶
Demo of a basic pie chart plus a few additional features.
In addition to the basic pie chart, this demo shows a few optional features:
- slice labels
- auto-labeling the percentage
- offsetting a slice with “explode”
- drop-shadow
- custom start angle
Note about the custom start angle:
The default startangle
is 0, which would start the “Frogs” slice on thepositive x-axis. This example sets startangle = 90
such that everything isrotated counter-clockwise by 90 degrees, and the frog slice starts on thepositive y-axis.

import matplotlib.pyplot as plt
# Pie chart, where the slices will be ordered and plotted counter-clockwise:
labels = 'Frogs', 'Hogs', 'Dogs', 'Logs'
sizes = [15, 30, 45, 10]
explode = (0, 0.1, 0, 0) # only "explode" the 2nd slice (i.e. 'Hogs')
fig1, ax1 = plt.subplots()
ax1.pie(sizes, explode=explode, labels=labels, autopct='%1.1f%%',
shadow=True, startangle=90)
ax1.axis('equal') # Equal aspect ratio ensures that pie is drawn as a circle.
plt.show()
Scatter Demo2¶
Demo of scatter plot with varying marker colors and sizes.

import numpy as np
import matplotlib.pyplot as plt
import matplotlib.cbook as cbook
# Load a numpy record array from yahoo csv data with fields date, open, close,
# volume, adj_close from the mpl-data/example directory. The record array
# stores the date as an np.datetime64 with a day unit ('D') in the date column.
with cbook.get_sample_data('goog.npz') as datafile:
price_data = np.load(datafile)['price_data'].view(np.recarray)
price_data = price_data[-250:] # get the most recent 250 trading days
delta1 = np.diff(price_data.adj_close) / price_data.adj_close[:-1]
# Marker size in units of points^2
volume = (15 * price_data.volume[:-2] / price_data.volume[0])**2
close = 0.003 * price_data.close[:-2] / 0.003 * price_data.open[:-2]
fig, ax = plt.subplots()
ax.scatter(delta1[:-1], delta1[1:], c=close, s=volume, alpha=0.5)
ax.set_xlabel(r'$\Delta_i$', fontsize=15)
ax.set_ylabel(r'$\Delta_{i+1}$', fontsize=15)
ax.set_title('Volume and percent change')
ax.grid(True)
fig.tight_layout()
plt.show()
Polar Demo¶
Demo of a line plot on a polar axis.

import numpy as np
import matplotlib.pyplot as plt
r = np.arange(0, 2, 0.01)
theta = 2 * np.pi * r
ax = plt.subplot(111, projection='polar')
ax.plot(theta, r)
ax.set_rmax(2)
ax.set_rticks([0.5, 1, 1.5, 2]) # Less radial ticks
ax.set_rlabel_position(-22.5) # Move radial labels away from plotted line
ax.grid(True)
ax.set_title("A line plot on a polar axis", va='bottom')
plt.show()
Subplot example¶
Many plot types can be combined in one figure to createpowerful and flexible representations of data.
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(19680801)
data = np.random.randn(2, 100)
fig, axs = plt.subplots(2, 2, figsize=(5, 5))
axs[0, 0].hist(data[0])
axs[1, 0].scatter(data[0], data[1])
axs[0, 1].plot(data[0], data[1])
axs[1, 1].hist2d(data[0], data[1])
plt.show()

ate tick labels¶
Show how to make date plots in matplotlib using date tick locators and formatters. See major_minor_demo1.py for more information on controlling major and minor ticks
All matplotlib date plotting is done by converting date instances into days since 0001-01-01 00:00:00 UTC plus one day (for historical reasons). The conversion, tick locating and formatting is done behind the scenes so this is most transparent to you. The dates module provides several converter functions matplotlib.dates.date2num
and matplotlib.dates.num2date
. These can convert between datetime.datetime
objects and numpy.datetime64
objects.

