本文简单介绍一下spring boot+JPA实现简单的增删改查功能
一、项目结构简述
二、搭建spring boot+jap环境
三、代码示例
四、spring boot的启动方式
一、项目结构简述
1、项目的目录结构注意点
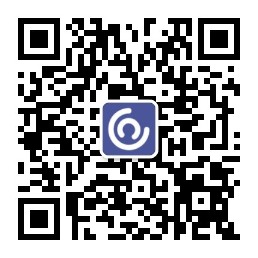
问题说明:
①使用内置服务器启动springboot
项目时,会从@SpringBootApplication
修饰类所在的包开始,加载当前包和所有子包下的类,将由@Component
@Repository
@Service
@Controller
修饰的类交由spring
进行管理;
②如上图,项目中的启动类为ApplacationController,他与其他层代码的处于同一个包下面,项目启动时会扫描到其他层的代码,就不会报如下错误(如果启动类所在的包与其它层代码所在的包处于平级目录中,即使启动类使用了@ComponentScan注解一样会报下面的错误):
Action: Consider defining a bean of type 'cn.oschina.dao.PersonRespority' in your configuration.
二、搭建spring boot+jap环境
1、pom.xml文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>cn.oschaina</groupId> <artifactId>simple-spring-boot</artifactId> <version>0.0.1-SNAPSHOT</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.9.RELEASE</version> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 添加Mysql和JPA--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.45</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.7.0</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> </project>
2、application.properites(或者是application.yml)
server.port=8081 server.context-path=/girl spring.datasource.url=jdbc:mysql://127.0.0.1:3306/test spring.datasource.username=root spring.datasource.password=root spring.datasource.driverClassName=com.mysql.jdbc.Driver # Specify the DBMS #spring.jpa.database=MYSQL # Hibernate ddl auto (create, create-drop, update) spring.jpa.hibernate.ddl-auto=update # Show or not log for each sql query spring.jpa.show-sql=true # Naming strategy #spring.jpa.hibernate.naming-strategy=org.hibernate.cfg.ImprovedNamingStrategy # stripped before adding them to the entity manager) spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
三、代码示例
1、代码示例
(1)启动类
package cn.oschina; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ConfigurableApplicationContext; /** * 应用启动类 自动扫描: * * 使用内置服务器启动springboot项目时,会从@SpringBootApplication修饰类所在的包开始,加载当前包和所有子包下的类, * * 将由@Component @Repository @Service @Controller修饰的类交由spring进行管理 * * @author Freedom * */ @SpringBootApplication public class ApplacationController { public static void main(String[] args) { ConfigurableApplicationContext context = SpringApplication.run( ApplacationController.class, args); String[] profiles = context.getEnvironment().getActiveProfiles(); if (profiles != null) { for (String profile : profiles) { System.out.println("------------start with profile : " + profile); } } } }
(2)控制层
package cn.oschina.controller; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import cn.oschina.entity.Person; import cn.oschina.service.IPersonService; @RestController public class PersonController { @Autowired private IPersonService personService; @GetMapping(value = "/persons") public List<Person> persons() { return personService.findAll(); } @GetMapping("/person/{id}") public Person getPersonById(@PathVariable("id") Integer id) { return personService.findOne(id); } @PostMapping("/save") public Person savaPerson(@RequestParam("name") String name, @RequestParam("age") Integer age) { Person p = new Person(); p.setAge(age); p.setName(name); return personService.insert(p); } @PutMapping("/update/{id}") public Person updatePerson(@PathVariable("id") Integer id, @RequestParam("name") String name) { Person p = new Person(); p.setId(id); p.setName(name); return personService.update(p); } @DeleteMapping("/delete/{id}") public void deletePerson(@PathVariable("id") Integer id) { personService.delete(id); } }
(3)业务层
package cn.oschina.service; import java.util.List; import cn.oschina.entity.Person; /** * 对表tbl_person的增删改查 * * @author Freedom * */ public interface IPersonService { List<Person> findAll(); Person findOne(Integer id); Person insert(Person p); Person update(Person p); void delete(Integer id); }
package cn.oschina.service; import java.util.List; import javax.annotation.Resource; import org.springframework.stereotype.Service; import cn.oschina.dao.PersonRespority; import cn.oschina.entity.Person; /** * 对表tbl_person的增删改查 * * @author Freedom * */ @Service public class PersonService implements IPersonService { @Resource private PersonRespority personRespority; @Override public List<Person> findAll() { return personRespority.findAll(); } @Override public Person findOne(Integer id) { return personRespority.findOne(id); } @Override public Person insert(Person p) { return personRespority.save(p); } /** * 更新也是使用save()方法 */ @Override public Person update(Person p) { return personRespority.save(p); } @Override public void delete(Integer id) { personRespority.delete(id); } }
(4)数据访问层
package cn.oschina.dao; import org.springframework.data.jpa.repository.JpaRepository; import cn.oschina.entity.Person; public interface PersonRespority extends JpaRepository<Person, Integer> { }
(5)实体
package cn.oschina.entity; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "tbl_person") public class Person { @Id @GeneratedValue private int id; private String name; private int age; public Person() { } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
2、运行分析
(1)POST请求时
(2)PUT方式请求说明
四、spring boot的启动方式
1、@SpringBootApplication注解的类,并且main函数中执行SpringApplication.run方法
public static void main(String[] args) { ConfigurableApplicationContext context = SpringApplication.run( ApplacationController.class, args); String[] profiles = context.getEnvironment().getActiveProfiles(); if (profiles != null) { for (String profile : profiles) { System.out.println("------------start with profile : " + profile); } } }
2、使用maven指令方式
①找到项目所在的根目录;
②执行mvn spring-boot:run指令;