import datetime
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import matplotlib.cbook as cbook
years = mdates.YearLocator() # every year
months = mdates.MonthLocator() # every month
yearsFmt = mdates.DateFormatter('%Y')
# Load a numpy record array from yahoo csv data with fields date, open, close,
# volume, adj_close from the mpl-data/example directory. The record array
# stores the date as an np.datetime64 with a day unit ('D') in the date column.
with cbook.get_sample_data('goog.npz') as datafile:
r = np.load(datafile)['price_data'].view(np.recarray)
fig, ax = plt.subplots()
ax.plot(r.date, r.adj_close)
# format the ticks
ax.xaxis.set_major_locator(years)
ax.xaxis.set_major_formatter(yearsFmt)
ax.xaxis.set_minor_locator(months)
# round to nearest years...
datemin = np.datetime64(r.date[0], 'Y')
datemax = np.datetime64(r.date[-1], 'Y') + np.timedelta64(1, 'Y')
ax.set_xlim(datemin, datemax)
# format the coords message box
def price(x):
return '$%1.2f' % x
ax.format_xdata = mdates.DateFormatter('%Y-%m-%d')
ax.format_ydata = price
ax.grid(True)
# rotates and right aligns the x labels, and moves the bottom of the
# axes up to make room for them
fig.autofmt_xdate()
plt.show()
Log Demo¶
Examples of plots with logarithmic axes.

import numpy as np
import matplotlib.pyplot as plt
# Data for plotting
t = np.arange(0.01, 20.0, 0.01)
# Create figure
fig, ((ax1, ax2), (ax3, ax4)) = plt.subplots(2, 2)
# log y axis
ax1.semilogy(t, np.exp(-t / 5.0))
ax1.set(title='semilogy')
ax1.grid()
# log x axis
ax2.semilogx(t, np.sin(2 * np.pi * t))
ax2.set(title='semilogx')
ax2.grid()
# log x and y axis
ax3.loglog(t, 20 * np.exp(-t / 10.0), basex=2)
ax3.set(title='loglog base 2 on x')
ax3.grid()
# With errorbars: clip non-positive values
# Use new data for plotting
x = 10.0**np.linspace(0.0, 2.0, 20)
y = x**2.0
ax4.set_xscale("log", nonposx='clip')
ax4.set_yscale("log", nonposy='clip')
ax4.set(title='Errorbars go negative')
ax4.errorbar(x, y, xerr=0.1 * x, yerr=5.0 + 0.75 * y)
# ylim must be set after errorbar to allow errorbar to autoscale limits
ax4.set_ylim(ymin=0.1)
fig.tight_layout()
plt.show()
Legend using pre-defined labels¶
Defining legend labels with plots.

import numpy as np
import matplotlib.pyplot as plt
# Make some fake data.
a = b = np.arange(0, 3, .02)
c = np.exp(a)
d = c[::-1]
# Create plots with pre-defined labels.
fig, ax = plt.subplots()
ax.plot(a, c, 'k--', label='Model length')
ax.plot(a, d, 'k:', label='Data length')
ax.plot(a, c + d, 'k', label='Total message length')
legend = ax.legend(loc='upper center', shadow=True, fontsize='x-large')
# Put a nicer background color on the legend.
legend.get_frame().set_facecolor('#00FFCC')
plt.show()
Sample plots in Matplotlib¶
Here you’ll find a host of example plots with the code that generated them.
Multiple subplots in one figure¶
Multiple axes (i.e. subplots) are created with the subplot()
function:
Images¶
Matplotlib can display images (assuming equally spaced horizontal dimensions) using the imshow()
function.

Example of using imshow()
to display a CT scan
Contouring and pseudocolor¶
The pcolormesh()
function can make a colored representation of a two-dimensional array, even if the horizontal dimensions are unevenly spaced. The contour()
function is another way to represent the same data:

Example comparing pcolormesh()
and contour()
for plotting two-dimensional data
Histograms¶
The hist()
function automatically generates histograms and returns the bin counts or probabilities:
Three-dimensional plotting¶
The mplot3d toolkit (see Getting started and 3D plotting) has support for simple 3d graphs including surface, wireframe, scatter, and bar charts.
Thanks to John Porter, Jonathon Taylor, Reinier Heeres, and Ben Root for the mplot3d
toolkit. This toolkit is included with all standard Matplotlib installs.
Streamplot¶
The streamplot()
function plots the streamlines of a vector field. In addition to simply plotting the streamlines, it allows you to map the colors and/or line widths of streamlines to a separate parameter, such as the speed or local intensity of the vector field.
This feature complements the quiver()
function for plotting vector fields. Thanks to Tom Flannaghan and Tony Yu for adding the streamplot function.
Ellipses¶
In support of the Phoenix mission to Mars (which used Matplotlib to display ground tracking of spacecraft), Michael Droettboom built on work by Charlie Moad to provide an extremely accurate 8-spline approximation to elliptical arcs (see Arc
), which are insensitive to zoom level.
Bar charts¶
Use the bar()
function to make bar charts, which includes customizations such as error bars:
You can also create stacked bars (bar_stacked.py), or horizontal bar charts (barh.py).
Pie charts¶
The pie()
function allows you to create pie charts. Optional features include auto-labeling the percentage of area, exploding one or more wedges from the center of the pie, and a shadow effect. Take a close look at the attached code, which generates this figure in just a few lines of code.
Scatter plots¶
The scatter()
function makes a scatter plot with (optional) size and color arguments. This example plots changes in Google’s stock price, with marker sizes reflecting the trading volume and colors varying with time. Here, the alpha attribute is used to make semitransparent circle markers.
GUI widgets¶
Matplotlib has basic GUI widgets that are independent of the graphical user interface you are using, allowing you to write cross GUI figures and widgets. See matplotlib.widgets
and the widget examples.
Filled curves¶
The fill()
function lets you plot filled curves and polygons:
Thanks to Andrew Straw for adding this function.
Date handling¶
You can plot timeseries data with major and minor ticks and custom tick formatters for both.
See matplotlib.ticker
and matplotlib.dates
for details and usage.
Log plots¶
The semilogx()
, semilogy()
and loglog()
functions simplify the creation of logarithmic plots.
Thanks to Andrew Straw, Darren Dale and Gregory Lielens for contributions log-scaling infrastructure.
Legends¶
The legend()
function automatically generates figure legends, with MATLAB-compatible legend-placement functions.
Thanks to Charles Twardy for input on the legend function.
TeX-notation for text objects¶
Below is a sampling of the many TeX expressions now supported by Matplotlib’s internal mathtext engine. The mathtext module provides TeX style mathematical expressions using FreeType and the DejaVu, BaKoMa computer modern, or STIX fonts. See the matplotlib.mathtext
module for additional details.
Matplotlib’s mathtext infrastructure is an independent implementation and does not require TeX or any external packages installed on your computer. See the tutorial at Writing mathematical expressions.
Native TeX rendering¶
Although Matplotlib’s internal math rendering engine is quite powerful, sometimes you need TeX. Matplotlib supports external TeX rendering of strings with the usetex option.
EEG GUI¶
You can embed Matplotlib into pygtk, wx, Tk, or Qt applications. Here is a screenshot of an EEG viewer called pbrain.

The lower axes uses specgram()
to plot the spectrogram of one of the EEG channels.
For examples of how to embed Matplotlib in different toolkits, see:
Subplot example¶
Many plot types can be combined in one figure to create powerful and flexible representations of data.
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(19680801)
data = np.random.randn(2, 100)
fig, axs = plt.subplots(2, 2, figsize=(5, 5))
axs[0, 0].hist(data[0])
axs[1, 0].scatter(data[0], data[1])
axs[0, 1].plot(data[0], data[1])
axs[1, 1].hist2d(data[0], data[1])
plt.show()
